How Does Solidity Works?
Last Updated :
07 Aug, 2023
Solidity is a programming language that helps developers create special programs called smart contracts. These contracts are used on the Ethereum blockchain, Which is like a giant computer that runs Decentralized Applications (DApps). In this article, We will explain how Solidity works in a simple way, using Examples to make it easier to understand.
What is Solidity?
Solidity is a programming language that looks similar to JavaScript, and it’s used only for making smart contracts.
- Smart contracts are like computer programs that run on the Ethereum Network. They work automatically and do specific things when people meet certain conditions.
- Solidity is built to work well with Ethereum, and it supports many useful features like object-oriented programming and events.
- The file extension for Solidity is .sol.
- Solidity provides several built-in types, including address, uint (unsigned integer), int (signed integer), bool (boolean), and byte (a fixed-size byte array).
- It allows for creating user-defined types, such as enums and structs.
Smart Contract
Imagine we want to create a contract that says “Hello” and allows people to change the greeting message. Below is the Solidity program to implement the above approach:
Solidity
pragma solidity ^0.8.0;
contract helloGeeks {
string public greeting;
constructor()
{
greeting = "Hello, World!" ;
}
function setGreeting(string memory _greeting) public
{
greeting = _greeting;
}
function getGreeting() public view returns (string memory)
{
return greeting;
}
}
|
Output:
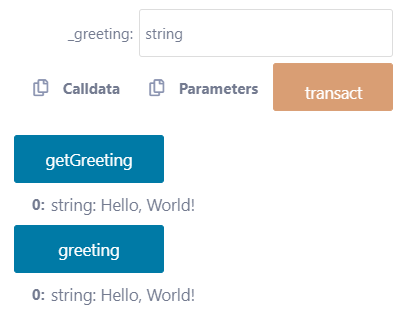
Explanation:
In this code, we define a contract called helloGeeks. It has a variable called greeting that stores the message. We also have two functions: setGreeting to change the message and getGreeting to read the current message.
How Does Solidity Work?
1. Public and Private Functions
In Solidity, functions are the building blocks of smart contracts. Some functions can be accessed from outside the contract (public), while others are only available within the contract (private).
Solidity
pragma solidity ^0.8.0;
contract VotingContract {
mapping(address => bool ) public hasVoted;
uint256 public totalVotes;
function vote() public {
require(!hasVoted[msg.sender], "You have already voted." );
hasVoted[msg.sender] = true ;
totalVotes++;
}
}
|
Output: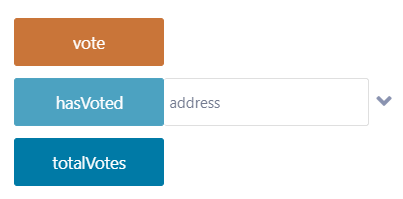
In this example, the vote function is public, allowing anyone to vote. It checks if the sender has voted before and increments the totalVotes counter.
2. Standard and Code Logic
Solidity follows a set of rules and logic similar to other programming languages. The behavior of a smart contract is determined by the functions and conditions defined in the code.
Solidity
pragma solidity ^0.8.0;
contract LotteryContract {
address public manager;
address[] public players;
constructor() {
manager = msg.sender;
}
function enter() public payable {
require(msg.value > 0.01 ether, "Minimum 0.01 ether required to enter." );
players.push(msg.sender);
}
function random() private view returns (uint256) {
return uint256(keccak256(abi.encodePacked(block.difficulty, block.timestamp, players)));
}
function pickWinner() public restricted {
uint256 index = random() % players.length;
address winner = players[index];
payable(winner).transfer(address( this ).balance);
players = new address[](0);
}
modifier restricted() {
require(msg.sender == manager, "Only the manager can call this function." );
_;
}
}
|
Output:
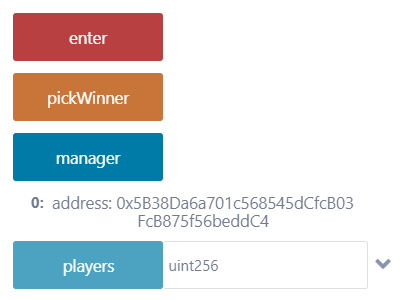
Explanation:
In this example, we have a Lottery Contract. Players can enter the lottery by sending more than 0.01 ether. The pickWinner function randomly selects a winner and transfers the contract’s balance to the winner’s address. The restricted modifier ensures that only the contract manager can call the pickWinner function.
3. Immutability
Once deployed on the Ethereum blockchain, a smart contract becomes immutable, meaning its code and behavior cannot be changed. This ensures the contract’s transparency and security, as participants can trust that the contract will always function as intended.
4. Gas Costs
When interacting with smart contracts on Ethereum, users must pay for the computational power used during the execution of functions. This payment is done using “gas.” Each operation within the contract consumes a specific amount of gas and users need to provide enough gas to complete the transaction. This system incentivizes developers to write efficient code and prevents malicious activities from overloading the Ethereum network.
5. Data Types
Solidity has different types of data that can be used, such as numbers, addresses, true/false values, text, arrays, and more.
Solidity
pragma solidity ^0.8.0;
contract DataTypesExample {
uint256 public myNumber;
address public myAddress;
bool public myBool;
string public myString;
uint256[] public myArray;
constructor() {
myNumber = 42;
myAddress = 0x5B38Da6a701c568545dCfcB03FcB875f56beddC4;
myBool = true ;
myString = "Hello, Solidity!" ;
myArray.push(1);
myArray.push(2);
}
}
|
Output:
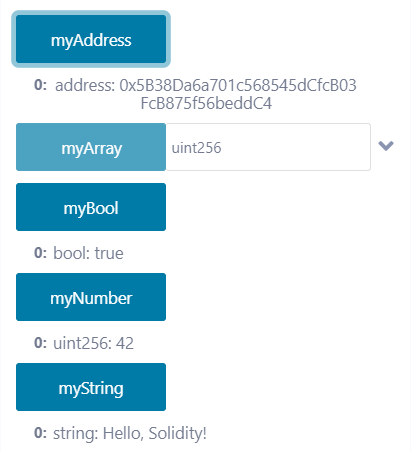
Explanation:
In this code, we define a contract called DataTypesExample that shows different data types. We have a number (myNumber), an address (myAddress), a true/false value (myBool), a text message (myString), and an array of numbers (myArray).
6. Control Structures
Solidity provides tools to control how the program behaves, such as conditional statements (if-else), loops (for, while), and switches.
Solidity
pragma solidity ^0.8.0;
contract ControlStructuresExample {
uint256 public number;
function checkEven(uint256 _number) public {
if (_number % 2 == 0) {
number = _number;
} else {
number = 0;
}
}
function sumRange(uint256 _limit) public {
uint256 sum = 0;
for (uint256 i = 1; i <= _limit; i++) {
sum += i;
}
number = sum;
}
}
|
Output:
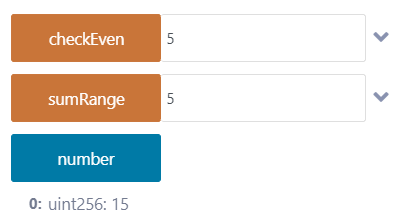
Explanation:
In this code, we define a contract called ControlStructuresExample. It has two functions: checkEven checks if a number is even and assigns it to the number variable if it is, otherwise sets number to 0. The sumRange function calculates the sum of numbers from 1 to a given limit using a loop.
7. Modifiers
Modifiers are like rules that we can apply to functions. They allow us to add extra checks or behaviors to functions.
Solidity
pragma solidity ^0.8.0;
contract ModifiersExample {
address public owner;
modifier onlyOwner() {
require(msg.sender == owner, "Only the contract owner can call this function." );
_;
}
constructor() {
owner = msg.sender;
}
function changeOwner(address _newOwner) public onlyOwner {
owner = _newOwner;
}
}
|
Output:
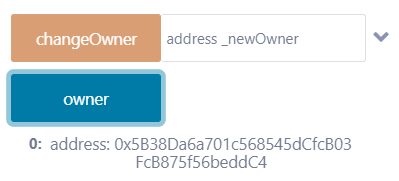
Explanation:
In this code, we define a contract called ModifiersExample. It has a modifier called onlyOwner that checks if the caller of a function is the contract owner. If not, it throws an error. The changeOwner function can only be called by the owner because of the onlyOwner modifier.
8. Events
Events allow contracts to communicate with external applications. They are like messages that can be logged on the blockchain and listened to by other programs.
Solidity
pragma solidity ^0.8.0;
contract EventsExample {
event NumberSet(uint256 number);
function setNumber(uint256 _number) public {
emit NumberSet(_number);
}
}
|
Output:

Explanation:
In this code, we define a contract called EventsExample. It has an event called NumberSet that is emitted when the setNumber function is called. Other programs can listen to this event and react accordingly.
Share your thoughts in the comments
Please Login to comment...