How to get YouTube channel id using Node.js ?
Last Updated :
29 Mar, 2022
The following approach covers how to get the YouTube channel Id using NodeJS. We will use the @gonetone/get-youtube-id-by-url node-package to achieve so. This package will help us for getting the YouTube channel id by using the channel URL.
Use the following steps to install the module and get the YouTube channel Id in node.js:
Step 1: Creating a directory for our project and making that our working directory.
$ mkdir channel-id-gfg
$ cd channel-id-gfg
Step 2: Use the npm init command to create a package.json file for our project.
$ npm init
OR
$ npm init -y /* For auto add the required field */
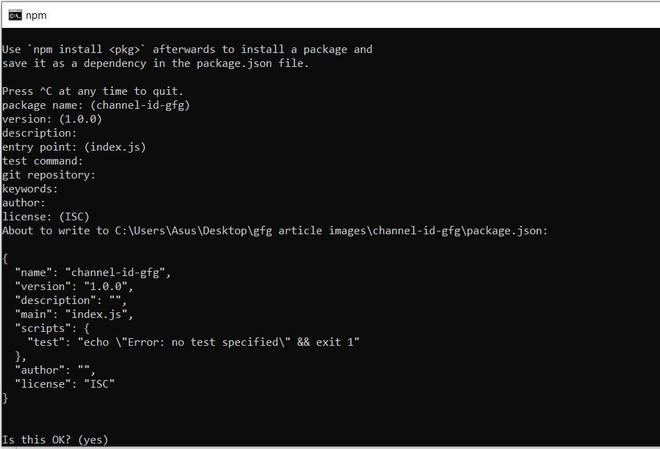
Note: Keep pressing enter and enter “yes/no” accordingly at the terminal line.
Step 3: Installing the Express.js and @gonetone/get-youtube-id-by-url module. Now in your channel-id-gfg(name of your folder) folder type the following command line:
npm install express @gonetone/get-youtube-id-by-url
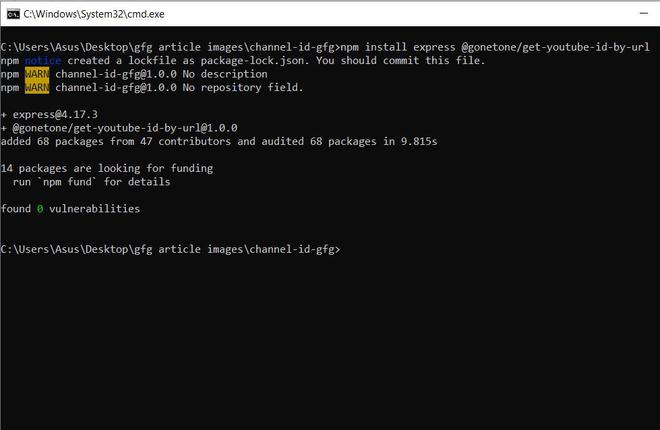
Step 4: Creating index.js and index.html files, our project structure will look like this.
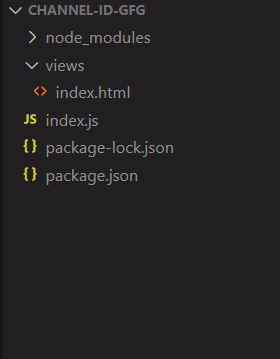
Step 5: Creating a basic server. Write down the following code in the index.js file.
index.js
const express = require( 'express' );
const app = express();
app.get( '/' , (req , res)=>{
res.send( "GeeksforGeeks" );
});
app.listen(4000 , ()=>{
console.log( "server is running on port 4000" );
});
|
Output:
GeeksforGeeks
Step 6: Now let’s implement the functionality by which we get the YouTube channel Id. Here we are using the channelId method, which is available in @gonetone/get-youtube-id-by-url.
index.html
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" />
< meta http-equiv = "X-UA-Compatible" content = "IE=edge" />
< meta name = "viewport" content =
"width=device-width, initial-scale=1.0" />
< title >YouTube Channel Id</ title >
< style >
h1 {
color: green;
}
input {
width: 200px;
height: 20px;
margin: 10px;
}
</ style >
</ head >
< body >
< center >
< h1 >GeeksforGeeks</ h1 >
< form method = "post" action = "/channel-id" >
< input
type = "text"
name = "url"
placeholder = "Enter channel URL"
required
/>
< br />
< button type = "submit" >Get Channel Id</ button >
</ form >
</ center >
</ body >
</ html >
|
index.js
const express = require( "express" );
const { channelId } = require( "@gonetone/get-youtube-id-by-url" );
const bodyParser = require( "body-parser" );
const app = express();
app.use(
bodyParser.urlencoded({
extended: true ,
})
);
app.get( "/" , (req, res) => {
res.sendFile(__dirname + "/views/index.html" );
});
app.post( "/channel-id" , (req, res) => {
const url = req.body.url;
channelId(url)
.then((id) => {
const response =
`<center><h2>Channel Id is - ${id}</h2><center>`;
res.send(response);
})
. catch ((err) => {
res.send( "Some error occurred" );
});
});
app.listen(4000, () => {
console.log( "Server running on port 4000" );
});
|
Step 7: Run the server using the following command.
node index.js
Output: Now open http://localhost:4000 on your browser to see the below output.
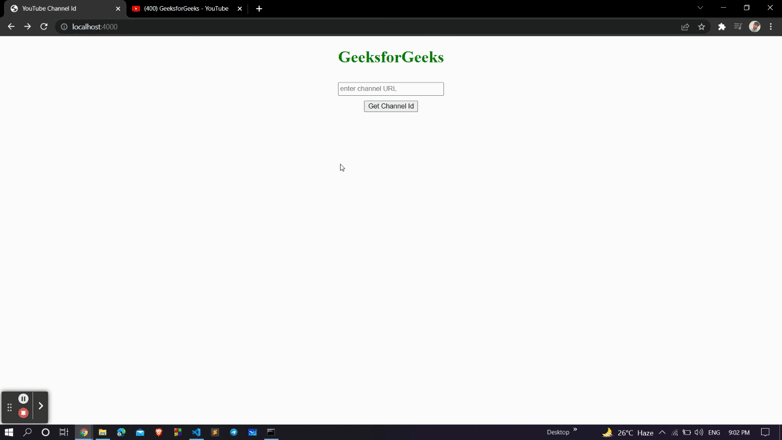
Reference: https://www.npmjs.com/package/@gonetone/get-youtube-id-by-url
Share your thoughts in the comments
Please Login to comment...