How to prevent duplicate submission in a form using jQuery?
Last Updated :
28 Mar, 2024
Preventing duplicate submission in a form using jQuery involves implementing measures to ensure that the form is only submitted once, regardless of how many times the user interacts with the submit button. To prevent duplicate form submissions in web applications using jQuery we can use various methods like One-time event binding and disabling submit button.
Use the below approaches to prevent duplicate submissions in a form using jQuery:
In this approach, jQuery’s .one() method ensures that the form submission event is bound only once, preventing duplicate submissions. Upon submission, it disables the form elements and displays a success message. Further form submissions are blocked after the initial submission, ensuring data integrity.
Example: Implementation of preventing duplicate submission in a form with jQuery using jQuery One-Time Event Binding.
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport"
content="width=device-width,
initial-scale=1.0">
<title>Prevent Duplicate Form Submission</title>
<style>
.navbar {
background-color: #333;
padding: 10px 0;
width: 100%;
top: 0;
z-index: 1000;
}
.logo-container {
display: flex;
justify-content: center;
align-items: center;
}
.logo {
width: 80px;
height: auto;
}
body {
margin: 0;
padding: 0;
display: flex;
flex-direction: column;
align-items: center;
min-height: 100vh;
}
#content {
display: flex;
flex-direction: column;
align-items: center;
}
h1 {
margin-top: 20px;
text-align: center;
}
form {
width: 300px;
margin-top: 20px;
padding: 20px;
border: 1px solid #ccc;
border-radius: 5px;
box-shadow: 0 0 10px rgba(0, 0, 0, 0.1);
}
input[type="text"],
input[type="password"],
button {
width: 100%;
margin-bottom: 10px;
padding: 10px;
border: 1px solid #ccc;
border-radius: 5px;
box-sizing: border-box;
}
button {
background-color: #007bff;
color: #fff;
cursor: pointer;
}
button:disabled {
opacity: 0.6;
cursor: not-allowed;
}
button:hover:enabled {
background-color: #0056b3;
}
#successMessage {
display: none;
color: green;
margin-top: 10px;
}
#submittedData {
display: none;
margin-top: 20px;
padding: 10px;
background-color: #f5f5f5;
border: 1px solid #ccc;
border-radius: 5px;
}
</style>
</head>
<body>
<nav class="navbar">
<div class="logo-container">
<img class="logo" src=
"https://media.geeksforgeeks.org/gfg-gg-logo.svg" alt="Logo"/>
</div>
</nav>
<div id="content">
<h1>Using jQuery One-Time Event Binding to prevent
duplicate form submissions
</h1>
<form id="myForm">
<input type="text" name="username"
placeholder="Username" required>
<input type="password" name="password"
placeholder="Password" required>
<button type="submit">Submit</button>
</form>
<div id="successMessage">
Form submitted successfully!
</div>
<div id="submittedData"></div>
</div>
<script src=
"https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js">
</script>
<script>
$(document).ready(function () {
$('#myForm').one('submit', function (event) {
event.preventDefault();
// Display success message
$('#successMessage').show();
// Show submitted data
let formData = $(this).serialize();
$('#submittedData').html(
'<strong>Submitted Data:</strong><br>'
+ formData).show();
// Disabling form fields and button
$(this).find('input, button')
.prop('disabled', true);
});
});
</script>
</body>
</html>
Output:
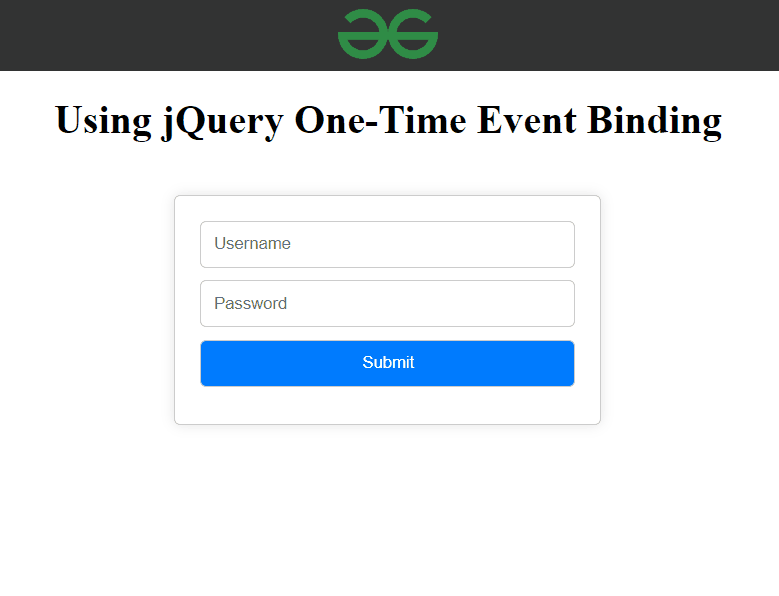
Output
This approach involves using jQuery, to disable the submit button immediately upon form submission. By disabling the button, users are unable to click it multiple times, effectively preventing duplicate form submissions, enhancing user experience and preventing unintended actions.
Example: Implementation of preventing duplicate submission in a form by disabling the submit button.
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport"
content="width=device-width,
initial-scale=1.0">
<title>Prevent Duplicate Form Submission</title>
<style>
.navbar {
background-color: #333;
padding: 10px 0;
}
.logo-container {
display: flex;
justify-content: center;
align-items: center;
}
.logo {
width: 80px;
height: auto;
}
</style>
<script src="https://code.jquery.com/jquery-3.6.0.min.js">
</script>
<script>
$(document).ready(function () {
$('#myForm').submit(function (event) {
// Prevent default form submission
event.preventDefault();
// Check if the form has already been submitted
if ($(this).data('submitted')) {
return;
}
// Disable the submit button
$('#submitBtn').prop('disabled', true);
let formData = $(this).serialize();
$('#submittedData').html(formData);
$('#statusMessage').text('Submitting...');
$(this).data('submitted', true);
setTimeout(function () {
$('#statusMessage').text('');
}, 3000);
});
});
</script>
</head>
<body>
<nav class="navbar">
<div class="logo-container">
<img alt="Logo" class="logo" src=
"https://media.geeksforgeeks.org/gfg-gg-logo.svg" />
</div>
</nav><br />
<h2>Prevent duplicate submission
in a form using jQuery
</h2>
<form id="myForm">
<input type="text" name="name"
placeholder="Name" required><br>
<input type="email" name="email"
placeholder="Email" required><br>
<input type="submit" id="submitBtn"
value="Submit">
</form>
<p id="statusMessage"></p>
<div id="submittedData">
</div>
</body>
</html>
Output:
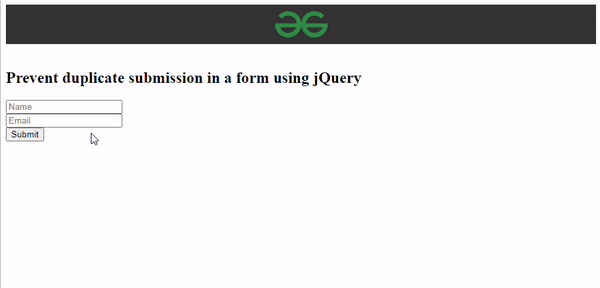
Share your thoughts in the comments
Please Login to comment...