How to check a button is clicked or not in JavaScript ?
Last Updated :
07 Dec, 2023
In this article, we will see how to check whether a button is clicked or not using JavaScript.
There are two methods to check whether a button is clicked or not, these are:
First, we will create a button, then use document.querySelector() to select the button element, and after that apply onclick event to check whether the button is clicked or not.
Example: In this example, we have used above explained approach.
HTML
<!DOCTYPE html>
< html >
< head >
< title >
How to check a button is clicked
or not in JavaScript?
</ title >
< style >
body {
text-align: center;
}
h1 {
color: green;
}
input[type="button"] {
padding: 5px 10px;
margin-top: 10px;
}
</ style >
</ head >
< body >
< h1 >GeeksforGeeks</ h1 >
< h3 >
How to check a button is clicked
or not in JavaScript?
</ h3 >
< input type = "button"
class = "button" value = "Button"
onclick = "myGeeks()" >
< script >
function myGeeks() {
document.querySelector(".button").
onclick = function () {
alert("Button Clicked");
}
}
</ script >
</ body >
</ html >
|
Output:
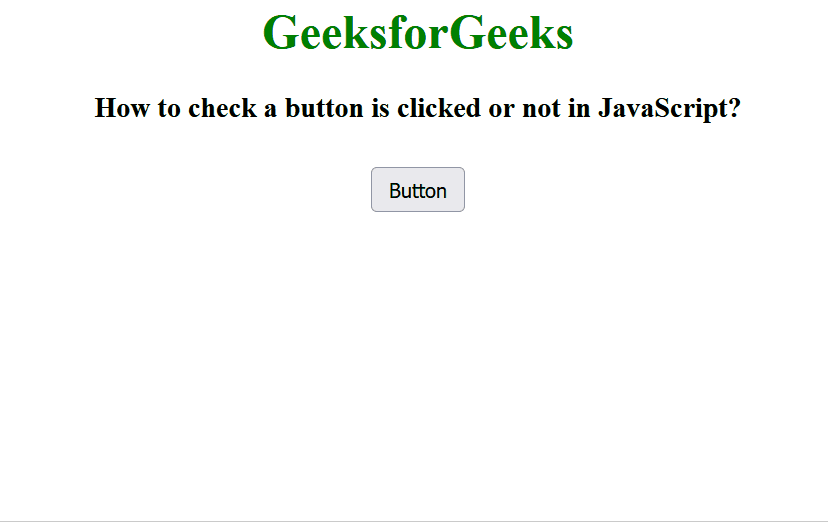
Output
Method 2: Using click Event
First, we will create a button, then use document.querySelector() to select the button element, and after that apply addEventListener() and click event to check whether the button is clicked or not.
Example: In this example, we have use above explained approach.
HTML
<!DOCTYPE html>
< html >
< head >
< title >
How to check a button is clicked
or not in JavaScript?
</ title >
< style >
body {
text-align: center;
}
h1 {
color: green;
}
input[type="button"] {
padding: 5px 10px;
margin-top: 10px;
}
</ style >
</ head >
< body >
< h1 >GeeksforGeeks</ h1 >
< h3 >
How to check a button is clicked
or not in JavaScript?
</ h3 >
< input type = "button" class = "button"
value = "Button" onclick = "myGeeks()" >
< script >
function myGeeks() {
document.querySelector(".button")
.addEventListener("click", function () {
alert("Button Clicked");
});
}
</ script >
</ body >
</ html >
|
Output:
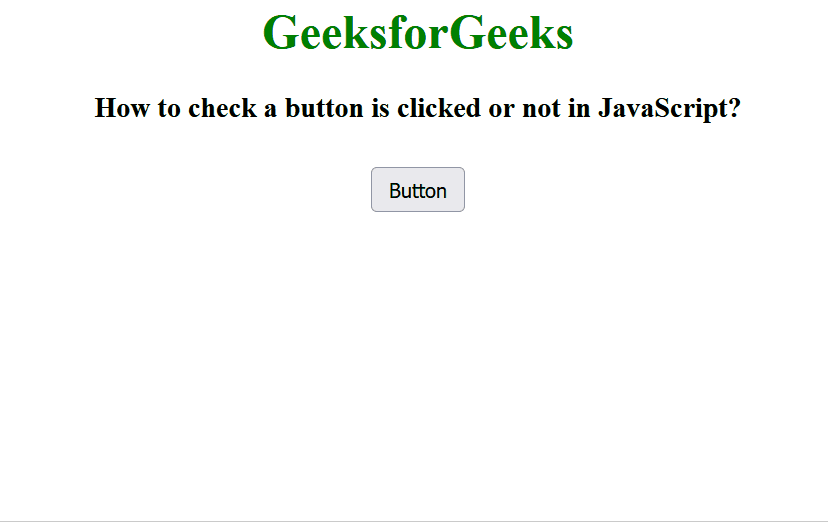
Share your thoughts in the comments
Please Login to comment...