How to handle redirects in Express JS?
Last Updated :
14 Feb, 2024
Express JS uses redirects to send users from one URL to another. This can be done for various reasons, such as handling outdated URLs or guiding users through a process. In ExpressJS, you define routes and use them to direct users to the desired URL. It’s a way to keep your application organized and user-friendly.
Steps to Handle Redirects in Express JS:
Step 1: Import the express: Install the express package in your app using the following command.
npm install express
Step 2: Create an express application: To create an express application by calling express() function i.e.
const app = express()
Step 3: Define the routes: Use ExpressJS to define routes in your application using methods like app.get()
or app.post()
.
app.get('/old-page', (req, res) => {
res.redirect('/new-page');
});
app.post('/submit-form', (req, res) => {
// Perform some processing...
res.redirect('/thank-you');
});
Handling redirects in ExpressJS involves creating an Express application, defining routes that specify URL paths and corresponding route handlers, and using the res.redirect()
method within route handlers to redirect users to different URLs. Finally, we start the server to listen for incoming requests on a specified port.
Example: Below is the example to handle redirects in ExpressJS.
Javascript
const express = require( 'express' );
const app = express();
app.get( '/old-url' , (req, res) => {
res.redirect( '/new-url' );
});
app.post( '/submit-form' , (req, res) => {
res.redirect( '/thankyou' );
});
app.get( '/new-url' , (req, res) => {
res.send( 'This is the new URL' );
});
app.get( '/thankyou' , (req, res) => {
res.send( 'Thank you for submitting the form!' );
});
const port = 3000;
app.listen(port,
() => {
console.log(`Server is running on http:
});
|
Start the server using the following command.
node server.js
Output:
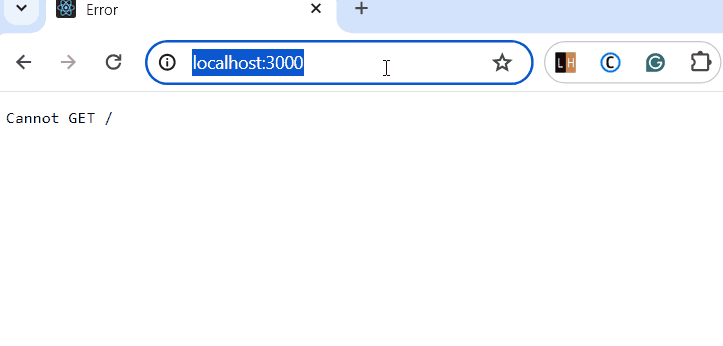
Output
Share your thoughts in the comments
Please Login to comment...