How to select a div with a certain class using jQuery ?
Last Updated :
27 Oct, 2021
Given a HTML document containing many div element with classes. Here the task is to select a div with a certain class, that doesn’t have another class. There are two approaches to solve this problem. In one of them, we will use a method and in the other one, we will use not selector.
Approach 1: First, select the DIV with certain class using jQuery Selector and then use :not selector to ignore the elements of specific class.
- Example: This example implements the above approach.
html
<!DOCTYPE HTML>
< html >
< head >
< title >
Select a div with a certain class,
that doesn't have another class.
</ title >
< style >
body {
text-align: center;
}
h1 {
color: green;
}
.div {
background: green;
height: 50px;
width: 200px;
margin: 0 auto;
color: white;
border: 2px solid black;
}
</ style >
< script src =
</ script >
</ head >
< body >
< h1 >
GeeksforGeeks
</ h1 >
< p >
Click on button to select a div with a certain
class, that doesn't have another class.
</ p >
< div class = "first div" >
This is first Div
</ div >
< br >
< div class = "second div" >
This is second Div
</ div >
< br >
< div class = "third div" >
This is third Div
</ div >
< br >
< button onClick = "GFG_Fun()" >
click here
</ button >
< br >
< p id = "geeks" >
</ p >
< script >
/* main function */
function GFG_Fun() {
/* using the :not selector */
$('.div:not(.first)')
.css("background-color", "#173F5F");
$('#geeks')
.text("DIV Box of class 'first' is not selected.");
}
</ script >
</ body >
</ html >
|
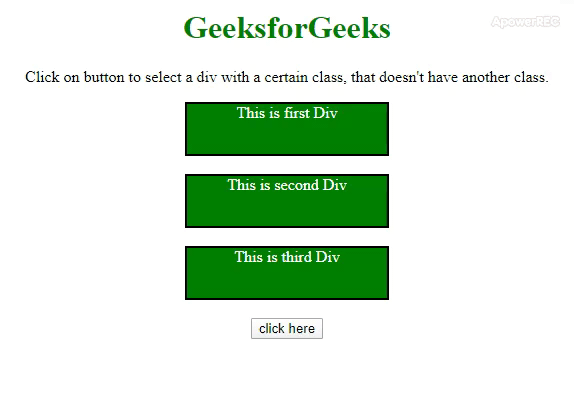
Approach 2: First, select the DIV with certain class using jQuery Selector and then Use .not() method to ignore the elements of specific class.
- Example: This example implements the above approach.
html
<!DOCTYPE HTML>
< html >
< head >
< title >
Select a div with a certain class,
that doesn't have another class.
</ title >
< style >
body {
text-align: center;
}
h1 {
color: green;
}
.div {
background: green;
height: 50px;
width: 200px;
margin: 0 auto;
color: white;
border: 2px solid black;
}
</ style >
< script src =
</ script >
</ head >
< body >
< h1 >
GeeksforGeeks
</ h1 >
< p >
Click on button to select a div with a certain
class, that doesn't have another class.
</ p >
< div class = "first div" >
This is first Div
</ div >
< br >
< div class = "second div" >
This is second Div
</ div >
< br >
< div class = "third div" >
This is third Div
</ div >
< br >
< button onClick = "GFG_Fun()" >
click here
</ button >
< br >
< p id = "geeks" >
</ p >
< script >
/* main function */
function GFG_Fun() {
/* using the .not() method */
$('.div').not('.first')
.css("background-color", "#173F5F");
$('#GFG_DOWN')
.text("DIV Box of class 'first' is not selected.");
}
</ script >
</ body >
</ html >
|
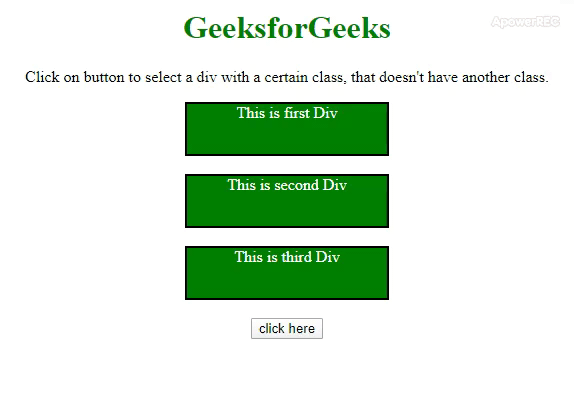
Share your thoughts in the comments
Please Login to comment...