Address in Solidity
Last Updated :
07 Aug, 2023
In Solidity, the address type represents Ethereum addresses. Understanding how to work with addresses is essential for developing smart contracts that interact with users and other contracts on the Ethereum blockchain. This article aims to provide a comprehensive guide to addresses in Solidity, accompanied by relevant examples and covering various subtopics.
Basics of Addresses and Address Literals
The address type in Solidity is a 20-byte value that represents an Ethereum address. It can hold the address of a user or a contract on the Ethereum network. Here’s an example of declaring and using an address variable in Solidity:
Solidity
pragma solidity ^0.8.0;
contract helloGeeks {
address public userAddress;
function setAddress(address _newAddress) public {
userAddress = _newAddress;
}
}
|
Output
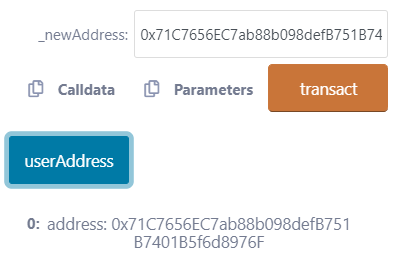
In this example, the userAddress variable of type address is declared as a public variable. The setAddress function takes an address parameter and assigns its value to the userAddress variable.
Additionally, Solidity allows using address literals to specify addresses directly in the code. Here’s an example:
Solidity
pragma solidity ^0.8.0;
contract helloGeeks {
address public userAddress = 0x71C7656EC7ab88b098defB751B7401B5f6d8976F;
}
|
Output
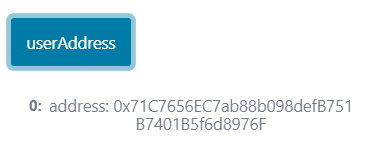
Address vs Address Payable
In Solidity, there are two address types: address and address payable. The address type is used for both user addresses and contract addresses, while the address payable type is specifically used for addresses that can receive Ether. Here’s an example:
Solidity
pragma solidity ^0.8.0;
contract helloGeeks {
address public userAddress;
address payable public recipientAddress;
function setAddress(address _userAddress, address payable _recipientAddress) public {
userAddress = _userAddress;
recipientAddress = _recipientAddress;
}
}
|
Output
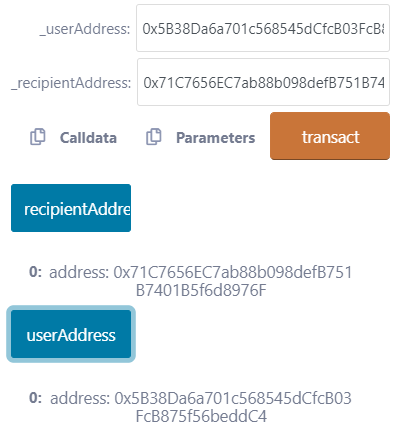
In this example, the userAddress variable is of type address, while the recipientAddress variable is of type address payable This difference allows the recipientAddress address to receive Ether.
Methods Available with Addresses
Solidity provides several methods that can be used with addresses to perform various operations. These methods include call(), delegatecall(), and staticcall().
1. call()
The call() method allows the executing code of another contract and transferring Ether in a single function call. Here’s an example:
Solidity
pragma solidity ^0.8.0;
contract helloGeeks {
address public recipientAddress;
function sendEther() public payable {
( bool success, ) = recipientAddress.call{value: msg.value}( "" );
require(success, "Transfer failed." );
}
}
|
Output
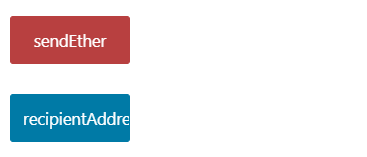
.png)
Transaction
In this example, the sendEther function transfers the amount of Ether sent with the transaction to the recipientAddress address using the call() method.
2. delegatecall()
The delegatecall() method is similar to call(), but it preserves the context of the calling contract. It allows executing code from another contract while maintaining the calling contract’s storage and state. Here’s an example:
Solidity
pragma solidity ^0.8.0;
contract helloGeeks {
address public targetAddress;
function executeDelegateCall(
address _targetAddress,
bytes memory _data
) public returns ( bool , bytes memory) {
( bool success, bytes memory result) = _targetAddress.delegatecall(_data);
return (success, result);
}
}
|
Output
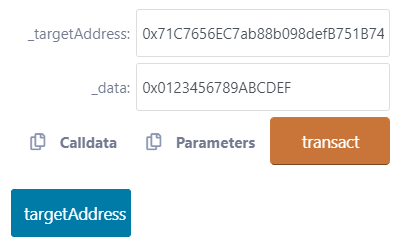
In this example, the executeDelegateCall function performs a delegate call to the contract specified by the _targetAddress address, passing the _data parameter. It returns the success status and the result of the delegate call.
The staticcall() method allows executing the code of another contract without modifying the calling contract’s state. It is primarily used for reading data from other contracts. Here’s an example:
Solidity
pragma solidity ^0.8.0;
contract helloGeeks {
address public targetAddress;
function readData(
address _targetAddress,
bytes memory _data
) public view returns (bytes memory) {
( bool success, bytes memory result) = _targetAddress.staticcall(_data);
require(success, "Static call failed." );
return result;
}
}
|
Output
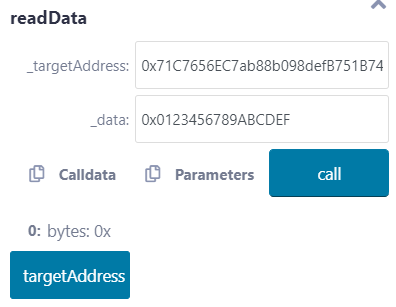
In this example, the readData function performs a static call to the contract specified by the _targetAddress address, passing the _data parameter. It returns the result of the static call.
Type Conversions between Addresses and Address Payable
Solidity supports type conversions between address and address payable types. Here’s an example:
Solidity
pragma solidity ^0.8.0;
contract helloGeeks {
address public userAddress;
address payable public recipientAddress;
function convertAddress(address _userAddress) public {
recipientAddress = payable(_userAddress);
}
}
|
Output
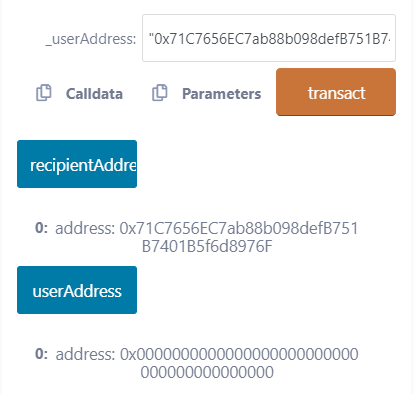
In this example, we have a helloGeeks contract that includes two address variables: userAddress and recipientAddress. The convertAddress function takes an address parameter _userAddress and converts it explicitly to an address payable using payable(_userAddress). The resulting address payable is then assigned to the recipientAddress variable. This conversion allows the contract to handle address payable functionality and perform operations like sending Ether.
Methods Returning an Address Type
Methods returning an address type in Solidity allow functions to provide Ethereum addresses as outputs. For instance, consider the Solidity contract helloGeeks with a public address variable named userAddress initialized with the Ethereum address 0x71C7656EC7ab88b098defB751B7401B5f6d8976F. The contract includes a function called getUserAddress with view visibility and a return type of address. Invoking this function will return the Ethereum address stored in the userAddress variable, allowing external callers to access and utilize the user’s address within the contract. This functionality facilitates retrieving and exposing address information, enabling seamless interaction with addresses and enhancing the capabilities of smart contracts.
Solidity
pragma solidity ^0.8.0;
contract helloGeeks {
address public userAddress = 0x71C7656EC7ab88b098defB751B7401B5f6d8976F;
function getUserAddress() public view returns (address) {
return userAddress;
}
}
|
Output
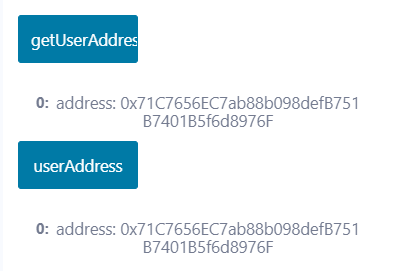
In this example, the getUserAddress function returns the value of the userAddress variable, which is of type address.
The zero address in Solidity, denoted by address(0), is a reserved value that represents an empty or non-existent Ethereum Address. It is commonly used for initialization, comparison operations, and error handling in smart contracts. Here’s an example:
Solidity
pragma solidity ^0.8.0;
contract helloGeeks {
address public emptyAddress = address(0);
}
|
Output
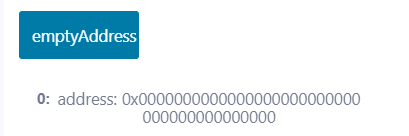
The emptyAddress variable is assigned the value of the zero address using address(0). This indicates that the variable is not associated with a valid Ethereum address at the time of initialization. By leveraging the zero address, developers can ensure proper initialization, handle exceptional cases and enforce secure coding practices when working with addresses in Solidity.
Share your thoughts in the comments
Please Login to comment...