How to Change the Button Label when Clicked using JavaScript ?
Last Updated :
07 Mar, 2024
Changing the Label of the Button element when clicked in JavaScript can be used to provide more information to the user such as the text of the Submit button will change to the Submitted as soon as the form submission is completed.
The below approaches can be used to accomplish this task:
Changing Button Label Using innerHTML property
The innerHTML property in JavaScript is used to dynamically change the content inside any element. The changeLabel function, which is triggered by the button’s onclick attribute, selects the button and updates its innerHTML, changing the button label.
Syntax:
selctedHTMLElement.innerHTML = "contentToAppend";
Example: The below example uses innerHTML property to change the button label when clicked in JavaScript.
HTML
<!DOCTYPE html>
< html >
< head >
< title >
Change Button Label
</ title >
< style >
body {
font-family: Arial, sans-serif;
text-align: center;
margin: 40px;
}
h1 {
color: green;
}
h3 {
color: #333;
}
button {
padding: 10px 20px;
font-size: 16px;
background-color: #4CAF50;
color: white;
border: none;
border-radius: 4px;
cursor: pointer;
}
button:hover {
background-color: #45a049;
}
</ style >
</ head >
< body >
< h1 >GeeksforGeeks</ h1 >
< h3 >
Using innerHTML property
</ h3 >
< button onclick = "changeLabel()" >
Click me
</ button >
< script >
function changeLabel() {
const button =
document.querySelector('button');
button.innerHTML = 'Button Clicked!';
}
</ script >
</ body >
</ html >
|
Output:
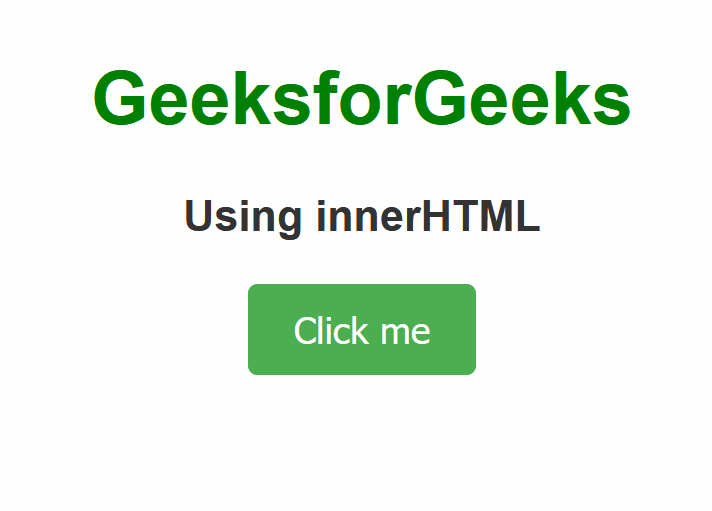
Changing Button Label Using innerText property
The innerText property in JavaScript is used to dynamically modify the text content within any element. The toggleLabel function, triggered by the button’s onclick event, checks the current label and toggles between two states, changing the button’s text.
Syntax:
selectedHTMLElement.innerText = text
Example: The below example uses innerText property to change the button label when clicked in JavaScript.
HTML
<!DOCTYPE html>
< html >
< head >
< title >
Button Label
</ title >
< style >
body {
font-family: Arial, sans-serif;
text-align: center;
margin: 40px;
}
button {
padding: 10px 20px;
font-size: 16px;
cursor: pointer;
}
h1 {
color: green;
}
</ style >
</ head >
< body >
< h1 >GeeksforGeeks</ h1 >
< h3 >Using innerText property</ h3 >
< button onclick = "toggleLabel()" >
Click me
</ button >
< script >
function toggleLabel() {
const btn =
document.querySelector('button');
if (btn.innerText === 'Click me') {
btn.innerText = 'Label Changed!';
} else {
btn.innerText = 'Click me';
}
}
</ script >
</ body >
</ html >
|
Output:
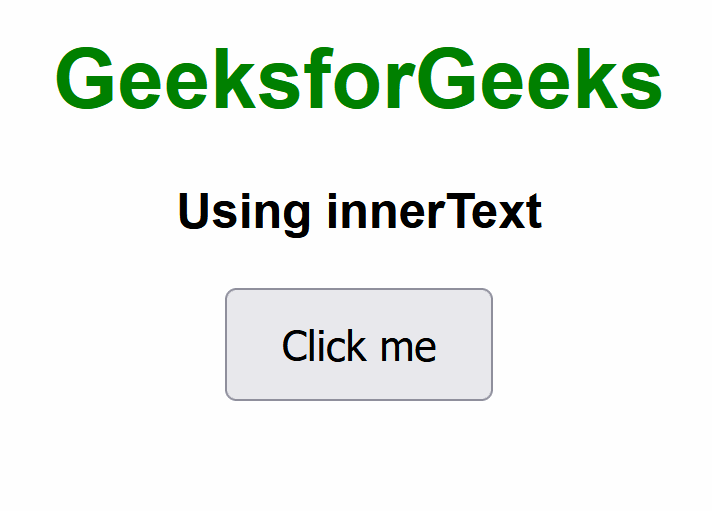
Share your thoughts in the comments
Please Login to comment...