How to Change Button Label in Alert Box using JavaScript ?
Last Updated :
16 Apr, 2024
In JavaScript, the alert method is used to display an alert box with a message. By default, the alert box contains an “OK” button. We cannot directly update the default alert box, However, we can customize the button label by creating a custom alert box using HTML, CSS, and JavaScript.
Approach
- Create the UI of the web page using different HTML elements, and a stylish alert box using CSS.
- Define a hidden HTML div element for the custom alert box. This div will contain the message and the custom button.
- Use JavaScript to display the custom alert box when needed. Set the message and the button label dynamically.
- Attach an event listener to the custom button to handle its click event.
Example: This example shows how we can implement this approach.
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport"
content="width=device-width, initial-scale=1.0">
<title>Custom Alert Box</title>
<style>
h1 {
color: green;
}
body {
text-align: center;
}
.custom-alert {
display: none;
position: fixed;
top: 40%;
left: 50%;
transform: translate(-50%, -50%);
background-color: #f8d7da;
color: #721c24;
padding: 10px;
border: 1px solid #f5c6cb;
border-radius: 5px;
text-align: center;
}
</style>
</head>
<body>
<h1>GeeksForGeeks</h1>
<button onclick="showCustomAlert()">Show Custom Alert</button>
<div id="customAlert" class="custom-alert">
<p id="alertMessage"></p>
<button id="customButton">Close</button>
</div>
<script>
function showCustomAlert() {
const message = "This is a custom alert box!";
const buttonText = "Close me";
const alertBox = document.getElementById('customAlert');
const alertMessage = document.getElementById('alertMessage');
const customButton = document.getElementById('customButton');
// Set the message and button label
alertMessage.innerText = message;
customButton.innerText = buttonText;
// Show the custom alert box
alertBox.style.display = 'block';
// Handle button click
customButton.addEventListener('click', function () {
alertBox.style.display = 'none'; // Hide the alert box
});
}
</script>
</body>
</html>
Output:
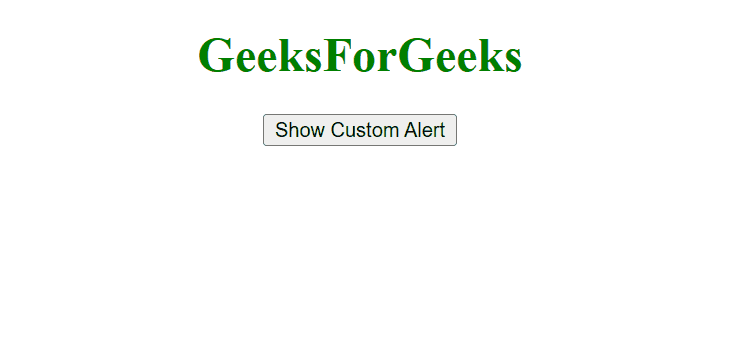
Share your thoughts in the comments
Please Login to comment...