How to Implement Internationalization (i18n) in Redux Applications?
Last Updated :
06 May, 2024
Implementing internationalization (i18n) in a Redux application enhances its accessibility and usability by allowing users to interact with the application in their preferred language. With i18n, your Redux app becomes multilingual, accommodating users from diverse linguistic backgrounds and improving their overall experience.
Approach to Implement Internationalization in Redux Applications
1. Redux Project Structure: Create a Redux project structure with appropriate folders for actions, reducers, components, and other necessary files. Ensure that your Redux setup includes the Redux store configuration, root reducer, and any middleware required.
2. Defining Messages Object: Define a messages object within your Redux application, which will store the language-specific messages loaded from the imported message files. The messages object should have keys representing language codes (e.g., ‘en’, ‘fr’, ‘sp’, ‘hi’, ‘rs’) and values containing the corresponding message objects.
3. Initializing Locale State: Initialize a locale state within your Redux store or component state to keep track of the currently selected language. Set the default language (e.g., ‘en’) as the initial value for the locale state.
4. Handling Language Change: Implement a method or action to handle language changes triggered by the user. This method should update the locale state in the Redux store or component state based on the selected language.
5. Rendering JSX with IntlProvider: Render your Redux components wrapped in an IntlProvider component provided by the react-intl library. Pass the locale and messages as props to the IntlProvider component to provide internationalization context to its child components.
6. Displaying Translated Messages: Within your Redux components, use the FormattedMessage component provided by react-intl to display translated messages. Retrieve the translated messages from the messages object based on the currently selected language (retrieved from the locale state).
Steps to create a React Application and Installing Modules
Step 1: Create a Redux application.
npx create-react-app <-foldername->
Step 2: Move to the project directory.
cd <-foldername->
Step 3: Install necessary dependencies in Redux application.
npm install react-intl redux react-redux
Project Structure
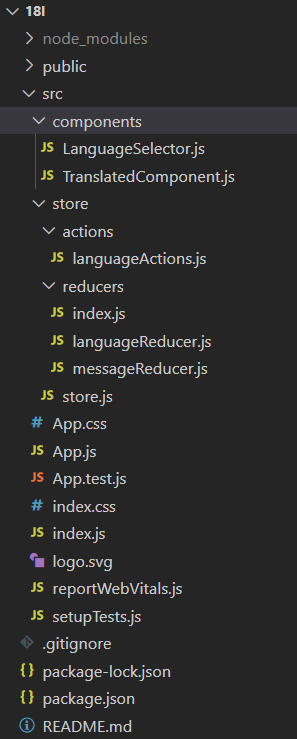
The Updated dependencies in package.json file is:
"dependencies": {
"react": "^18.2.0",
"react-dom": "^18.2.0",
"react-intl": "^6.6.5",
"react-redux": "^9.1.0",
"react-scripts": "5.0.1",
"redux": "^5.0.1"
},
Example: Below is an example of Implementing Internationalization in Redux Application.
JavaScript
// index.js
// this is the project initial file
import React from 'react';
import ReactDOM from 'react-dom/client';
import App from './App';
import reportWebVitals from './reportWebVitals';
import { Provider } from "react-redux";
import store from "./store/store";
const root = ReactDOM.createRoot(document.getElementById('root'));
root.render(
<Provider store={store}>
<App />
</Provider>
);
reportWebVitals();
JavaScript
// src/App.js
// This is the App.js where Language Selector and Translated Components imported
import React from "react";
import { IntlProvider } from "react-intl";
import { useDispatch, useSelector } from "react-redux";
import LanguageSelector from "./components/LanguageSelector";
import TranslatedComponent from "./components/TranslatedComponent";
function App() {
// eslint-disable-next-line no-unused-vars
const dispatch = useDispatch();
const locale = useSelector((state) => state.language.locale);
const messages = useSelector((state) => state.messages[locale]);
return (
<IntlProvider locale={locale} messages={messages}>
<div>
<LanguageSelector />
<TranslatedComponent />
</div>
</IntlProvider>
);
}
export default App;
JavaScript
// LanguageSelector Component
// src/components/LanguageSelector.js
import React from 'react';
import { useDispatch } from 'react-redux';
import { changeLanguage } from '../store/actions/languageActions';
const LanguageSelector = () => {
const dispatch = useDispatch();
const handleChange = (e) => {
dispatch(changeLanguage(e.target.value));
};
return (
<div>
<select onChange={handleChange}>
<option value="en">English</option>
<option value="fr">French</option>
<option value="sp">Spanish</option>
<option value="hi">Hindi</option>
<option value="rs">Russian</option>
</select>
</div>
);
}
export default LanguageSelector;
JavaScript
// Translated Component
// src/components/TranslatedComponent.js
import React from 'react';
import { FormattedMessage } from 'react-intl';
const TranslatedComponent = () => {
return (
<div>
<h1><FormattedMessage id="app.title" /></h1>
<p><FormattedMessage id="app.description" /></p>
</div>
);
};
export default TranslatedComponent;
JavaScript
// src/store/actions/languageActions.js
export const changeLanguage = (language) => {
return {
type: 'CHANGE_LANGUAGE',
payload: language
};
};
JavaScript
// src/store/reducers/index.js
import { combineReducers } from 'redux';
import languageReducer from './languageReducer';
import messageReducer from './messageReducer';
const rootReducer = combineReducers({
language: languageReducer,
messages: messageReducer
});
export default rootReducer;
JavaScript
// src/store/reducers/languageReducer.js
const initialState = {
locale: 'en' // Set initial language to English
};
const languageReducer = (state = initialState, action) => {
switch (action.type) {
case 'CHANGE_LANGUAGE':
return {
...state,
locale: action.payload
};
default:
return state;
}
};
export default languageReducer;
JavaScript
// src/store/reducers/messageReducer.js
const initialState = {
en: {
"app.title": "Redux internationalization (i18n)",
"app.description": "This is a simple example of internationalization in Redux using react-intl."
},
fr: {
"app.title": "Internationalisation Redux (i18n)",
"app.description": "Ceci est un exemple simple d'internationalisation dans Redux en utilisant react-intl."
},
sp: {
"app.title": "Internacionalización Redux (i18n)",
"app.description": "Este es un ejemplo simple de internacionalización en Redux usando react-intl."
},
hi: {
"app.title": "Redux अंतर्राष्ट्रीयकरण (i18n)",
"app.description": "यह Redux में अंतरराष्ट्रीयकरण का एक सरल उदाहरण है जो react-intl का उपयोग करता है।"
},
rs: {
"app.title": "Redux интернационализация (i18n)",
"app.description": "Это простой пример интернационализации в Redux с использованием react-intl."
}
};
const messageReducer = (state = initialState, action) => {
switch (action.type) {
default:
return state;
}
};
export default messageReducer;
JavaScript
// src/store/store.js
import { createStore } from 'redux';
import rootReducer from './reducers';
const store = createStore(rootReducer);
export default store;
Start your application suing the following command:
npm run start
Output:
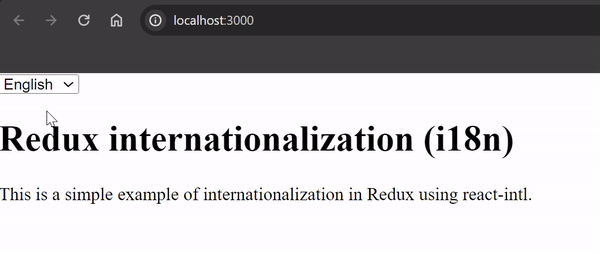
Conclusion
In conclusion, incorporating internationalization into Redux applications enables developers to build multilingual interfaces that accommodate users from diverse linguistic backgrounds. By following the provided approach and steps, you can seamlessly integrate i18n into your Redux projects, ensuring that users receive a localized experience tailored to their language preferences. This not only enhances accessibility but also improves user engagement and satisfaction, making your application more inclusive and user-friendly across different regions and cultures.
FAQs
Why is internationalization important for Redux applications?
Internationalization ensures that Redux applications can be easily adapted to different languages and regions, making them accessible to a global audience. It enhances user experience by allowing users to interact with the application in their preferred language.
2. How can Redux applications support multiple languages?
Redux applications can support multiple languages by managing language-specific content and translations using libraries like react-intl. By organizing message files for different languages and updating the application state accordingly, Redux apps can dynamically display content in the user’s chosen language.
3. What are the benefits of using react-intl with Redux for internationalization?
Integrating react-intl with Redux simplifies the management of internationalization in Redux applications. react-intl provides components and utilities for formatting messages, handling language switching, and managing locale-specific content, streamlining the development process and ensuring consistent localization across the app.
4. How can I handle language changes in a Redux application?
Language changes can be handled in a Redux application by dispatching actions to update the language state in the Redux store. Components can subscribe to changes in the language state and re-render with the updated translations when the language is switched.
Share your thoughts in the comments
Please Login to comment...