Type Conversion in Solidity
Last Updated :
09 Aug, 2023
Solidity is a popular programming language specifically designed for developing smart contracts on the Ethereum blockchain. As a statically-typed language, Solidity offers various features to handle data types and conversions. Conversions play a crucial role in transforming data from one type to another, allowing developers to manipulate and interact with variables efficiently. In this article, we will explore the concept of conversions in Solidity and how they can be utilized effectively.
Solidity provides several built-in functions and operators for type conversions, enabling developers to convert between different data types such as integers, strings, addresses, and more. These conversions are essential for performing arithmetic operations, input/output operations, and interacting with external contracts and libraries.
Type Conversions
Let’s dive into some common types of conversions and how they can be used in Solidity:
1. Integer Conversions
Integer conversions in Solidity involve converting values between different integer types, such as uint8 to uint256, to accommodate varying ranges and precision requirements. This allows for efficient storage and manipulation of integers while ensuring data integrity.
Example:
Solidity
pragma solidity ^0.8.0;
contract helloGeeks {
function convert() public pure returns (uint256) {
uint8 a = 100;
uint256 b = uint256(a);
return b;
}
}
|
Output:
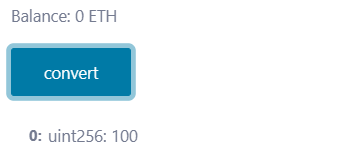
Explanation:
In the above example, the code defines a Solidity contract named helloGeeks with a public function convert that returns a uint256 value.
- Inside the function, a uint8 variable a is assigned the value 100. To convert a to a uint256, the type-casting syntax uint256(a) is used, and the result is stored in a uint256 variable b.
- Finally, the function returns the converted b.
- This integer conversion allows storing a smaller-sized integer (uint8) in a larger-sized integer (uint256) for a wider range and precision without data loss.
2. String Conversions
String conversions in Solidity involve converting non-string data types, such as integers or addresses, to their string representations. This enables the manipulation and display of data in a human-readable format, facilitating communication and data presentation in smart contracts.
Example:
Solidity
pragma solidity ^0.8.0;
contract helloGeeks {
function convertToString() public pure returns (string memory) {
uint256 x = 42;
string memory str = toString(x);
return str;
}
function toString(uint256 value) internal pure returns (string memory) {
if (value == 0) {
return "0" ;
}
uint256 temp = value;
uint256 digits;
while (temp != 0) {
digits++;
temp /= 10;
}
bytes memory buffer = new bytes(digits);
while (value != 0) {
digits--;
buffer[digits] = bytes1(uint8(48 + (value % 10)));
value /= 10;
}
return string(buffer);
}
}
|
Output:
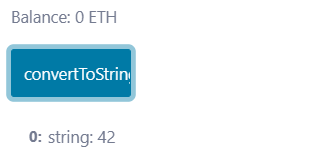
Explanation:
In the above example, the contract helloGeeks has two functions.
- The convertToString function returns a string value. It assigns the value 42 to a variable x of type uint256. The toString function is called with x as an argument to convert it to a string, and the result is stored in str. The toString function takes a uint256 input and returns a string. If the input is zero, it directly returns “0”.
- Otherwise, it calculates the number of digits by dividing the input by 10 until it reaches zero, incrementing a counter. It creates a buffer of bytes with a length equal to the number of digits.
- Then, it extracts the individual digits from the input, starting from the most significant digit, converts them to ASCII representation, and stores them in the buffer.
- Finally, the buffer is converted back to a string, and the string representation of the original value is returned.
3. Address Conversions
In Solidity, addresses can be converted between different types, such as converting an address to a uint or a string. Converting an address to a uint can be useful when performing arithmetic operations while converting it to a string can be beneficial for output formatting or concatenation.
Example:
Solidity
pragma solidity ^0.8.0;
contract helloGeeks {
function convert() public pure returns (string memory) {
address addr = address(0xec6758926Df05d19ea1EFebf0731EFe381Cd6B07);
string memory addrStr = toString(addr);
return addrStr;
}
function toString(address _addr) internal pure returns (string memory) {
bytes32 value = bytes32(uint256(uint160(_addr)));
bytes memory alphabet = "0123456789abcdef" ;
bytes memory str = new bytes(42);
str[0] = '0' ;
str[1] = 'x' ;
for (uint256 i = 0; i < 20; i++) {
str[2 + i * 2] = alphabet[uint8(value[i + 12] >> 4)];
str[3 + i * 2] = alphabet[uint8(value[i + 12] & 0x0f)];
}
return string(str);
}
}
|
Output:
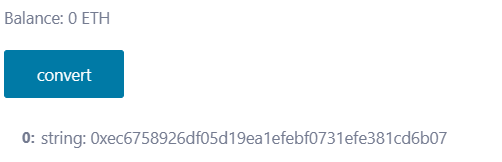
Explanation:
In the above example, the helloGeeks contract has two functions.
- The convert function returns a string. It assigns an Ethereum address to an address variable named addr.
- To convert the address to a string, the code calls the toString function with addr as an argument.
- The toString function takes an address input and returns a string. It converts the address to a bytes32 value, creates a bytes array to store the resulting string, adds the 0x prefix, extracts the hexadecimal characters from the bytes32 value, and converts the bytes array to a string.
4. Type Conversions for External Interactions
Type conversions for external interactions in Solidity, such as using the payable keyword, allow for seamless interaction with external contracts, enabling transfers of Ether and access to specific functionalities exclusive to payable addresses. It ensures compatibility and enables smooth integration with other contracts or external entities in the Ethereum ecosystem.
Example:
Solidity
pragma solidity ^0.8.0;
contract helloGeeks {
function convert() public pure returns (address payable) {
address addr = 0xec6758926Df05d19ea1EFebf0731EFe381Cd6B07;
address payable addrPayable = payable(addr);
return addrPayable;
}
}
|
Output:
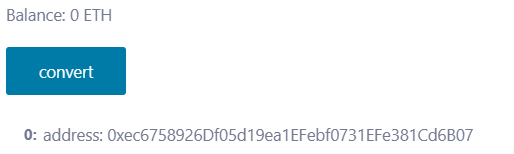
Explanation:
In the above example, the helloGeeks contract has a convert function that returns an address payable.
- Inside the function, an Ethereum address is assigned to an address variable named addr.
- The code uses the payable keyword to convert addr to an address payable variable called addrPayable. This conversion allows for interactions with external contracts and transfers to addresses.
- The function then returns addrPayable, the converted address in its payable form.
Share your thoughts in the comments
Please Login to comment...