How to pass multiple parameter to @Directives in Angular ?
Last Updated :
26 Mar, 2024
Angular directives are TypeScript classes decorated with the @Directive
decorator. These are powerful tools for manipulating the DOM and adding behavior to elements. They can modify the behavior or appearance of DOM elements within an Angular application.
Directives can be broadly categorized into three types:
- Component Directives: These are directives with a template and can be used as standalone components.
- Attribute Directives: These change the appearance or behavior of an element, component, or another directive.
- Structural Directives: These change the DOM layout by adding or removing elements.
We will discuss the following approaches to pass multiple parameter to @directives in Angular:
Steps to Create Angular Project
Step 1: Create a new Angular project using the following command.
ng new gfg-directives
Folder Structure:
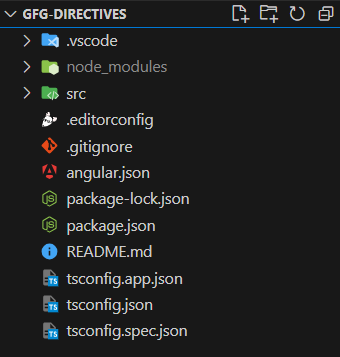
Project Structure of the new Angular application
Dependencies:
"dependencies": {
"@angular/animations": "^17.3.0",
"@angular/common": "^17.3.0",
"@angular/compiler": "^17.3.0",
"@angular/core": "^17.3.0",
"@angular/forms": "^17.3.0",
"@angular/platform-browser": "^17.3.0",
"@angular/platform-browser-dynamic": "^17.3.0",
"@angular/router": "^17.3.0",
"rxjs": "~7.8.0",
"tslib": "^2.3.0",
"zone.js": "~0.14.3"
},
"devDependencies": {
"@angular-devkit/build-angular": "^17.3.0",
"@angular/cli": "^17.3.0",
"@angular/compiler-cli": "^17.3.0",
"@types/jasmine": "~5.1.0",
"jasmine-core": "~5.1.0",
"karma": "~6.4.0",
"karma-chrome-launcher": "~3.2.0",
"karma-coverage": "~2.2.0",
"karma-jasmine": "~5.1.0",
"karma-jasmine-html-reporter": "~2.1.0",
"typescript": "~5.4.2"
}
Approach 1: Using Component Directives
Create a new component suing the following command.
ng generate component component-directive
Folder Structure:
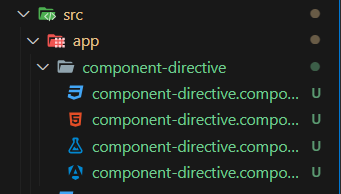
Component Structure
Code Example:
HTML
<!-- component-directive.component.html -->
<p>{{greetingMessage}}</p>
HTML
<!-- app.component.html -->
<app-component-directive
[firstName]="'Geeks'"
[lastName]="'ForGeeks'">
</app-component-directive>
JavaScript
//component-directive.component.ts
import { Component, Input } from '@angular/core';
@Component({
selector: 'app-component-directive',
templateUrl: './component-directive.component.html',
styleUrls: ['./component-directive.component.css']
})
export class ComponentDirectiveComponent {
@Input() firstName!: string;
@Input() lastName!: string;
greetingMessage = ""
ngOnInit() {
this.greetingMessage = `Welcome Back ${this.firstName}
${this.lastName}`
}
}
Output:
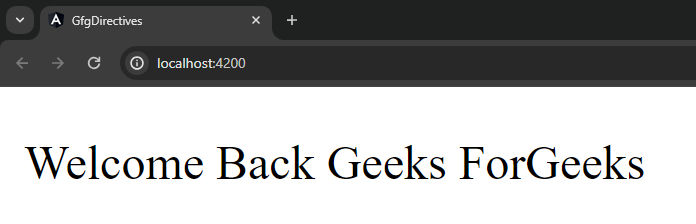
Browser
Approach 2: Using Attribute Directives
Create a new directive using the following command
ng generate directive attribute-directive
Folders Structure:
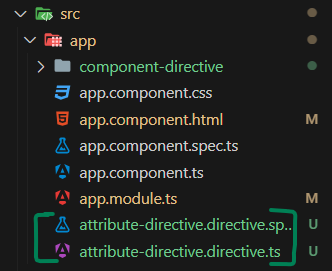
Attribute Directive Structure
Code Example:
HTML
<!-- app.component.html -->
<div appAttributeDirective [colorName]="'yellow'" [opacity]=0.4>
Hover over me to see the magic!
</div>
JavaScript
//attribute-directive.directive.ts
import { Directive, ElementRef, HostListener, Input } from '@angular/core';
@Directive({
selector: '[appAttributeDirective]'
})
export class AttributeDirectiveDirective {
constructor(private elementRef: ElementRef) { }
@Input() colorName!: string;
@Input() opacity!: number;
@HostListener('mouseenter') onMouseEnter() {
this.highlight(this.colorName, this.opacity);
}
@HostListener('mouseleave') onMouseLeave() {
this.highlight('', 1);
}
private highlight(color: string, opacity: number) {
this.elementRef.nativeElement.style.opacity = opacity;
this.elementRef.nativeElement.style.backgroundColor = color;
}
}
Output:
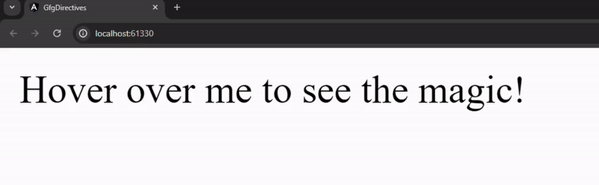
Browser
Approach 3: Using Structural Directives
Create a new directive using the following coammand.
ng generate directive structuredirective
Folder Structure:
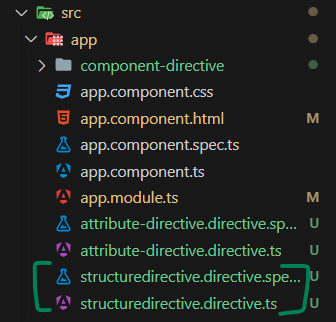
Structure Directive
Code Example:
HTML
<!-- app.component.html -->
<div *appStructuredirective="'GFGAllUsers';token:'AdminToken'"></div>
JavaScript
//structuredirective.directive.ts
import { Directive, Input } from '@angular/core';
@Directive({
selector: '[appStructuredirective]'
})
export class StructuredirectiveDirective {
@Input()
set appStructuredirective(value: string) {
console.log('appStructuredirective: ', value);
}
@Input()
set appStructuredirectiveToken(value: string) {
console.log('appStructuredirectiveToken: ', value);
}
}
Output:
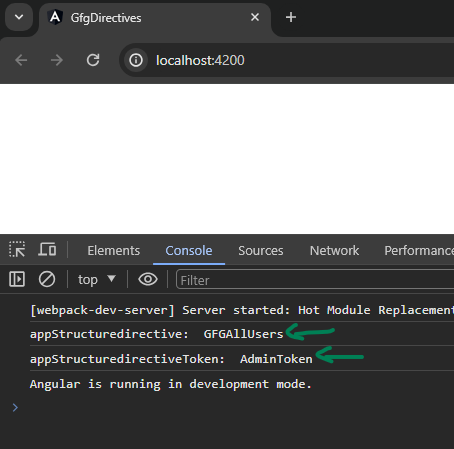
Browser’s Console
Share your thoughts in the comments
Please Login to comment...