Recursion in Angular Directives
Last Updated :
10 Jan, 2024
Recursion in Angular directives refers to the ability of a directive to include instances of itself within its template. This allows for the creation of nested structures and dynamic rendering based on the data. Recursive directives are useful when dealing with hierarchical data or tree-like structures.
Creating Angular application & module installation
Step 1: Create an Angular application using the following command.
ng new appname
Step 2: After creating your project folder i.e. appname, move to it using the following command.
cd appname
Step 3: Generate Directive using command
ng generate directive gfg
Project Structure
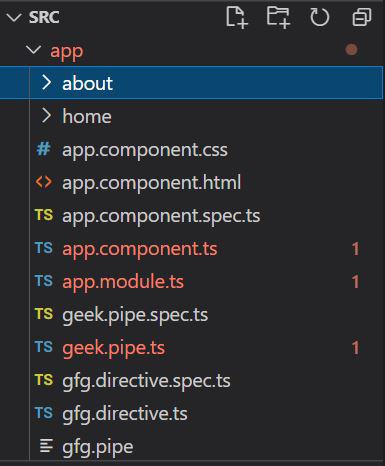
Example 1: Rendering a Simple Recursive List: We have created a list of objects in app.component.ts and we will recur over it in app.component.html. We have used the ngIf directive which checks the condition, whether the children exist or not. If children exist, then we have to recur to the children.
HTML
< h2 style = "color: green;" >GeeksforGeeks</ h2 >
< h2 >Recursion in Angular Directives</ h2 >
< ul >
< li * appGfg = "data" >
{{ data.name }}
< ul >
< ng-container * ngIf = "data.children" >
< li * ngFor = "let child of data.children" >
{{child.name}}
< ul >
< ng-container * ngIf = "child.children" >
< li * ngFor = "let innerChild of child.children" >
{{innerChild.name}}
</ li >
</ ng-container >
</ ul >
</ li >
</ ng-container >
</ ul >
</ li >
</ ul >
|
Javascript
import { Component } from '@angular/core' ;
import { BrowserModule, DomSanitizer }
from '@angular/platform-browser'
@Component({
selector: 'app-root' ,
templateUrl: './app.component.html' ,
styleUrls: [ './app.component.css' ]
})
export class AppComponent {
data = {
name: 'Courses' ,
children: [
{
name: 'FrontEnd' ,
children: [
{ name: 'HTML' , children: [] },
{ name: 'CSS' , children: [] },
{ name: 'Javascript' , children: [] }
]
},
{
name: 'Backend' ,
children: [
{ name: 'Java' , children: [] },
{ name: 'Node' , children: [] },
{ name: 'Python' , children: [] }
]
}
]
};
data2: any = ""
}
|
Javascript
import { NgModule }
from '@angular/core' ;
import { AppComponent }
from './app.component' ;
import { BrowserModule }
from '@angular/platform-browser' ;
import { GfgDirective }
from './gfg.directive' ;
@NgModule({
bootstrap: [
AppComponent
],
declarations: [
AppComponent, GfgDirective
],
imports: [
BrowserModule
]
})
export class AppModule { }
|
Javascript
import { Directive, Input, TemplateRef, ViewContainerRef }
from '@angular/core' ;
@Directive({
selector: '[appGfg]'
})
export class GfgDirective {
@Input() appGfg: any;
constructor(
private templateRef: TemplateRef<any>,
private viewContainerRef: ViewContainerRef
) { }
ngOnChanges() {
this .viewContainerRef.clear();
this .viewContainerRef.createEmbeddedView( this .templateRef, {
$implicit: this .appGfg
});
}
}
|
Output:
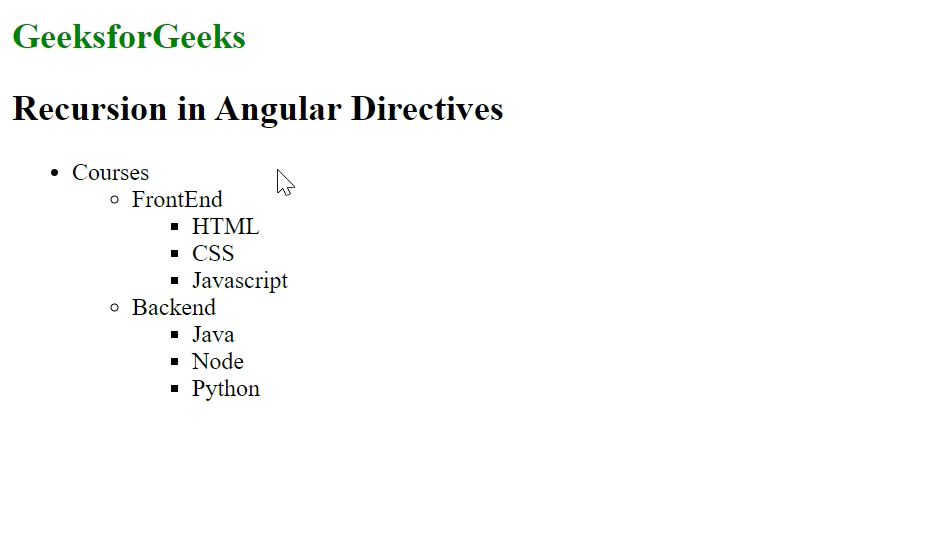
Example 2: In this example, we will do a recursion as soon as we find any of the children. Each node will be treated as unique and the document will be displayed for each node separately. Via recursion, it will reach each child. Each node can have child nodes, and the same component can render them recursively.
HTML
< h2 style = "color: green;" >GeeksforGeeks</ h2 >
< h2 >Recursion in Angular Directives</ h2 >
< div * appGfg = "data" >
{{ data.name }}
< div * ngIf = "data.children" >
< app-root * ngFor = "let child of data.children"
[data]="child">
</ app-root >
</ div >
</ div >
|
Javascript
import { Component, Input } from '@angular/core' ;
import { BrowserModule, DomSanitizer }
from '@angular/platform-browser'
@Component({
selector: 'app-root' ,
templateUrl: './app.component.html' ,
styleUrls: [ './app.component.css' ]
})
export class AppComponent {
@Input() data = {
name: 'Courses' ,
children: [
{
name: 'FrontEnd' ,
children: [
{ name: 'HTML' , children: [] },
{ name: 'CSS' , children: [] },
{ name: 'Javascript' , children: [] }
]
},
{
name: 'Backend' ,
children: [
{ name: 'Java' , children: [] },
{ name: 'Node' , children: [] },
{ name: 'Python' , children: [] }
]
}
]
};
data2: any = ""
@Input() node: any
}
|
Javascript
import { NgModule }
from '@angular/core' ;
import { AppComponent }
from './app.component' ;
import { BrowserModule }
from '@angular/platform-browser' ;
import { GfgDirective }
from './gfg.directive' ;
@NgModule({
bootstrap: [
AppComponent
],
declarations: [
AppComponent, GfgDirective
],
imports: [
BrowserModule
]
})
export class AppModule { }
|
Javascript
import { Directive, Input, TemplateRef, ViewContainerRef }
from '@angular/core' ;
@Directive({
selector: '[appGfg]'
})
export class GfgDirective {
@Input() appGfg: any;
constructor(
private templateRef: TemplateRef<any>,
private viewContainerRef: ViewContainerRef
) { }
ngOnChanges() {
this .viewContainerRef.clear();
this .viewContainerRef.createEmbeddedView( this .templateRef, {
$implicit: this .appGfg
});
}
}
|
Output
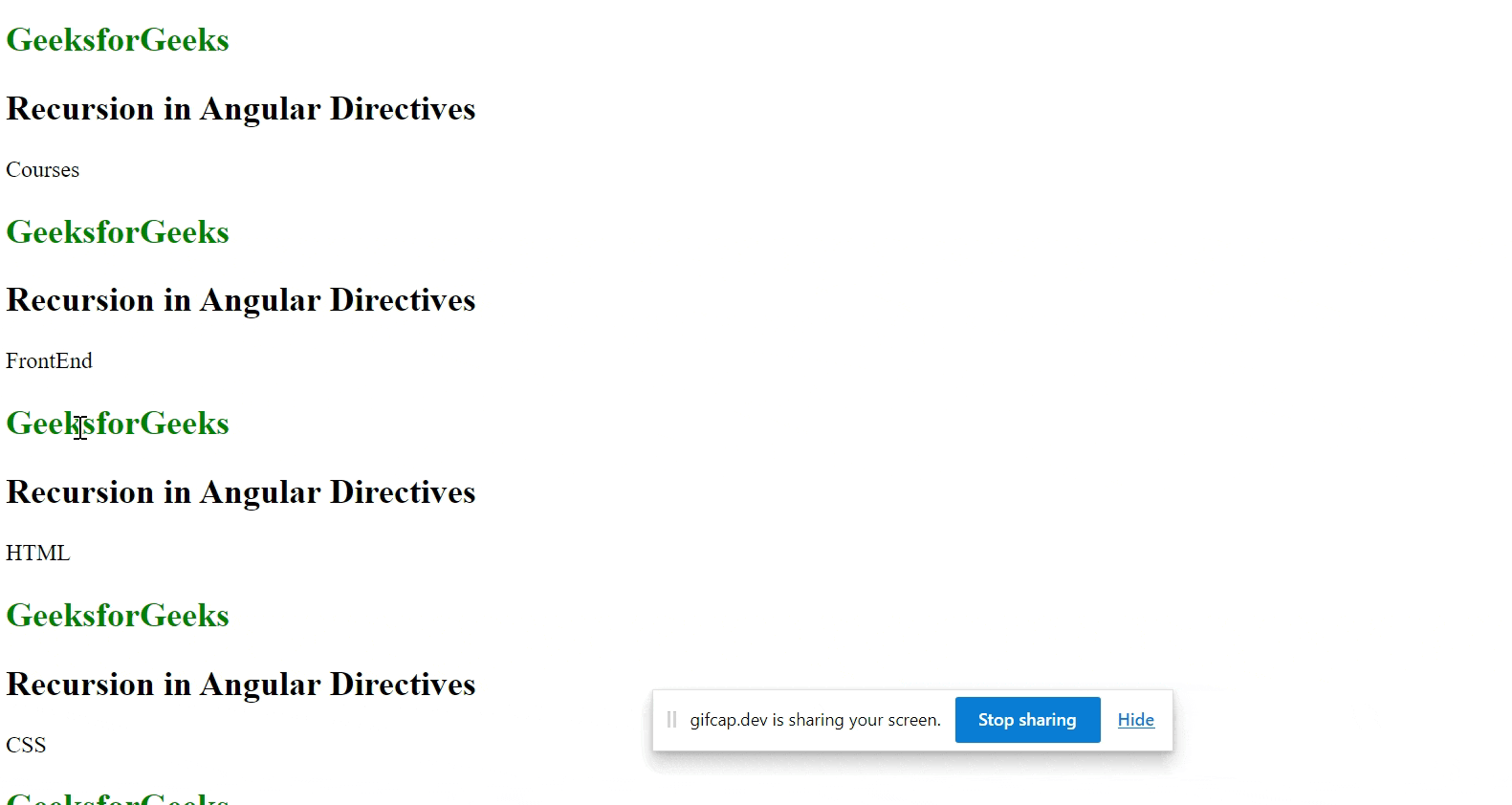
Conclusion
Recursion is a powerful concept in computer science that involves a function calling itself to solve a problem. In the context of Angular, a popular JavaScript framework for building web applications, recursion can be a valuable tool for creating dynamic and reusable components.
Share your thoughts in the comments
Please Login to comment...