How to Add or Apply Global Variables in VueJS ?
Last Updated :
09 Jan, 2024
In Vue.js, we can have global variables that are applied by attaching them to the Vue instance prototype. These are accessible throughout the components of the application. This is helpful for data management and sharing. In this article, we will see the two different approaches to adding or applying global variables in VueJS with practical examples.
Approach 1: Using Vue Prototype
In this approach, we will use the Vue.prototye.$gVar to define the global variable that is accessed in the entire application. There is an input box and button through which the updation and application of the global variable will be managed.
Syntax:
Vue.prototype.$globalVariable = 'initialValue';
Example: Below is the implementation of the above-discussed approach.
HTML
<!DOCTYPE html>
< html >
< head >
< title >Example 1</ title >
< script src =
</ script >
< style >
body {
font-family: 'Arial',
sans-serif;
text-align: center;
margin: 50px;
}
input {
padding: 10px;
margin-bottom: 20px;
}
button {
padding: 10px 20px;
background-color: #4CAF50;
color: white;
border: none;
border-radius: 5px;
cursor: pointer;
}
</ style >
</ head >
< body >
< h1 style = "color: green;" >
GeeksforGeeks
</ h1 >
< h3 >
Approach 1: Using
Vue.prototype
</ h3 >
< div id = "app" >
< h2 >{{ message }}</ h2 >
< input v-model = "gVar"
placeholder="Enter a
global variable">
< button @ click = "updateGVar" >
Apply Global Variable
</ button >
</ div >
< script >
Vue.prototype.$gVar = '';
new Vue({
el: '#app',
data: {
message:
'This is Global Variable ',
gVar: ''
},
methods: {
updateGVar() {
this.$gVar = this.gVar;
this.message =
`Global Variable Updated:
${this.$gVar}`;
}
}
});
</ script >
</ body >
</ html >
|
Output:
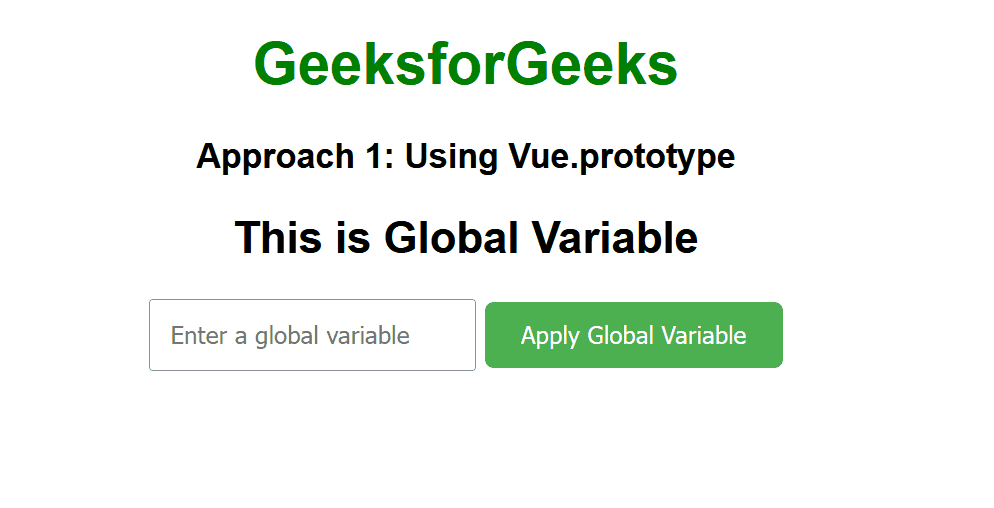
Approach 2: Using Vue.mixin
In this approach, we are defining the mixin with the data object containing the global variable ‘globalVar‘ and the methods to update and rest it. By applying the mixin global to the Vue Instance, we can access and modify the shared global variable in the application.
Syntax:
Vue.mixin({
data() {
return {
globalVariable: {
// global variable properties
}
};
},
});
Example: Below is the implementation of the above-discussed approach.
HTML
<!DOCTYPE html>
< html >
< head >
< title > Example 2</ title >
< style >
body {
font-family: 'Arial', sans-serif;
text-align: center;
}
button {
padding: 10px 20px;
font-size: 16px;
cursor: pointer;
background-color: #4CAF50;
color: white;
border: none;
border-radius: 5px;
margin-top: 10px;
}
</ style >
</ head >
< body >
< div id = "app" >
< h1 style = "color: green;" >
GeeksforGeeks
</ h1 >
< h3 >
Approach 2: Using Vue.mixin
</ h3 >
< h4 >{{ message }}</ h4 >
< label for = "newMsg" >
Change Global Variable:
</ label >
< input type = "text"
id = "newMsg"
v-model = "newMsg" >
< button @ click = "updateGVar" >
Update Global Variable
</ button >
< button @ click = "resetGVar" >
Reset Global Variable
</ button >
</ div >
< script src =
</ script >
< script >
Vue.mixin({
data() {
return {
globalVar: {
message:
'Hello, GFG!'
},
newMsg: ''
};
},
computed: {
message() {
return this.
globalVar.message;
}
},
methods: {
updateGVar() {
if (this.newMsg.trim() !== '') {
this.globalVar.message =
this.newMsg;
this.newMsg = '';
}
},
resetGVar() {
this.globalVar.message =
'Hello, Global Variable!';
}
}
});
new Vue({
el: '#app',
});
</ script >
</ body >
</ html >
|
Output:
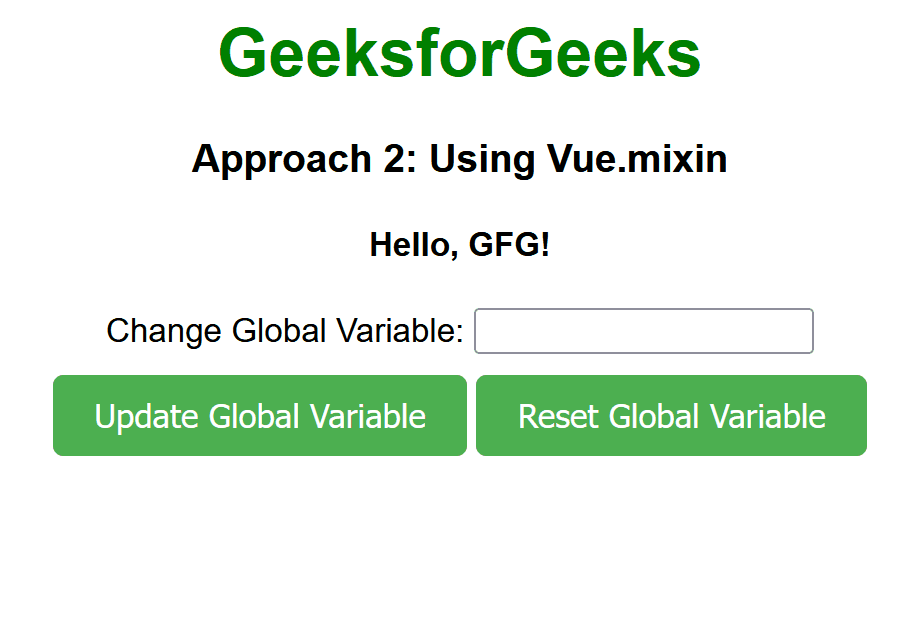
Share your thoughts in the comments
Please Login to comment...