How to create Breadcrumb Navigation Using React ?
Last Updated :
19 Feb, 2024
Breadcrumbs are useful in navigation and provide users with links representing their path through a website or application. In this tutorial, we’ll create a simple e-commerce website with product listings and detail pages, incorporating breadcrumbs for a better user experience.
Output Preview: Let us have a look at how the final output will look like.
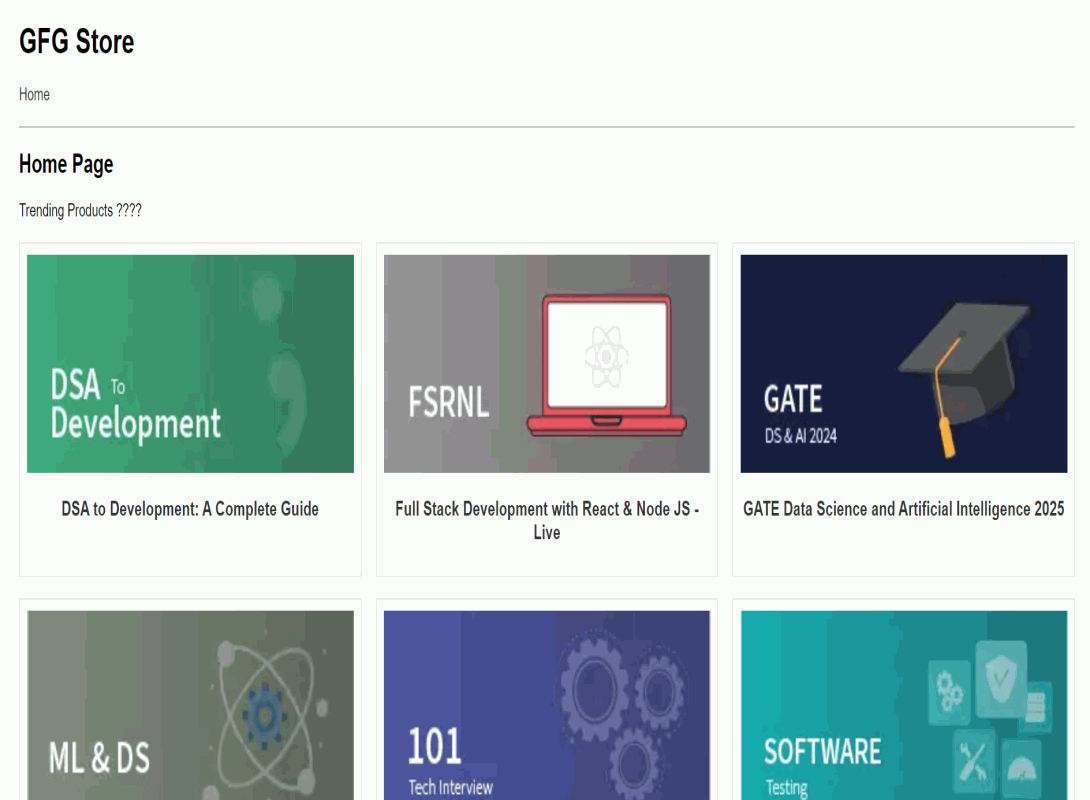
Prerequisites:
Approach to create Breadcrumb Navigation
We’ll create a React application with three main pages: Home, Product Listing, and Product Detail. The breadcrumbs will dynamically adjust based on the current route, providing users with a clear path to their current location.
Steps to Create the Breadcrumb Navigation in React
Step 1: Set Up Your React App with Vite
npm create vite@latest
- Project name: Enter breadcrumbs.
- Select a framework: Choose React.
- Select a variant: Choose JavaScript + SWC.
Step 2: Navigate to the Project Directory
cd breadcrumbs
Step 3: Install the project dependencies using:
npm install
Step 4: Install React Router
npm install react-router-dom
Project Structure:
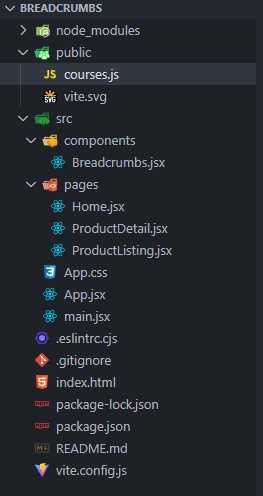
project structure
The updated dependencies in package.json file will look like:
"dependencies": {
"react": "^18.2.0",
"react-dom": "^18.2.0",
"react-router-dom": "^6.22.0"
}
"devDependencies": {
"@types/react": "^18.2.55",
"@types/react-dom": "^18.2.19",
"@vitejs/plugin-react-swc": "^3.5.0",
"eslint": "^8.56.0",
"eslint-plugin-react": "^7.33.2",
"eslint-plugin-react-hooks": "^4.6.0",
"eslint-plugin-react-refresh": "^0.4.5",
"vite": "^5.1.0"
}
Example: Create the required files and the following code.
Javascript
import { Link, useLocation } from "react-router-dom" ;
const Breadcrumbs = () => {
const location = useLocation();
const pathnames = location.pathname.split( "/" ).filter((x) => x);
let breadcrumbPath = "" ;
return (
<div className= "breadcrumbs" >
<Link to= "/" >Home</Link>
{pathnames.map((name, index) => {
breadcrumbPath += `/${name}`;
const isLast = index === pathnames.length - 1;
console.log(pathnames, breadcrumbPath);
return isLast ? (
<span key={breadcrumbPath}> / {name}</span>
) : (
<span key={breadcrumbPath}>
{ " " }
/ <Link to={breadcrumbPath}>{name}</Link>
</span>
);
})}
</div>
);
};
export default Breadcrumbs;
|
Javascript
import { useEffect, useState } from "react" ;
import { Link } from "react-router-dom" ;
import courses from "../../public/courses.js" ;
const Home = () => {
const [trendingProducts, setTrendingProducts] = useState([]);
useEffect(() => {
const slicedTrendingProducts = courses.slice(0, 6);
setTrendingProducts(slicedTrendingProducts);
}, []);
return (
<div>
<h2>Home Page</h2>
<span>Trending Products ????</span>
<div className= "product-grid" >
{trendingProducts?.map((product) => (
<div key={product.id} className= "product-card" >
<Link to={`/products/${product.id}`}>
<img src={product.thumbnail} alt={product.title} />
<h3>{product.title}</h3>
</Link>
</div>
))}
</div>
<Link to= "/products" >
<button style={{ width: "100%" , padding: 10 }}>
View All Products
</button>
</Link>
</div>
);
};
export default Home;
|
Javascript
import { useEffect, useState } from "react" ;
import { useParams } from "react-router-dom" ;
import courses from "../../public/courses" ;
const ProductDetail = () => {
const { id } = useParams();
const [product, setProduct] = useState( null );
useEffect(() => {
const selectedProduct = courses.find(
(course) => course.id.toString() === id
);
if (selectedProduct) {
setProduct(selectedProduct);
} else {
console.error(`Product with id ${id} not found`);
}
}, [id]);
return (
<div>
<h2>Product Detail Page</h2>
{product ? (
<div>
<img src={product.thumbnail} alt= "Product" />
<h3>{product.title}</h3>
<h3>$ {product.price}</h3>
<p>{product.description}</p>
</div>
) : (
<p>Loading...</p>
)}
</div>
);
};
export default ProductDetail;
|
Javascript
import { useEffect, useState } from "react" ;
import { Link } from "react-router-dom" ;
import courses from "../../public/courses" ;
const ProductListing = () => {
const [products, setProducts] = useState([]);
console.log(products);
useEffect(() => {
setProducts(courses);
}, []);
return (
<div>
<h2>Product Listing</h2>
<div className= "product-grid" >
{products?.map((product) => (
<div key={product.id} className= "product-card" >
<Link to={`/products/${product.id}`}>
<img src={product.thumbnail} alt={product.title} />
<h3>{product.title}</h3>
</Link>
</div>
))}
</div>
</div>
);
};
export default ProductListing;
|
Javascript
import { BrowserRouter as Router, Routes, Route } from "react-router-dom" ;
import Breadcrumbs from "./components/Breadcrumbs" ;
import Home from "./pages/Home" ;
import ProductListing from "./pages/ProductListing" ;
import ProductDetail from "./pages/ProductDetail" ;
import "./App.css" ;
const App = () => {
return (
<Router>
<div className= "app" >
<h1>GFG Store</h1>
<Breadcrumbs />
<hr />
<Routes>
<Route path= "/" element={<Home />} />
<Route path= "/products" element={<ProductListing />} />
<Route path= "/products/:id" element={<ProductDetail />} />
</Routes>
</div>
</Router>
);
};
export default App;
|
Javascript
import React from "react" ;
import ReactDOM from "react-dom/client" ;
import App from "./App.jsx" ;
ReactDOM.createRoot(document.getElementById( "root" )).render(
<React.StrictMode>
<App />
</React.StrictMode>
);
|
Javascript
const courses = [
{
id: 1,
thumbnail:
title: "DSA to Development: A Complete Guide" ,
description:
"Learn the essentials of Data Structures and Algorithms and transition seamlessly into Full Stack Development with this comprehensive guide." ,
price: 19999,
},
{
id: 2,
thumbnail:
title: "Full Stack Development with React & Node JS - Live" ,
description:
"Immerse yourself in a live course that covers everything from frontend development with React to server-side scripting with Node JS." ,
price: 14999,
},
{
id: 3,
thumbnail:
title: "GATE Data Science and Artificial Intelligence 2025" ,
description:
"Prepare for the GATE exam with a focus on Data Science and Artificial Intelligence, and stay ahead in the rapidly evolving field of technology." ,
price: 9999,
},
{
id: 4,
thumbnail:
title: "Complete Machine Learning & Data Science Program" ,
description:
"Master the art of machine learning and data science through a comprehensive program that covers everything from fundamentals to advanced techniques." ,
price: 5999,
},
{
id: 5,
thumbnail:
title: "DSA to Development: A Complete Guide" ,
description:
"Enhance your problem-solving skills, ace technical interviews, and become a proficient developer with this comprehensive guide." ,
price: 19999,
},
{
id: 6,
thumbnail:
title:
"Complete Guide to Software Testing and Automation: Master Java, Selenium and Cucumber" ,
description:
"Become an expert in software testing and automation by mastering Java, Selenium, and Cucumber with this comprehensive guide." ,
price: 14999,
},
{
id: 7,
thumbnail:
title: "JAVA Backend Development - Live" ,
description:
"Join a live course and delve into the world of JAVA backend development, learning the intricacies of building robust and scalable server-side applications." ,
price: 9999,
},
{
id: 8,
thumbnail:
title: "Complete Interview Preparation" ,
description:
"Prepare thoroughly for technical interviews, including coding rounds, system design, and behavioral interviews, with this all-inclusive guide." ,
price: 5999,
},
{
id: 9,
thumbnail:
title: "Data Structures & Algorithms in Python - Self Paced" ,
description:
"Learn data structures and algorithms at your own pace, using Python as the programming language, with this self-paced course." ,
price: 19999,
},
{
id: 10,
thumbnail:
title: "Data Structures and Algorithms - Self Paced" ,
description:
"Take a deep dive into data structures and algorithms through a self-paced course that covers essential concepts for programming and problem-solving." ,
price: 14999,
},
];
export default courses;
|
CSS
.app {
padding : 0 20px ;
font-family : sans-serif ;
}
.product-grid {
display : grid;
grid-template-columns: 1 fr 1 fr 1 fr;
grid-gap: 20px ;
padding : 20px 0 ;
}
.product-card {
border : 1px solid #ddd ;
padding : 10px ;
text-align : center ;
}
.product-card a {
text-decoration : none ;
color : #333 ;
}
.product-card img {
width : 100% ;
height : 250px ;
object-fit: cover;
max-height : 200px ;
}
.breadcrumbs {
margin-bottom : 20px ;
font-size : 16px ;
text-transform : capitalize ;
}
.breadcrumbs a {
text-decoration : none ;
color : #333 ;
}
.breadcrumbs span {
color : #999 ;
}
|
Steps to run the application:
Step 1: Type the following command in the terminal.
npm run dev
Step 2: Open web browser and type the following URL.
http://localhost:5173/
Output:
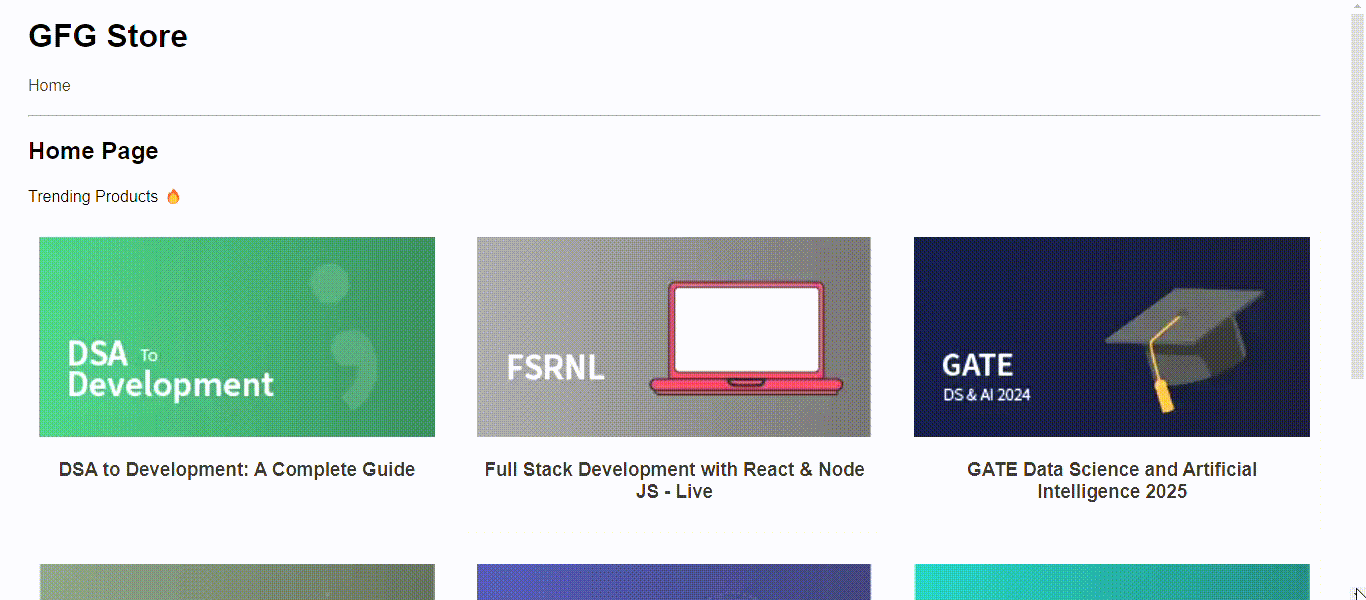
output
Share your thoughts in the comments
Please Login to comment...