How to Add a Subject to a Mailto link in HTML ?
Last Updated :
12 Mar, 2024
In HTML, to add a subject to a Mailto link, we can use the Subject as a query parameter just after the specified email address and pass the subject of the email as its value. Along with this, we can use JavaScript to add custom subjects according to the user input.
Directly adding the subject to the email
In this approach, we will use the mailto link with the subject parameter directly embedded in the HTML. The provided email link, when clicked, opens the user’s default email client with the specified subject line.
Syntax:
<a href="mailto:emailAddress?subject=subjectContent">
Send Email with Subject
</a>
Example: The below example uses the subject parameter directly inside the mailto link to add a Subject to it.
HTML<!DOCTYPE html>
<html>
<head>
<title>Example 1</title>
<style>
body {
font-family: 'Arial', sans-serif;
background-color: #f4f4f4;
margin: 0;
padding: 20px;
box-sizing: border-box;
text-align: center;
}
.email-link {
color: #3498db;
text-decoration: none;
font-weight: bold;
padding: 10px 20px;
border: 2px solid #3498db;
border-radius: 5px;
display: inline-block;
margin-top: 20px;
transition: background-color 0.3s;
}
.email-link:hover {
background-color: #5bdb34;
color: #fff;
}
</style>
</head>
<body>
<h1 style="color: green;">
GeeksforGeeks
</h1>
<h3>
Using subject Header Only
</h3>
<a class="email-link" href=
"mailto:review-team@geeksforgeeks.org?subject=Hello GeeksforGeeks">
Send Email with Subject
</a>
</body>
</html>
Output:
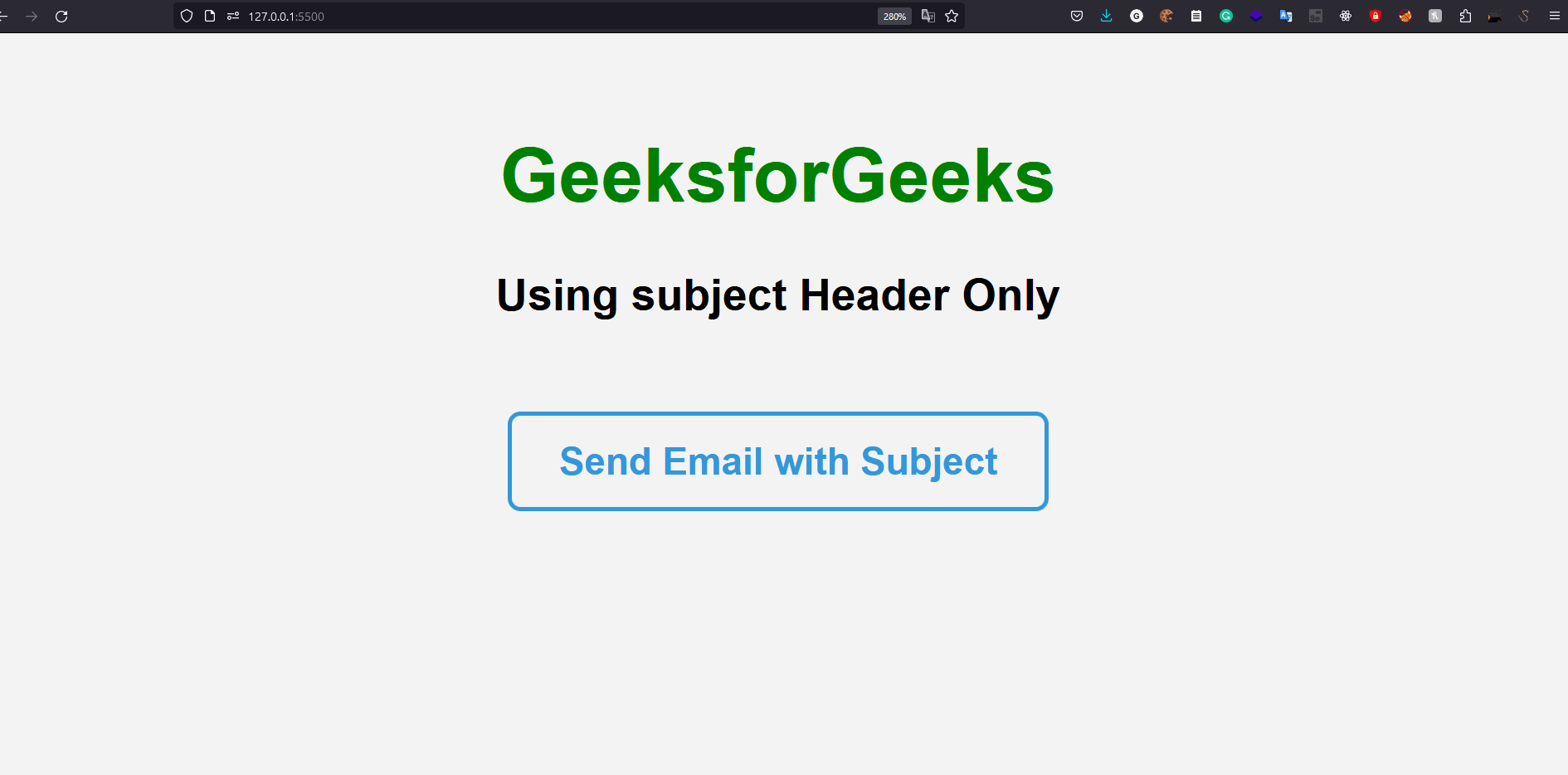
Adding subject using JavaScript
In this approach, we use the mailto link with JavaScript to dynamically set the subject based on user input. The provided HTML includes an input field for users to enter a custom subject, and the JavaScript function to append it to the email link.
Syntax:
let link = "mailto:emailAddress?subject="
+ encodeURIComponent(sub);
Example: The below example uses JavaScript to add a subject to a mailto link in HTML.
HTML<!DOCTYPE html>
<html>
<head>
<title>Example 2</title>
<style>
body {
font-family: 'Arial', sans-serif;
background-color: #f4f4f4;
margin: 0;
padding: 20px;
box-sizing: border-box;
text-align: center;
}
.email-link {
color: #3498db;
text-decoration: none;
font-weight: bold;
padding: 10px 20px;
border: 2px solid #3498db;
border-radius: 5px;
display: inline-block;
margin-top: 20px;
transition: background-color 0.3s;
}
.email-link:hover {
background-color: #3498db;
color: #fff;
}
.custom-subject-input {
padding: 8px;
margin-top: 10px;
border: 1px solid #ccc;
border-radius: 5px;
}
</style>
</head>
<body>
<h1 style="color: green;">
GeeksforGeeks
</h1>
<h3>
Using subject Header with JavaScript
</h3>
<label for="customSubject">
Enter Subject:
</label>
<input type="text" id="customSubject"
class="custom-subject-input"
placeholder="Enter the subject">
<br />
<a href="#" onclick="mailFn()" class="email-link">
Send Email
</a>
<script>
function mailFn() {
let sub =
document.getElementById('customSubject').value;
let link =
"mailto:review-team@geeksforgeeks.org?subject="
+ encodeURIComponent(sub);
window.location.href = link;
}
</script>
</body>
</html>
Output:
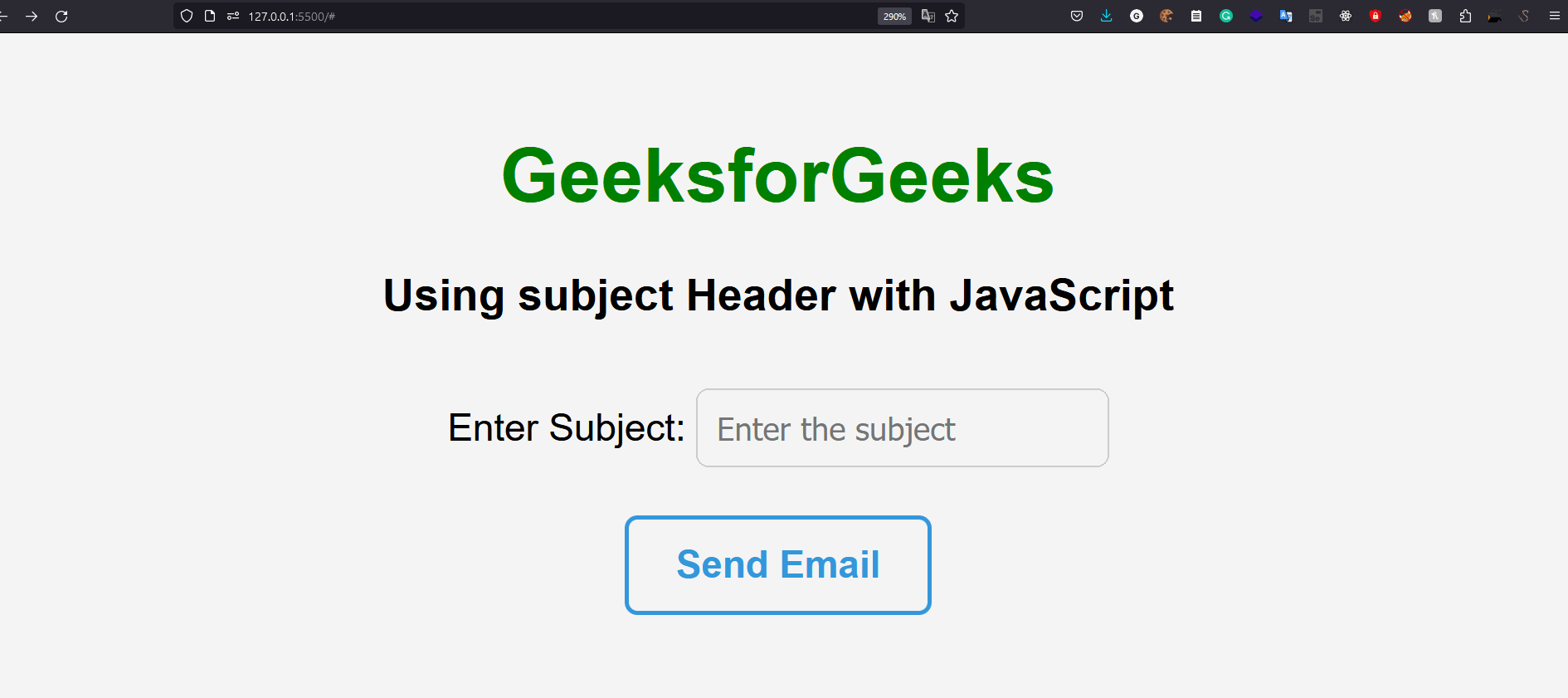
Share your thoughts in the comments
Please Login to comment...