How to Convert Callback to Promise in JavaScript ?
Last Updated :
26 Mar, 2024
Asynchronous programming in JavaScript often involves the use of callbacks. However, callbacks can lead to callback hell and make the code harder to read and maintain. Promises provide a cleaner way to handle asynchronous operations. Converting existing callback-based code to use promises can improve readability and maintainability.
Using the Promise constructor
This approach involves manually creating a promise around the asynchronous operation. It requires creating a new promise and resolving or rejecting it based on the outcome of the asynchronous operation.
Syntax:
function promisifiedFunction(arg1, arg2) {
return new Promise((resolve, reject) => {
myFunctionWithCallback(arg1, arg2, (error, result) => {
if (error) {
reject(error);
} else {
resolve(result);
}
});
});}
Example: In this, the promise-based function is used to fetch data from the API.
JavaScript
function fetchDataWithCallback(url, callback) {
setTimeout(() => {
const data = {
message: 'Data fetched successfully',
url: url
};
callback(null, data);
}, 1000);
function fetchDataPromise(url) {
return new Promise((resolve, reject) => {
fetchDataWithCallback(url, (error, data) => {
if (error) {
reject(error);
} else {
resolve(data);
}
});
});
}
fetchDataPromise('https://api.example.com/data')
.then(data => console.log('Data:', data))
.catch(error => console.error('Error:', error));
Output:
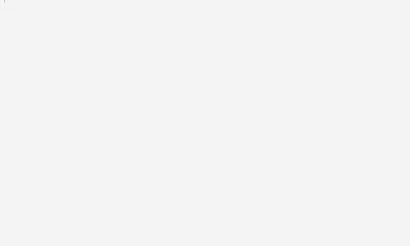
Output
Using a custom promise-based wrapper
This approach involves creating a custom wrapper function around the existing callback-based function.
Inside the wrapper function, a new promise is created, and the original function is called with a callback.
The promise is resolved or rejected based on the callback’s result.
Syntax:
function promisifiedFunction(arg1, arg2) {
return new Promise((resolve, reject) => {
originalFunctionWithCallback(arg1, arg2, (error, result) => {
if (error) {
reject(error);
} else {
resolve(result);
}
});
});}
Example: In this, the promise-based function is used to fetch data from the API.
JavaScript
function fetchDataWithCallback(url, callback) {
setTimeout(() => {
const data = {
message: 'Data fetched successfully',
url: url
};
callback(null, data);
}, 1000);
}
function fetchDataPromise(url) {
return new Promise((resolve, reject) => {
fetchDataWithCallback(url, (error, data) => {
if (error) {
reject(error);
} else {
resolve(data);
}
});
});
}
fetchDataPromise('https://api.example.com/data')
.then(data => console.log('Data:', data))
.catch(error => console.error('Error:', error));
Output:
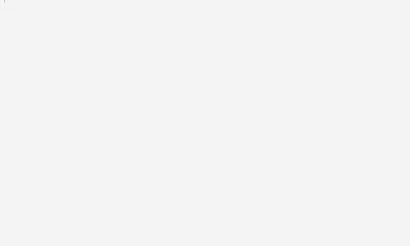
Output
Share your thoughts in the comments
Please Login to comment...