How to Catch and Parse Errors Properly in React form Submission Handler ?
Last Updated :
12 Dec, 2023
We use forms a lot to get information from users, like their email, username, and password. Users send us this info through the forms, and we store it on the web server. The Form is an element that is used on a large scale in many websites which makes it an integral component. Creating a Form in ReactJS is similar to HTML but in HTML the form we create is static, but in React we could add many unique functionalities like validations, etc.
What is Formsy?
Formsy is an open-source library that is a form input-builder and validator for React. We can catch and parse errors using this library. It helps us to deal with these two crucial things:
- Validation and error messages
- Handling form submission
Steps to Create the React Application:
Step 1: Creating React ApplicationÂ
npx create-react-app appname
Step 2: After creating your project folder, Go into your project folder.
cd appname
Step 3: Now we can add Formsy for building forms and handling errors.
npm i formsy-react
Project Structure:
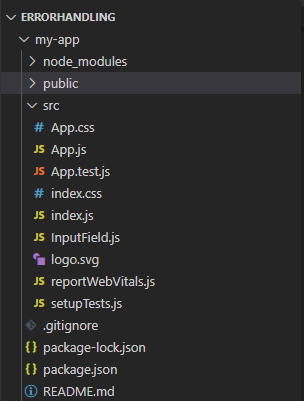
Project Structure
The updated dependencies in package.json file will look like:
"dependencies": {
"formsy-react": "^2.3.0",
"react": "^18.2.0",
"react-dom": "^18.2.0",
"react-scripts": "5.0.1",
"web-vitals": "^2.1.4",
}
Step 4: Create a file InputField.js which contains an HTML input tag. So that we can import it and use it in our forms(in App.js).
Example: First import withFormsy from formsy-react by writing the below code in InputField.js.
import { withFormsy } from 'formsy-react'
After importing withFormsy from formsy-react, we created an input field and added some common HTML attributes. We need to import Formsy from formsy-react.
import Formsy from 'formsy-react'
Javascript
import React, { useState } from 'react'
import './App.css'
import Formsy from 'formsy-react'
import InputField from './InputField'
const errors = {
isEmail: 'You have to type a valid email' ,
maxLength: 'You cannot type more than 25 characters' ,
minLength: 'You must type more than 6 characters' ,
}
function App() {
const [canSubmit, setCanSubmit] = useState( false );
const disableButton = () => {
setCanSubmit( false );
}
const enableButton = () => {
setCanSubmit( true );
}
const submit = () => {
console.log( 'Form is Submitted' );
}
return (
<div className= 'app' >
<h1>Form in React Js</h1>
<Formsy className= 'form'
onValidSubmit={submit}
onValid={enableButton}
onInvalid={disableButton}>
<InputField label= "First Name"
type= "text" name= "email"
validations= "maxLength:25"
validationErrors={errors}
placeholder= "Type your First Name"
required />
<InputField label= "Last Name"
type= "text" name= "lastname"
validations= "maxLength:25"
validationErrors={errors}
placeholder= "Type your last Name"
required />
<InputField label= "Email address"
type= "text" name= "email"
validations= "maxLength:30,isEmail"
validationErrors={errors}
placeholder= "Type your email address..."
required />
<InputField label= "Password"
type= "password" name= "password"
validations= "minLength:6"
validationErrors={errors}
placeholder= "Type your password..."
required />
<button type= "submit"
disabled={!canSubmit}>
Submit
</button>
</Formsy>
</div>
)
}
export default App
|
Javascript
import { withFormsy } from 'formsy-react'
import React from 'react'
class MyInput extends React.Component {
constructor(props) {
super (props)
this .changeValue = this .changeValue.bind( this )
}
changeValue(event) {
this .props.setValue(event.currentTarget.value);
}
render() {
const errorMessage = this .props.errorMessage;
return (
<div className= 'input-row' >
<label>
{ this .props.label}
{ this .props.isRequired ? '*' : null }
</label>
<input onChange={ this .changeValue}
type={ this .props.type}
placeholder={ this .props.placeholder}
value={ this .props.value || '' } />
<span>{errorMessage}</span>
</div>
)
}
}
export default withFormsy(MyInput)
|
We will wrap all input fields with the Formsy component which gives us some properties which help us with form validation. The properties that are helpful for us i.e. validations and validationError.
- validations: Here we can pass some validators separated by a comma. And with the validator, we can pass some arguments by using “:”.
- validationerrors: You can manually pass down errors to your form. It will show up when the requirement is not met.
In this way, we can add our own conditions, and error messages as per your requirements.
Wrapping with a Formsy tag also gives us functionalities like OnValidSubmit, OnValid, and OnInvalid which helps us in Form Submission.
- onValidSubmit: It ensures that what will be done after we submit the form with no errors, In this case, submit method will run and submits our form.
- onValid: It ensures the form can be only submitted when all input fields are valid. So in this case, if all the input fields are valid then we call the enableButton method which enables the submit button with the help of the state variable canSubmit defined above in Line 14.
- onInvalid: It ensures the form cannot be submitted when one of the input fields is invalid. So in this case, if one of the input fields is invalid then we call the disableButton method which disables the submit button with the help of a state variable canSubmit defined above in Line 14.Â
Step 5: Run the application using the following command from the root directory of the project:
npm start
Output: Open your browser and go to http://localhost:3000
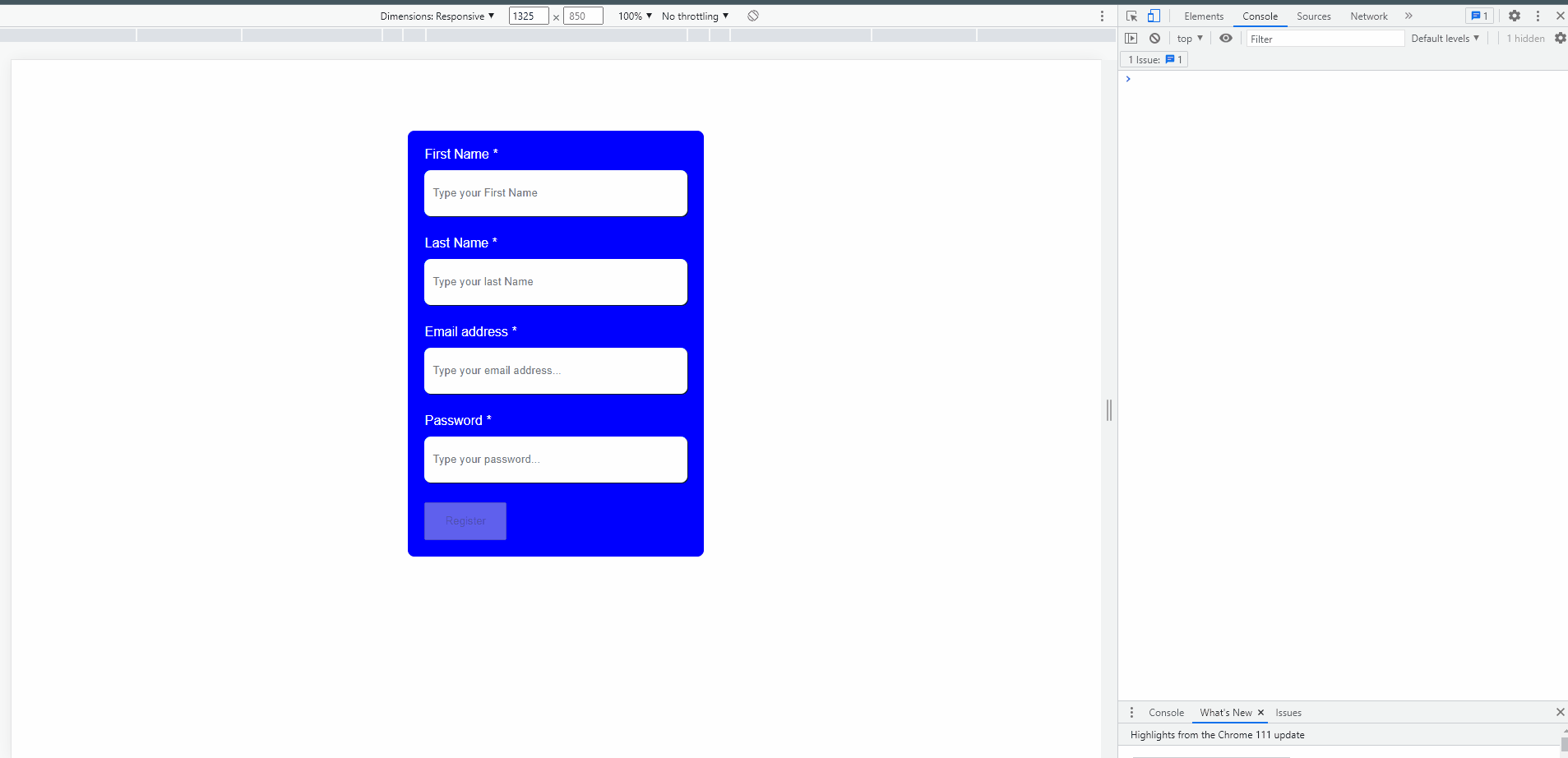
Output
Share your thoughts in the comments
Please Login to comment...