How to properly handle SIGINT with Express.js?
Last Updated :
25 Dec, 2023
Handling SIGINT in Express JS is mainly the process of shutting down the server. This SIGINT is mainly processed when we press the Ctrl + C in the terminal. For the proper shutting down of the server, we can handle this SIGINT using different approaches so that the proper cleanup of the task will be done and the server will get shut down properly.
In this article, we are going to properly handle SIGINT in Express with the given approaches.
Prerequisites
Steps to create Express application:
Step 1: In the first step, we will create the new folder by using the below command in the terminal.
mkdir folder-name
cd folder-name
Step 2: After creating the folder, initialize the NPM using the below command. Using this the package.json file will be created.
npm init-y
Step 3: Now, we will install all the dependencies for our project using the below command.
npm install express
Project Structure:
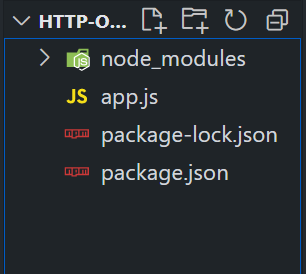
The updated dependencies in package.json file will look like:
"dependencies": {
"express": "^4.18.2"
}
Approach 1: Basic Signal Handling
- We are performing the basic signal handling in this approach, where the application will mainly listen for the SIGINT signal when the Ctrl+C is been triggered in the terminal.
- When the signal is received the Express server will get shut down properly by allowing the existing connections to finish the processing before exiting the process.
Example: Implementation of the above-discussed approach.
Javascript
const express = require( 'express' );
const app = express();
const port = 3000;
app.get( '/' , (req, res) => {
res.send( 'Hello, World!' );
});
const server = app.listen(port, () => {
console.log(`Server is running on port ${port}`);
});
process.on( 'SIGINT' , () => {
console.log( 'Received SIGINT. Closing server...' );
server.close(() => {
console.log( 'Server closed. Exiting process...' );
process.exit(0);
});
});
|
Output:
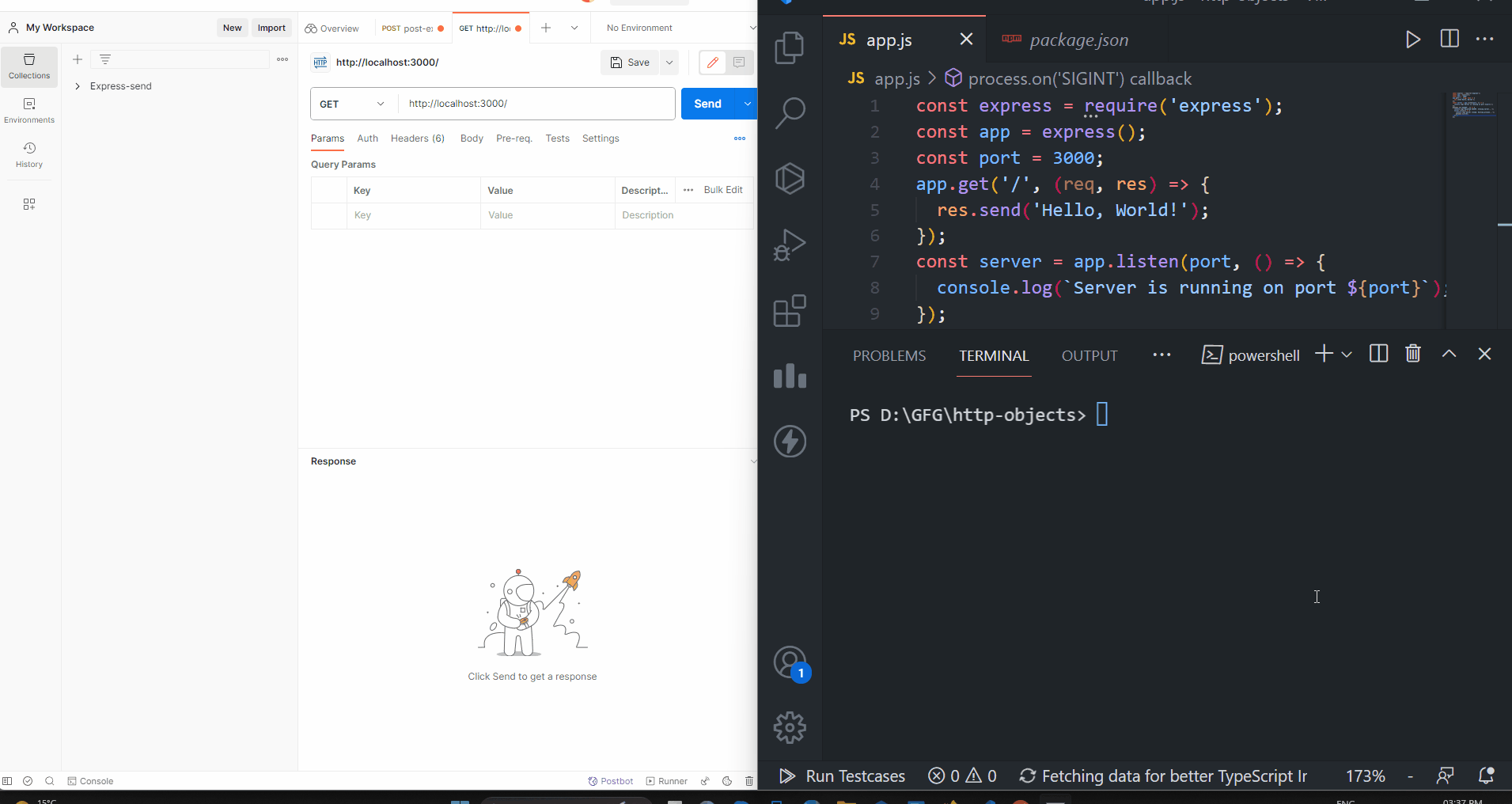
Approach 2: Using ‘once’ to Handle SIGINT
- We are using the process.once function to register a one-time event handler for SIGINT. This mainly makes sure that the server is closed properly.
- In the above approach, the callback is executed every time a SIGINT signal is received whereas, in this approach, the handler is executed only the first time as the SIGINT signal is received which prevents the subsequent executions and reduces the redundancy.
Example: Implementation of the above-discussed approach.
Javascript
const express = require( 'express' );
const app = express();
const port = 3000;
app.get( '/' , (req, res) => {
res.send( 'Hello, GFG!' );
});
const server = app.listen(port, () => {
console.log(`Server is running on port ${port}`);
});
let shuttingDown = false ;
process.once( 'SIGINT' , () => {
if (!shuttingDown) {
shuttingDown = true ;
console.log( 'Received SIGINT. Closing server...' );
server.close(() => {
console.log( 'Server closed. Exiting process...' );
process.exit(0);
});
}
});
|
Output:
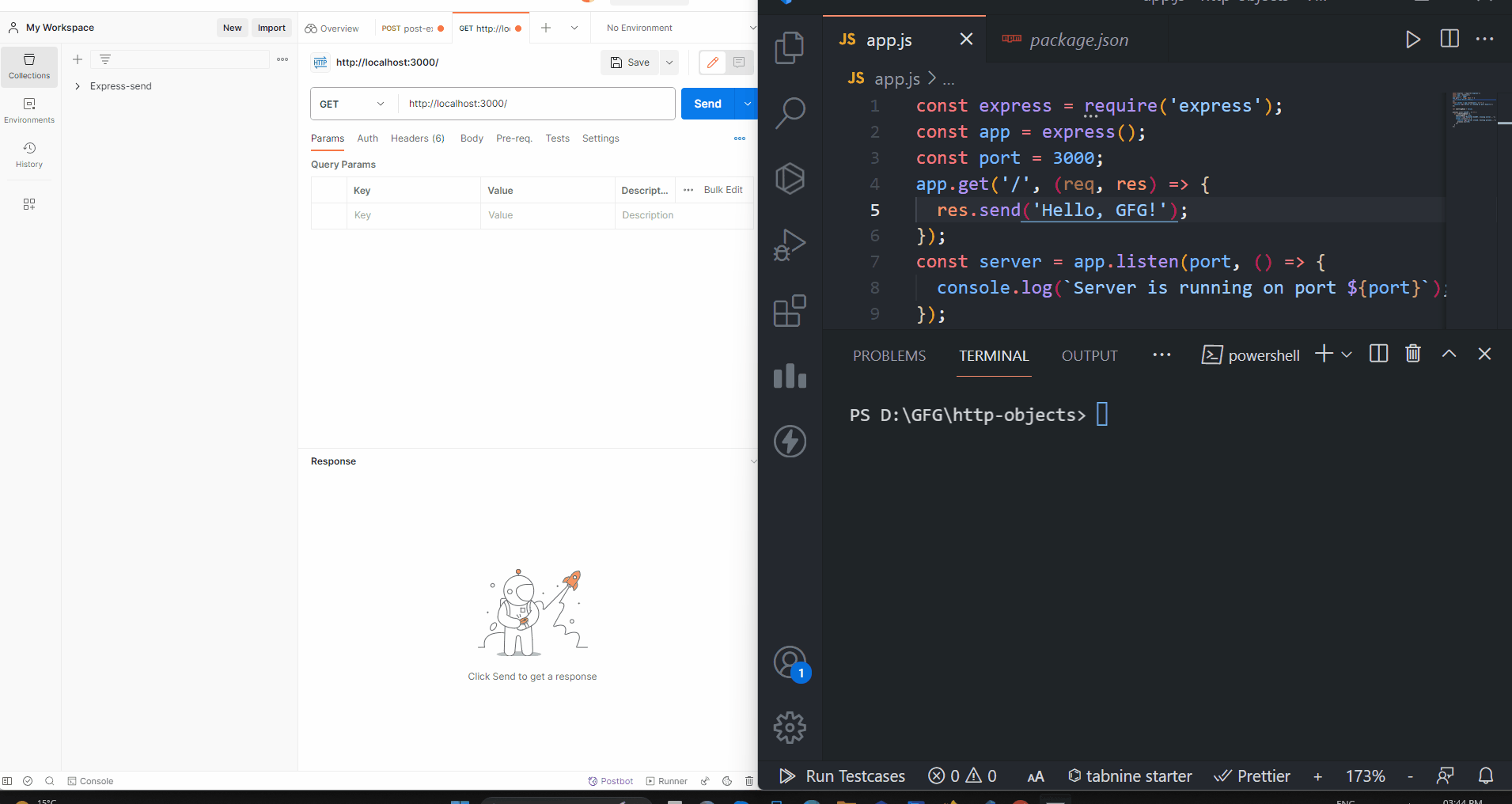
Share your thoughts in the comments
Please Login to comment...