How to Add External JS Scripts to VueJS Components?
Last Updated :
07 May, 2024
VueJS is a popular front-end JavaScript framework that allows us to develop single-page web applications with the help of components. We can also add external JS scripts in the framework to integrate any 3rd party web services into our application.
Below are the approaches to add external JS scripts to VueJS components:
Steps to create the VueJS app:
Step 1: Create an app
We will create a vue app with the below commands. We will then use this app to use video.js library.
vue create sample-vue
This will create a sample vue application with the below project structure.
Step 2: Go to the folder
cd sample-vue
Project structure:
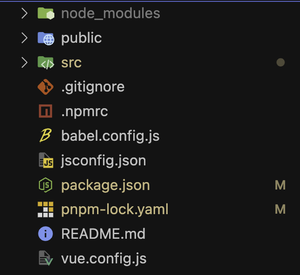
Using VueJS lifecycle hooks
In this approach, we will use the VueJS lifecycle hooks to create a script HTML node, and then insert that script node into the document itself.
Example: In this example, we will insert the script in the mounted lifecycle hook of VueJS.
HTML
//App.vue
<template>
<div>
<h1>Hello Vue.js!</h1>
</div>
</template>
<script>
export default {
mounted() {
const script = document.createElement("script");
script.src =
"https://cdn.jsdelivr.net/npm/hello-world-js@0.0.2/src/index.min.js";
document.body.appendChild(script);
},
};
</script>
Steps to run the server:
Now, we will run our web application by running the below command –
pnpm serve
Output:
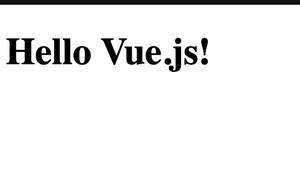
Import in a specific Vue component
In this approach, we will install the NPM package corresponding the script, and then use that package in our components. we will use the installed package in the App.vue file, and just call the method “helloWorld”, which is provided the package itself, when the component gets mounted.
pnpm install hello-world-npm
Example: In this example, we will install an NPM package, and then import that package to use it in the component.
JavaScript
<template>
<div>
<h1>Hello Vue.js!</h1>
</div>
</template>
<script>
import { helloWorld } from "hello-world-npm";
export default {
mounted() {
console.log(helloWorld());
},
};
</script>
Steps to run the server:
Now, we will run our web application by running the below command –
pnpm serve
Output:
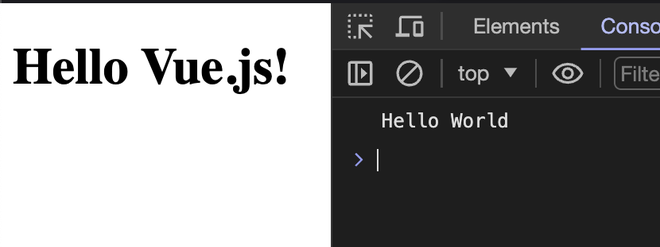
Share your thoughts in the comments
Please Login to comment...