How to render JSON in EJS using Express.js ?
Last Updated :
20 Mar, 2024
EJS (Embedded JavaScript) is a templating language that allows dynamic content generation in NodeJS applications. It allows us to embed JavaScript code directly into Our HTML code, and with the help of that we can generate the dynamic content on the server side. So with the help of ejs, we can perform SSR(Server-Side-Rendering).
In this tutorial, we’ll learn about the following approaches to render JSON in EJS and Express.
Approach 1: Direct Embedding
Steps to render the JSON data in the EJS template:
Step 1: Create a new server using the following command.
npm init -y
Step 2: Install the necessary package in your application using the following command.
npm i express ejs
Step 3: Define the JSON data in the carsData.json file.
{
"cars": [
{
"name": "Audi",
"model": "A4",
"price": 50000
},
{
"name": "BMW",
"model": "X5",
"price": 60000
},
{
"name": "Mercedes",
"model": "C-Class",
"price": 55000
},
{
"name": "Toyota",
"model": "Camry",
"price": 35000
}
]
}
Folder Structure:
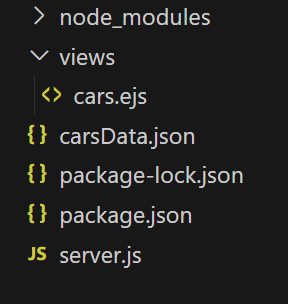
Folder structure
The updated dependencies in package.json file will look like:
"dependencies": {
"ejs": "^3.1.9",
"express": "^4.18.3"
}
Example: Write the following code in the files created as shown in folder structure
HTML
<%/* cars.ejs */%>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport"
content="width=device-width, initial-scale=1.0">
<title>Cars Info</title>
<style>
body {
font-family: Arial, sans-serif;
}
.container {
max-width: 600px;
margin: 0 auto;
padding: 20px;
}
select {
width: 100%;
padding: 10px;
font-size: 16px;
border: 1px solid #ccc;
border-radius: 5px;
margin-bottom: 10px;
cursor: pointer;
}
#carInfo {
background-color: #0dda29;
padding: 20px;
border-radius: 5px;
box-shadow: 0 2px 4px rgba(0, 0, 0, 0.1);
color: #fff;
}
</style>
</head>
<body>
<div class="container">
<h1>Here you can find many car's Information</h1>
<h2>Select a Car</h2>
<form>
<select id="carSelect" onchange="displayCarInfo()">
<option value="" disabled selected>--- Select a Car ---</option>
<% carsData.cars.forEach(function(car) { %>
<option value="<%= car.name %>">
<%= car.name %>
</option>
<% }); %>
</select>
</form>
<div id="carInfo">
<!-- Car information will be displayed here -->
</div>
</div>
<script>
var cars = JSON.parse('<%- JSON.stringify(carsData.cars) %>');
var carInfoDiv = document.getElementById('carInfo');
// Initialize the display once the page is loaded
window.onload = function() {
displayCarInfo();
};
function displayCarInfo() {
var carName = document.getElementById('carSelect').value;
carInfoDiv.style.display='none';
// Check if the selected value is empty or not
if (carName === '') {
// If no car is selected, clear the car info div
carInfoDiv.innerHTML = '';
return;
}
var selectedCar = cars.find(car => car.name === carName);
if(selectedCar){
carInfoDiv.style.display='block';
carInfoDiv.innerHTML = `
<h3>Selected Car Information</h3>
<p>Name: ${selectedCar.name}</p>
<p>Model: ${selectedCar.model}</p>
<p>Price: $${selectedCar.price}</p>
`;
}
}
</script>
</body>
</html>
Javascript
//server.js..........
const express = require('express');
const fs = require('fs');
const app = express();
const port = 3000;
// Set EJS as the view engine
app.set('view engine', 'ejs');
// Read JSON data from file
const carsData =
JSON.parse(fs.readFileSync('carsData.json', 'utf8'));
// Define a route to render the EJS template
app.get('/', (req, res) => {
// Render the 'user.ejs' template and pass the JSON data to it
res.render('cars', { carsData });
});
app.listen(port, (err) => {
if (err) {
console.log(err);
} else {
console.log(`Server is running
on http://localhost:${port}`);
}
});
To run the code wite i the following command in command prompt
node server.js
And open a new tab of your browser and type the following command to show the output
http://localhost:3000/
Output:
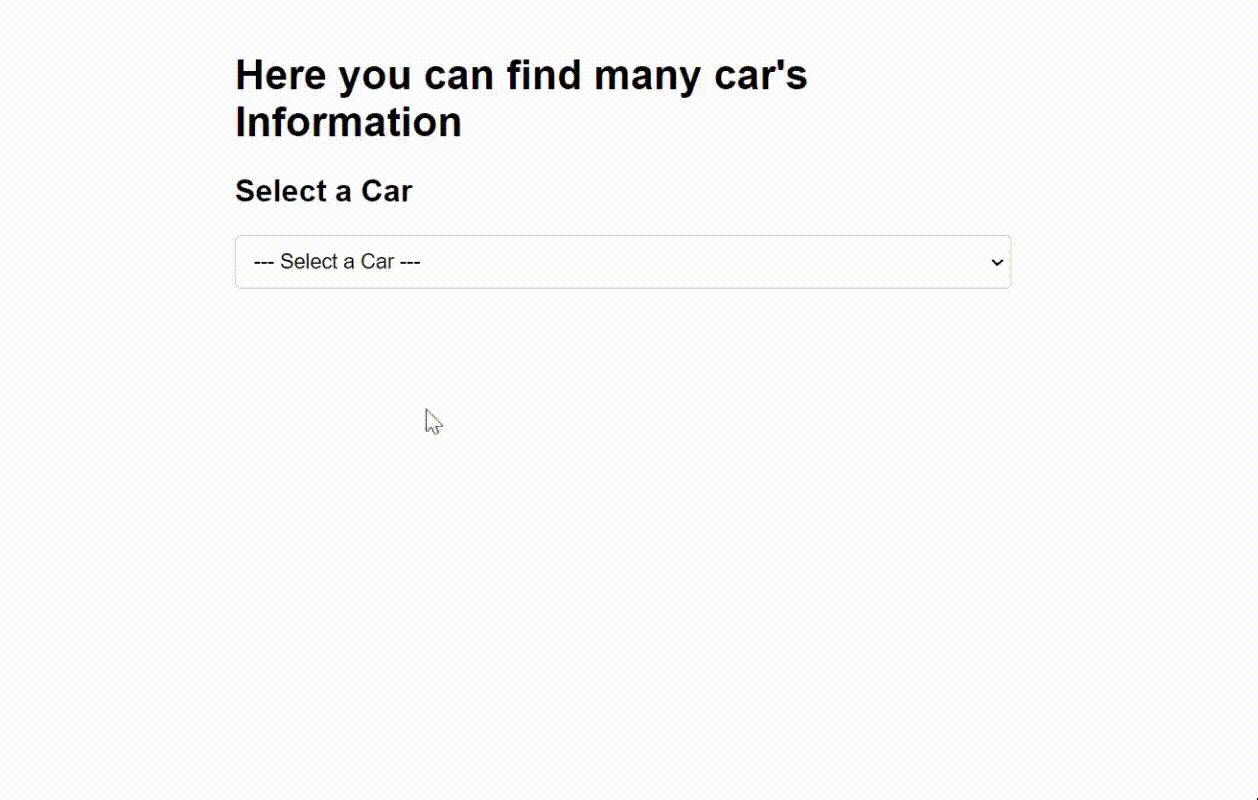
Output
Approach 2 : Using Middleware
- At first define a middleware function (fetchCarsData) to fetch the JSON data from a file.
- Then use fs.readFile to asynchronously read the JSON data from the file and use the “UTF-8” encoded string otherwise you got the Buffer object.
- And inside the middleware function, handle any errors that occur during the file reading process
Example:The example below illustrates the implementation torender json in EJS and express through above approach.
JavaScript
const express = require('express');
const fs = require('fs');
const app = express();
const port = 3000;
// Set EJS as the view engine
app.set('view engine', 'ejs');
// Define a middleware function to fetch the JSON data
const fetchCarsData = (req, res, next) => {
fs.readFile('carsData.json', 'utf8', (err, data) => {
if (err) {
return next(err); // Pass error to the error handling middleware
}
req.cars_Data = JSON.parse(data);
next(); // Move to the next middleware or route handler
});
};
// Use the middleware function for all routes
app.use(fetchCarsData);
// Define a route to render the EJS template
app.get('/', (req, res) => {
// Render the 'cars.ejs' template and pass the JSON data to it
res.render('cars', { carsData: req.cars_Data });
});
// Error handling middleware
app.use((err, req, res, next) => {
console.error(err.stack);
res.status(500).send('Something went wrong!');
});
app.listen(port, () => {
console.log(`Server is running on http://localhost:${port}`);
});
To run the code wite in the command prompt
node server.js
And open a new tab of your browser and type the following command to show the output
http://localhost:3000/
Output:
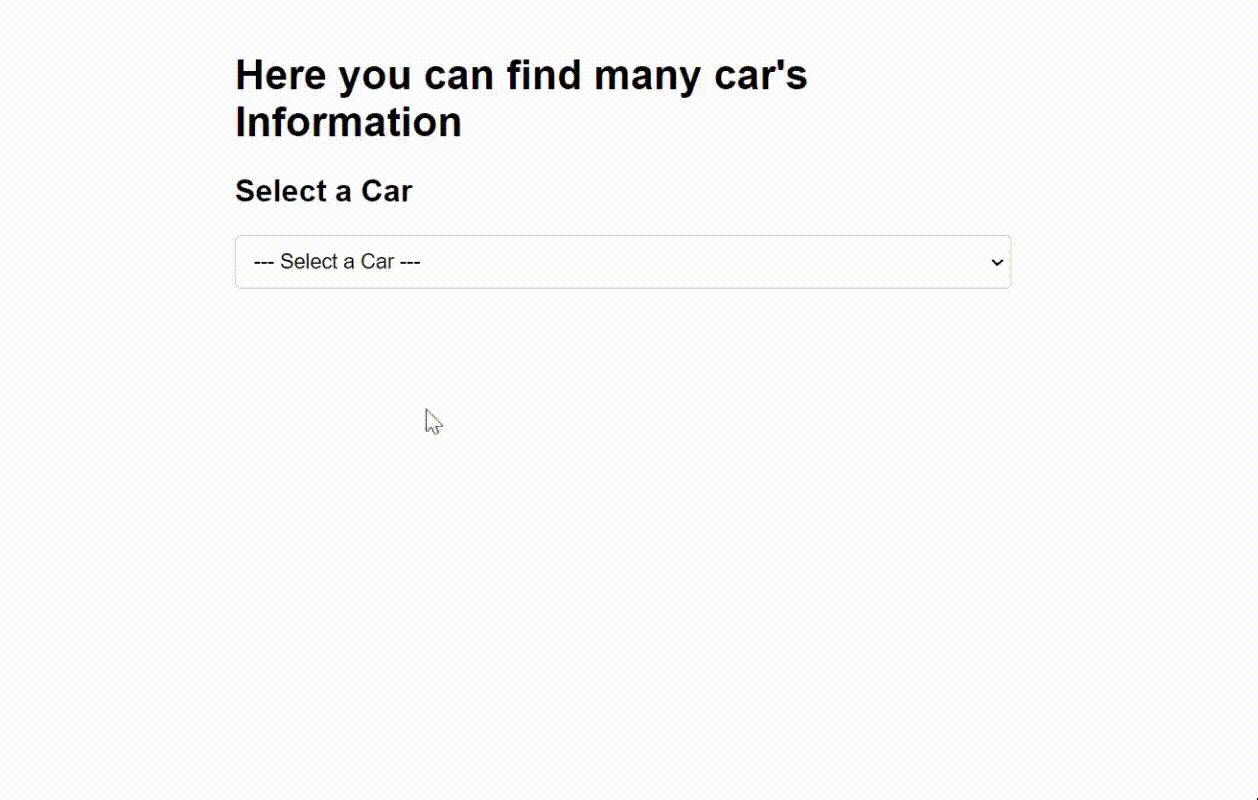
Output
Share your thoughts in the comments
Please Login to comment...