How to Implement Reveal on Scroll in React using Tailwind CSS ?
Last Updated :
30 Nov, 2023
In React, the reveal on the scroll is a technique, where elements on a page gradually appear or animate as the user scrolls down.
Prerequisites:
To implement reveal in scroll in react using Tailwind CSS we have these methods:
Step for Creating React Application and Installing Module:
Step 1: Create a React application using the following command:
npm create-react-app appname
Step 2: After creating your project folder i.e. folder name, move to it using the following command:
cd foldername
Step 3: After creating the React.js application, install the Tailwind CSS using the following command.
npm i -D tailwindcss postcss autoprefixer
npx tailwindcss init -p
Step 4: Add Tailwind CSS directives into index.css file of the project.
@tailwind base;
@tailwind components;
@tailwind utilities;
Step 5: Configure template paths in tailwind.config.js file using the following command:
module.exports = {
content: [
"./src/**/*.{js,jsx,ts,tsx}",
],
}
Project Structure:
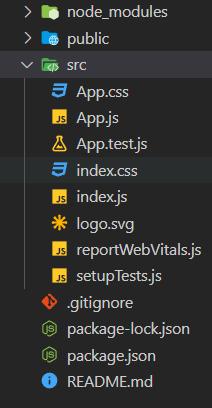
The updated dependencies in package.json file will look like.
"devDependencies": {
"autoprefixer": "^10.4.16",
"postcss": "^8.4.31",
"tailwindcss": "^3.3.5"
}
Approach 1: Using the Intersection Observer API
The Intersection Observer API is used to implement the reveal on scroll effect in React using Tailwind CSS. It allows you to asynchronously observe changes in the intersection of a target element with its container or the viewport.
Example: This example creates RevealOnScroll components using the tailwind classes and scroll observer.
Javascript
import React, { useEffect, useRef, useState } from "react" ;
const RevealOnScroll = ({ children }) => {
const [isVisible, setIsVisible] = useState( false );
const ref = useRef( null );
useEffect(() => {
const scrollObserver = new IntersectionObserver(([entry]) => {
if (entry.isIntersecting) {
setIsVisible( true );
scrollObserver.unobserve(entry.target);
}
});
scrollObserver.observe(ref.current);
return () => {
if (ref.current) {
scrollObserver.unobserve(ref.current);
}
};
}, []);
const classes = `transition-opacity duration-1000
${isVisible ? "opacity-100" : "opacity-0"
}`;
return (
<div ref={ref} className={classes}>
{children}
</div>
);
};
const App = () => {
return (
<div className= "container mx-auto text-center" >
<h1 className= "text-7xl font-bold mb-8 text-green" >
GeeksforGeeks
</h1>
<h2 className= "text-4xl " >
Reveal on Scroll in React using Tailwind CSS
</h2>
<RevealOnScroll>
<p className= "text-3xl h-[15em] mt-[10em]" >
Welcome to the computer science portal!
</p>
</RevealOnScroll>
<RevealOnScroll>
<p className= "text-3xl h-[15em] mt-[10em]" >
Learn Web Development
</p>
</RevealOnScroll>
<RevealOnScroll>
<p className= "text-3xl h-[15em] mt-[10em]" >
Learn Android Development
</p>
</RevealOnScroll>
<RevealOnScroll>
<p className= "text-3xl h-[15em] mt-[10em]" >
Learn Data Stuctures and Algorithms
</p>
</RevealOnScroll>
<RevealOnScroll>
<p className= "text-3xl h-[15em] mt-[10em]" >
and more...
</p>
</RevealOnScroll>
{
}
</div>
);
};
export default App;
|
Steps to Run the Application: Use this command in the terminal in the project directory.
npm start
Output: This output will be visible on the http://localhost:3000 on the browser window
.gif)
The Scroll Event Listener is used to implement the reveal on scroll effect in React using Tailwind CSS. It involves attaching an event listener to the scroll event of the window object or any scrollable element. When the user scrolls, the event listener fires and triggers the associated callback function.
Example: This example implements revel on scoll using scroll event listener to trigger and reveal content with cusotm scroll values.
Javascript
import React, { useEffect, useRef, useState } from "react" ;
const RevealOnScroll = ({ children }) => {
const [isVisible, setIsVisible] = useState( false );
const ref = useRef( null );
useEffect(() => {
const onWindScroll = () => {
const element = ref.current;
if (element) {
const { top } = element.getBoundingClientRect();
const isVisible = top < window.innerHeight;
setIsVisible(isVisible);
}
};
window.addEventListener( "scroll" , onWindScroll);
return () => {
window.removeEventListener( "scroll" , onWindScroll);
};
}, []);
const classes = `transition-opacity duration-1000
${isVisible ? "opacity-100" : "opacity-0"
}`;
return (
<div ref={ref} className={classes}>
{children}
</div>
);
};
const App = () => {
return (
<div className= "container mx-auto text-center" >
<h1 className= "text-7xl font-bold mb-8 text-green" >
GeeksforGeeks
</h1>
<h2 className= "text-4xl " >
Reveal on Scroll in React using Tailwind CSS
</h2>
<RevealOnScroll>
<p className= "text-3xl h-[15em] mt-[10em]" >
Welcome to the computer science portal!
</p>
</RevealOnScroll>
<RevealOnScroll>
<p className= "text-3xl h-[15em] mt-[10em]" >
Learn Web Development
</p>
</RevealOnScroll>
<RevealOnScroll>
<p className= "text-3xl h-[15em] mt-[10em]" >
Learn Android Development
</p>
</RevealOnScroll>
<RevealOnScroll>
<p className= "text-3xl h-[15em] mt-[10em]" >
Learn Data Stuctures and Algorithms
</p>
</RevealOnScroll>
<RevealOnScroll>
<p className= "text-3xl h-[15em] mt-[10em]" >
and more...
</p>
</RevealOnScroll>
</div>
);
};
export default App;
|
Steps to Run the Application: Use this command in the terminal in the project directory.
npm start
Output: This output will be visible on the http://localhost:3000 on the browser window
.gif)
Share your thoughts in the comments
Please Login to comment...