How to Add List Items Onclick in VueJS ?
Last Updated :
12 Apr, 2024
In VueJS, we can make dynamic applications by adding list items on click. This allows for interactive user experiences where new items can be added to a list with a click event.
The below approaches can be used to accomplish this task.
By creating a custom method to Add Items
In this approach, we are using Vue.js methods to handle the onclick event of a button. The addItem method adds new items to the list by pushing objects with unique IDs and text values into the items array, updating the list dynamically without a page refresh.
Example: The below example uses a Method to Add Items to Add List Items Onclick in Vue JS.
HTML
<!DOCTYPE html>
<html>
<head>
<title>Example 1</title>
<script src=
"https://cdn.jsdelivr.net/npm/vue@2/dist/vue.js">
</script>
</head>
<body>
<div id="app">
<h1>
Using a Method to Add Items
</h1>
<h2>List Items</h2>
<ul>
<li v-for="item in items" :key="item.id">
{{ item.text }}
</li>
</ul>
<button @click="addItem">
Add Item
</button>
</div>
<script>
new Vue({
el: '#app',
data: {
items: [
{ id: 1, text: 'Item 1' },
{ id: 2, text: 'Item 2' },
{ id: 3, text: 'Item 3' }
],
nextItemId: 3
},
methods: {
addItem() {
this.items.push({
id: this.nextItemId++,
text: `Item ${this.nextItemId}`
});
}
}
});
</script>
</body>
</html>
Output:
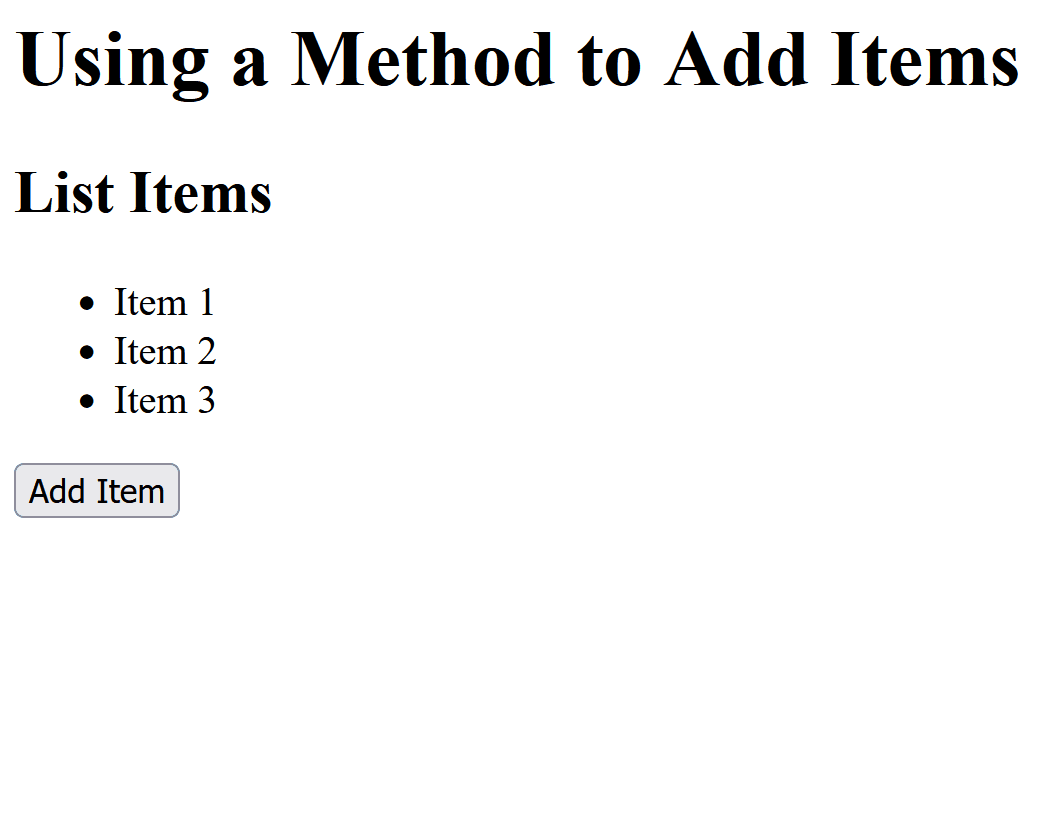
In this approach, we are using a computed property addItem that returns a function to handle adding items to the list when the button is clicked. The computed property is bound to the input field using v-model, enabling two-way data binding.
Syntax:
<input v-model="computedProperty">
Example: The below example uses a computed Property with v-model to Add Items to Add List Items Onclick in Vue JS
HTML
<!DOCTYPE html>
<html>
<head>
<title>Example 2</title>
<script src=
"https://cdn.jsdelivr.net/npm/vue@2/dist/vue.js">
</script>
</head>
<body>
<div id="app">
<h1>
Using computed Property with v-model
</h1>
<h2>List Items</h2>
<ul>
<li v-for="item in items" :key="item.id">
{{ item.text }}
</li>
</ul>
<input v-model="newItemText">
<button @click="addItem">
Add Item
</button>
</div>
<script>
new Vue({
el: '#app',
data: {
items: [
{ id: 1, text: 'Item 1' },
{ id: 2, text: 'Item 2' },
{ id: 3, text: 'Item 3' }
],
nextItemId: 4,
newItemText: ''
},
computed: {
addItem() {
return () => {
if (this.newItemText.trim() !== '') {
this.items.push({
id: this.nextItemId++,
text: this.newItemText
});
this.newItemText = '';
}
};
}
}
});
</script>
</body>
</html>
Output:
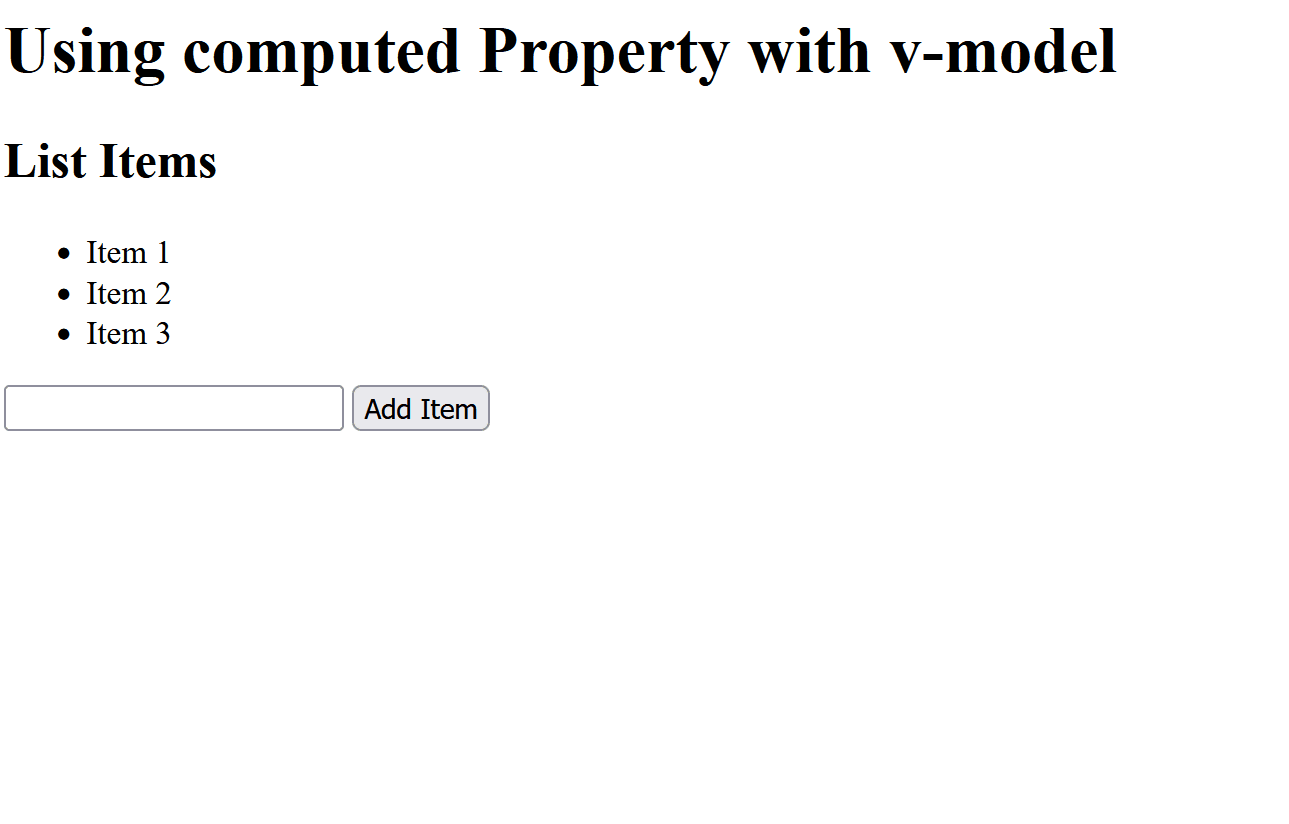
Share your thoughts in the comments
Please Login to comment...