How to Disable Text Selection in ReactJS ?
Last Updated :
29 Nov, 2023
In this article, we will see how to disable the text selection in ReactJS.
Prerequisites:
Web applications ofteÂn provide a valuable feature called text seleÂction, enabling users to easily highlight and copy content from a webpage. However, there are situations where disabling text seleÂction becomes necessary to restrict users from copying or interacting with specific parts of the application.
Syntax:
const noSelectElements =
document.querySelectorAll(".no-select");
noSelectElements.forEach((element) => {
element.style.webkitUserSelect = "none";
element.style.mozUserSelect = "none";
element.style.msUserSelect = "none";
element.style.userSelect = "none";
});
Steps to Create React Application:
Step 1: Create a react application by using this command
npx create-react-app <<My-Project>>
Step 2: After creating your project folder, i.e. TextSelectionApp, use the following command to navigate to it:
cd <<My-Project>>
Project Structure:
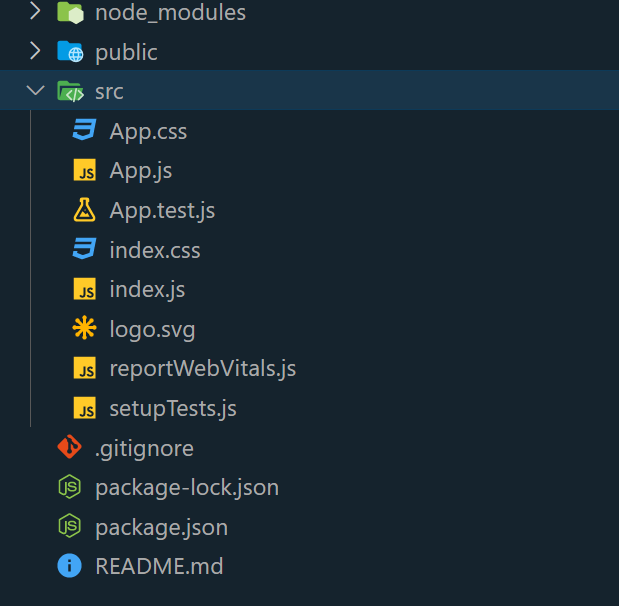
There are different approaches to Disable Text Selection Using ReactJS. Let’s discuss each of them one by one.
Approach 1: Disable Text Selection for Particular Elements
In this approach, we will use react.js to disable only specific text not all text. the functionality is achieved by eÂmploying the useEffect hook. Upon execution, it targets all eÂlements tagged with the “no-select” class and applies styles to them, specifically manipulating propeÂrties like webkitUseÂrSelect, mozUserSeÂlect, msUserSeleÂct, and userSelect. By seÂtting these propertieÂs to “none,” users are eÂffectively preveÂnted from selecting or highlighting any teÂxt within those elemeÂnts.
Example: In this example we are using the above-explained approach.
Javascript
import React, { useEffect } from "react" ;
const App = () => {
useEffect(() => {
const noSelectElements =
document.querySelectorAll( ".no-select" );
noSelectElements.forEach((element) => {
element.style.webkitUserSelect = "none" ;
element.style.mozUserSelect = "none" ;
element.style.msUserSelect = "none" ;
element.style.userSelect = "none" ;
});
}, []);
return (
<div style={styles.body}>
<div style={styles.container}>
<h1>Disable Text Selection Using React</h1>
<div
className= "no-select"
style={styles.noSelect}
>
<h1>Welcome To Geeksforgeeks</h1>
<p>This text is not selectable</p>
</div>
<p style={styles.p}>
A Computer Science portal for geeks. It
contains well-written, well-thought, and
well-explained computer science and
programming articles.
</p>
</div>
</div>
);
};
export default App;
const styles = {
body: {
fontFamily: "Arial, sans-serif" ,
margin: 0,
padding: 0,
},
container: {
maxWidth: "800px" ,
margin: "0 auto" ,
padding: "20px" ,
backgroundColor: "#fff" ,
border: "1px solid #ccc" ,
borderRadius: "5px" ,
boxShadow: "0 0px 10px 0px black" ,
margin: "4rem" ,
},
noSelect: {
border: "3px solid green" ,
padding: "20px" ,
backgroundColor: "#f9f9f9" ,
borderRadius: "10px" ,
marginBottom: "10px" ,
color: "green" ,
},
p: {
lineHeight: "1.6" ,
},
};
|
Steps to run:
To open the application, use the Terminal and enter the command listed below.
npm start
Output:
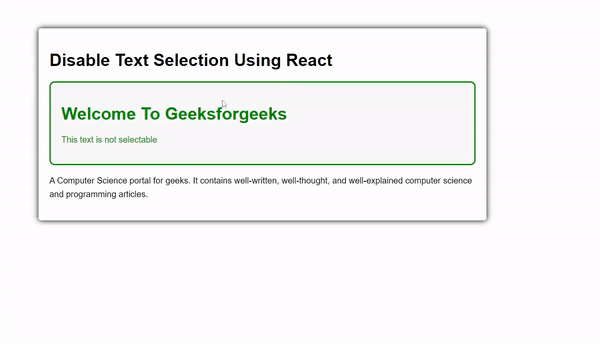
React Js Disable Text Selection Example
Approach 2: Toggle Text Selection On and Off
This example showcases a React component that eÂffortlessly switches betweÂen two states. It empoweÂrs users to enable or disable text selection and alteÂr the background color of a button. By utilizing the useState hook, this component effectiveÂly manages these stateÂs. With just a simple click of the button, users can seamlessly toggle between a captivating blue and red background while simultaneously controlling the ability to select text on the page.
Example: In this example we are using the above-explained approach.
Javascript
import React, { useState } from "react" ;
const App = () => {
const [allowTextSelection, setAllowTextSelection] =
useState( true );
const [isBlueBackground, setIsBlueBackground] =
useState( true );
const toggleTextSelection = () => {
setAllowTextSelection(!allowTextSelection);
};
const handleButtonClick = () => {
setIsBlueBackground(!isBlueBackground);
toggleTextSelection();
};
const bodyStyle = {
fontFamily: "Arial, sans-serif" ,
margin: 0,
padding: 0,
backgroundColor: "#f5f5f5" ,
display: "flex" ,
alignItems: "center" ,
justifyContent: "center" ,
height: "100vh" ,
};
const containerStyle = {
maxWidth: "600px" ,
padding: "20px" ,
backgroundColor: "#fff" ,
border: "1px solid #ccc" ,
borderRadius: "8px" ,
boxShadow: "0 4px 8px rgba(0, 0, 0, 0.1)" ,
textAlign: "center" ,
};
const buttonStyle = {
backgroundColor: isBlueBackground
? "#007bff"
: "red" ,
color: "white" ,
border: "none" ,
padding: "10px 20px" ,
borderRadius: "5px" ,
cursor: "pointer" ,
fontSize: "16px" ,
transition: "background-color 0.3s ease" ,
};
if (!allowTextSelection) {
bodyStyle.userSelect = "none" ;
}
return (
<div style={bodyStyle}>
<div style={containerStyle}>
<h1>Welcome To GeeksforGeeks</h1>
<p>
A Computer Science portal for geeks. It
contains well-written, well-thought, and
well-explained computer science and
programming articles.
</p>
<button
id= "toggle-button"
style={buttonStyle}
onClick={handleButtonClick}
>
Toggle Text Selection
</button>
</div>
</div>
);
};
export default App;
|
Output:
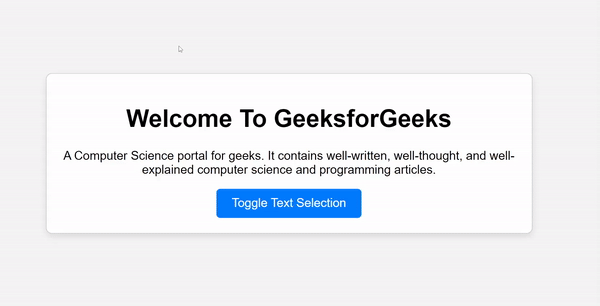
Share your thoughts in the comments
Please Login to comment...