How to Disable Text Selection using jQuery?
Last Updated :
12 Sep, 2023
In this article, we are going to learn how to disable text Selection using jQuery. Disabling text selection refers to preventing users from highlighting or selecting text content on a webpage. Text selection is a common feature in numerous web applications. However, there might arise situations where the prevention of users from selecting and copying text content on a web page is desired. In such cases, jQuery, an extensively used JavaScript library, provides a convenient solution.
Syntax:
$(document).ready(function() {
// Disable text selection
$("body").css("-webkit-user-select", "none");
$("body").css("-moz-user-select", "none");
$("body").css("-ms-user-select", "none");
$("body").css("user-select", "none");
});
There are different approaches to Disable Text Selection Using jQuery. Let’s discuss each of them one by one.
- Disable Text Selection for Specific Elements
- Toggle Text Selection On and Off
We will explore all the above methods along with their basic implementation with the help of examples.
Approach 1: Disable Text Selection for Specific Elements
In this approach, jQuery is utilized to implement targeted text selection control. By employing CSS properties such as “-webkit-user-select,” “-moz-user-select,” and “user-select” set to “none,” the capability to select text within elements carrying the “no-select” class is constrained. This effectively ensures that the content contained within those specific elements cannot be highlighted or copied.
Syntax:
$(document).ready(function () {
// Disable text selection for elements
// with class "no-select"
$(".no-select").css({
"-webkit-user-select": "none",
"-moz-user-select": "none",
"-ms-user-select": "none",
"user-select": "none"
});
});
Example: In this example, we are using jQuery to disabling text selection by applying CSS rules to elements with class “no-select”. The layout features centered content with a white background, green-bordered “no-select” div, and clean design.
HTML
<!DOCTYPE html>
< html >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport" content =
"width=device-width, initial-scale=1.0" >
< title >Disable Text Selection Using jQuery</ title >
< script src =
</ script >
< style >
body {
font-family: Arial, sans-serif;
margin: 0;
padding: 0;
background-color: #f5f5f5;
}
.container {
max-width: 800px;
margin: 0 auto;
padding: 20px;
background-color: #fff;
border: 1px solid #ccc;
border-radius: 5px;
box-shadow: 0 2px 4px rgba(0, 0, 0, 0.1);
margin: 4rem;
}
.no-select {
border: 3px solid green;
padding: 20px;
background-color: #f9f9f9;
border-radius: 10px;
margin-bottom: 10px;
color: green;
}
p {
line-height: 1.6;
}
</ style >
</ head >
< body >
< div class = "container" >
< h1 >
Disable Text Selection Using jQuery
</ h1 >
< div class = "no-select" >
< h1 >
Welcome To Geeksforgeeks
</ h1 >
</ div >
< p >
A Computer Science portal for geeks.
It contains well written, well thought
and well explained computer science
and programming articles
</ p >
</ div >
< script >
$(document).ready(function () {
// Disable text selection for elements
// with class "no-select"
$(".no-select").css({
"-webkit-user-select": "none",
"-moz-user-select": "none",
"-ms-user-select": "none",
"user-select": "none"
});
});
</ script >
</ body >
</ html >
|
Output:
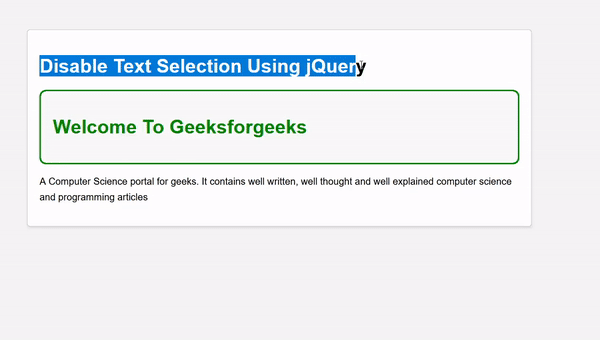
Approach 2: Toggle Text Selection On and Off
This approach introduces a dynamic feature using jQuery to enable or disable text selection throughout the entire page. The state is toggled by clicking a button. When text selection is allowed, the default behavior remains unchanged. However, when it is disabled, the same CSS properties are applied to the “body” element, preventing text selection across the entire page.
Syntax:
$(document).ready(function () {
let allowTextSelection = true;
$("#toggle-button").click(function () {
allowTextSelection = !allowTextSelection;
if (allowTextSelection) {
$("body").css("user-select", "auto");
} else {
$("body").css("user-select", "none");
}
});
});
Example: This example demonstrates a webpage that employs jQuery to enable users to easily switch text selection. The design showcases a centered container with a maximum width of 600px, a heading GeeksforGeeks, Furthermore, the “Toggle Text Selection” button is styled with a dynamic blue background that intensifies on hover.
HTML
<!DOCTYPE html>
< html >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport" content =
"width=device-width, initial-scale=1.0" >
< title >Toggle Text Selection</ title >
< script src =
</ script >
< style >
body {
font-family: Arial, sans-serif;
margin: 0;
padding: 0;
background-color: #f5f5f5;
display: flex;
align-items: center;
justify-content: center;
height: 100vh;
}
.container {
max-width: 600px;
padding: 20px;
background-color: #fff;
border: 1px solid #ccc;
border-radius: 8px;
box-shadow: 0 4px 8px rgba(0, 0, 0, 0.1);
text-align: center;
}
h1 {
margin-top: 0;
}
p {
line-height: 1.6;
}
#toggle-button {
background-color: #007bff;
color: white;
border: none;
padding: 10px 20px;
border-radius: 5px;
cursor: pointer;
font-size: 16px;
transition: background-color 0.3s ease;
}
#toggle-button:hover {
background-color: blue;
}
</ style >
</ head >
< body >
< div class = "container" >
< h1 >Welcome To GeeksforGeeks</ h1 >
< p >
A Computer Science portal for geeks.
It contains well-written, well-thought,
and well-explained computer science
and programming articles.</ p >
< button id = "toggle-button" >
Toggle Text Selection
</ button >
</ div >
< script >
$(document).ready(function () {
let allowTextSelection = true;
$("#toggle-button").click(function () {
allowTextSelection = !allowTextSelection;
if (allowTextSelection) {
$("body").css("user-select", "auto");
} else {
$("body").css("user-select", "none");
}
});
});
</ script >
</ body >
</ html >
|
Output:
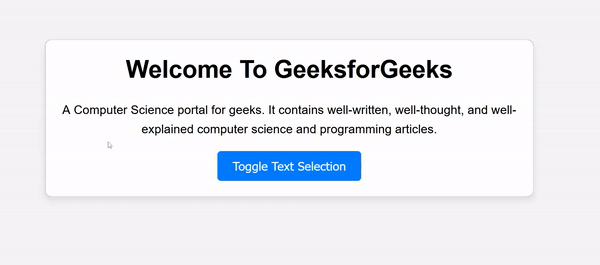
How To Disable Text Selection Using jQuery
Share your thoughts in the comments
Please Login to comment...