Solidity – Variables
Last Updated :
11 May, 2022
A Variable is basically a placeholder for the data which can be manipulated at runtime. Variables allow users to retrieve and change the stored information.
Rules For Naming Variables
1. A variable name should not match with reserved keywords.
2. Variable names must start with a letter or an underscore (_), and may contain letters from “a to z” or “A to Z” or digits from “0 to 9” and characters also.
Example:
Geeks123, geeks, _123geeks are valid variable names
123geeks, $Geeks, 12_geeks are invalid variable names
3. The name of variables are case sensitive i.e.
Example:
Geeks123 and geeks123 are different variables
Declaration of Variables
In Solidity declaration of variables is a little bit different, to declare a variable the user has to specify the data type first followed by access modifier.
Syntax:
<type> <access modifier> <variable name> ;
Example:
int public int_var;
Types of Variables
Solidity is a statically typed language i.e. each declared variable always has a default value based on its data type, which means there is no concept of ‘null’ or ‘undefined’. Solidity supports three types of variables:
1. State Variables: Values of these variables are permanently stored in the contract storage. Each function has its own scope, and state variables should always be defined outside of that scope.
Example: In the below example. the contract Solidity_var_Test initializes the values of an unsigned integer state variable using a constructor.
Solidity
pragma solidity ^0.5.0;
contract Solidity_var_Test {
uint8 public state_var;
constructor() public {
state_var = 16;
}
}
|
Output :
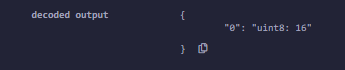
2. Local Variable: Values of these variables are present till the function executes and it cannot be accessed outside that function. This type of variable is usually used to store temporary values.
Example: In the below example, the contract Solidity_var_Test defines a function to declare and initialize the local variables and return the sum of the two local variables.
Solidity
pragma solidity ^0.5.0;
contract Solidity_var_Test {
function getResult() public view returns(uint){
uint local_var1 = 1;
uint local_var2 = 2;
uint result = local_var1 + local_var2;
return result;
}
}
|
Output :

3. Global Variables: These are some special variables that can be used globally and give information about the transactions and blockChain properties. Some of the global variables are listed below :
Variable |
Return value |
blockhash(uint blockNumber) returns (bytes32) |
Hash of a given block, works for only 256 most recent transactions excluding current blocks |
block.coinbase (address payable) |
Address of current blocks miner |
block.difficulty (uint) |
The difficulty of the current block |
block.gaslimit (uint) |
Gaslimit of the current block |
block.number (uint) |
Block number of the current block |
block.timestamp (uint) |
The timestamp of the current block as seconds since Unix epoch |
gasleft() returns (uint256) |
Amount of gas left |
msg.data (bytes calldata) |
Complete call data of block |
msg.sender (address payable) |
The sender of message i.e. current caller |
msg.sig (bytes4) |
First four bytes of call data i.e. function identifier |
msg.value (uint) |
Amount of Wei sent with a message |
now (uint) |
The timestamp of the current block |
gasleft() returns (uint256) |
Amount of gas left |
tx.gasprice (uint) |
Price of gas for the transaction |
tx.origin (address payable) |
Transaction sender |
Example: In the below example, the contact Test uses the msg.sender variable to access the address of the person deploying the contract.
Solidity
pragma solidity ^0.5.0;
contract Test {
address public admin;
constructor() public {
admin = msg.sender;
}
}
|
Output:
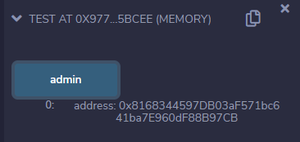
Share your thoughts in the comments
Please Login to comment...