How to Migrate a Microservice from MySQL to MongoDB
Last Updated :
26 Mar, 2024
Migrating from MySQL to MongoDB is a strategic decision that can unlock new possibilities for your database infrastructure. MongoDB’s document-based approach offers flexibility and scalability, enabling us to store and manage data more efficiently.
Before doing this migration journey, we must careful planning and consideration of key factors are essential. Understanding the differences between MySQL and MongoDB in terms of data format, query language, schema, and scalability is crucial for a successful migration.
Prerequisites
- The ability to code in JavaScript language.
- Installed MySQL on your system.
- Have an account on the MongoDB server
What is MongoDB?
- MongoDB stands out to be a frequently open-source powerful NoSQL document-based database product.
- In contrast to storing the information in tables, like in the conventional relational database, MongoDB contains such documents in the BSON format that are flexible and have dynamic structures known as JSON-like schema.
- This is a benefit that enables big data applications to store large volumes of data in a flexible and scalable way that traditional SQL databases may not be able to achieve.
- It supports a powerful query language that includes a wide range of query operators and aggregation pipelines for data manipulation and analysis.
Why Choose MongoDB Over SQL
Aspect
|
MySQL
|
MongoDB
|
Data Format
|
Tabular (Rows and Columns)
|
Document-based (JSON-like Documents)
|
Query Language
|
Structured Query Language (SQL)
|
JavaScript-like Queries
|
Schema
|
Fixed Schema (Structured)
|
Flexible Schema (Unstructured/Semi Structured)
|
Relationships
|
Relationships defined using Foreign Keys
|
Relationships embedded or referenced
|
Scalability
|
Vertical Scaling (Adding more resources)
|
Horizontal Scaling (Sharding)
|
Transactions
|
ACID Transactions
|
Multi-document Transactions (within a shard)
|
Complexity
|
Well-suited for complex queries
|
Better performance for simple queries
|
Performance
|
Performance can be impacted by complexity
|
High performance for simple queries
|
Hence, MongoDB overcomes the common challenges faced in terms of reliability, flexibility, scalling and performance over MySQL.
Key Considerations for Migrating to Microservices with MongoDB
Before Microservice Migration, consider team expertise and few other important things as follows:
1. Planning the Migration
- Schema Mapping: Decide about migration of your MySQL tables into MongoDB and design the structure of our collections, that is the way the data will be stored.
- Downtime Strategy: Ensure that there are no service interruptions, and the process can be carried out in a single implementation, or it could be done in stages if need be.
2. Data Migration
- Data Extraction: Transition to the next phase of our migration plan by utilizing mysqldump or scripting our own bit of code to transfer your MySQL data.
- Data Transformation: MongoDB and MySQL stores data differently in the sense that MongoDB relies on documents and a related structure, but MySQL does not. As a result, we may need to adapt during the process of migration. While the Load command is less functional, Mongo import and custom scripts, being more versatile from its foundations, can be efficient in this instance.
3. Code Adjustments
- Database Library Switch: We should also use the driver of MongoDB in our language and should not use the MySQL drivers.
- Query Language Shift: Since MongoDB comes with a advanced search interface through which we can perform CRUD operations, (Create, Read, Update, Delete) on documents. Its query is based on JavaScript using MongoDB Querying API.
4. Testing and Validation
- Unit Tests: Implementation of test ones that will make it possible to confirm a proper MongoDB technique should be done by our microservice.
- Integration Tests: Once the migration is done and the micro-service talks to the other services, have it tested.
- Performance Testing: Verify if our microservice performs well after its upward migration, by making sure that it operates as initially planned.
5. Deployment Restrictions
- Staged Rollout: This will be done in phased manner starting from a few restricted group of users, which will enable the detection and solving of bugs.
- Monitor and Refine: Implement monitoring of the microservices performance and user experience after the release. Prepare an open mind for those trivial changes that may be required at any time.
As these stages are the minor one of the migrations from MySQL to MongoDB (Document-oriented databases), the full procedure can be successfully accomplished!
Types of Migration
- Full Migration: There is a need to relocate all the MySQL data to MongoDB.
- Partial Migration: It consists in transferring either some certain tables or data from MySQL to MongoDB.
How to Migrate Microservice from MySQL Data to MongoDB Server
Follow these steps to migrate a microservice from MySQL to MongoDB:
- Analyze your SQL schema:First review the structure of SQL database objects, which comprise of entity tables including primary keys and indexes. This process is meant to give we an insight into the source and target data, its quality, and hidden migration-related aspects.
- Design your MongoDB schema: Create a MongoDB schema on your SQL (domain name) based on SQL schema analysis. One phase is represented by this where collections and documents are created, entities are defined, and indexing takes place. Bearing in mind MongoDB’s document- oried nature and denormalization, make sure we choose the dimensional and normalization techniques that work for your database design.
- Export SQL data: we should export data from the SQL database that you prefer to use via your chosen export tool. The most typical guesses are CSV, JSON, or XML. As for data and schema exports, be sure to create them before migration so we can use them as referencing material during the transfer.
- Transform SQL data: Compose scripts or use Apache Nifi to transform the SQL data that has been exported into a format that can go into the required MongoDB format. This mechanism could be the overhaul of the data, the update of field names, and the changing of data types which allow the data to have the same MongoDB schema.
- Import transformed data into MongoDB: Either use the mongoimport command or a tool like MongoDB Compass for importing the changed data into the MongoDB database. Fasten up please and make sure that we bring the data in the required collections and documents regarding how you arranged the MongoDB schema plan.
- Validate and test your MongoDB database: Make sure that our Mongo structure is the same as the data structure of relational database. Carry out hit and run of data magnificence, perform query tests and deal with performance tests to make sure our new database is running properly.
- Update application code: Alter our program code so that the MongoDB repository replaces the existing database. These particular things could be the process of rewriting SQL queries as MongoDB queries, altering the pattern of data access, and selection of correct MongoDB driver.
- Test application functionality: Performing a completely application debugging to ensure that it works as anticipated with the new MongoDB Database.
- Partial Migration: Always ensure to migrate our data partially to avoid downtime as well as fix the existing problems if any.
- Data Viewing: You can consistently monitor or view our data in MongoDB database using tools like MongoDB Atlas and MongoDB Compass.
Code Scripting Approach
Here’s an example of how we might migrate data from a MySQL table to a MongoDB collection using Node.js:
const mysql = require('mysql');
const MongoClient = require('mongodb').MongoClient;
// MySQL connection
const mysqlConnection = mysql.createConnection({
host: 'localhost',
user: 'mysql_user',
password: 'mysql_password',
database: 'mysql_database'
});
// MongoDB connection
MongoClient.connect('mongodb://localhost:27017', (err, client) => {
if (err) throw err;
const db = client.db('your_mongodb_database');
const collection = db.collection('mongodb_collection');
// Query MySQL table
mysqlConnection.query('SELECT * FROM your_mysql_table', (err, results) => {
if (err) throw err;
// Insert data into MongoDB
collection.insertMany(results, (err, result) => {
if (err) throw err;
console.log('Data migrated successfully');
client.close();
});
});
});
Congratulations! for successful Migration of microservice from MySQL to MongoDB.
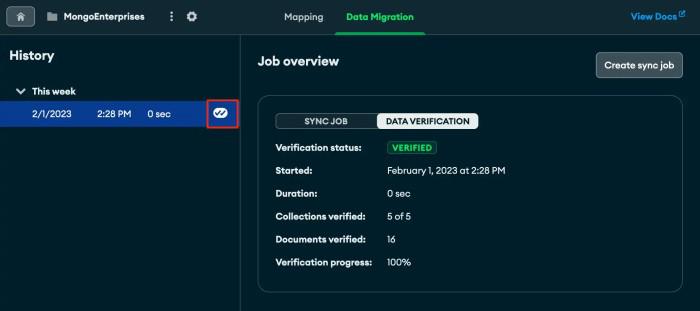
Data Migrated Successfully
Conclusion
Overall, migrating a microservice from MySQL to MongoDB is a complex process that requires careful planning, execution, and testing. By following the steps outlined in this guide, including schema analysis, data transformation, and code adjustments, you can successfully transition to MongoDB and take advantage of its flexible document-based architecture. It’s important to validate and test your MongoDB database thoroughly to ensure that it meets your performance and functionality requirements. Additionally, consider partial migration to minimize downtime and address any existing issues in your application code. With proper preparation and execution, you can achieve a successful migration to MongoDB and leverage its capabilities for your microservice
Share your thoughts in the comments
Please Login to comment...