How to Implement Caching in React Redux applications ?
Last Updated :
26 Mar, 2024
Caching is the practice of storing frequently used or calculated data temporarily in memory or disk. Its main purpose is to speed up access and retrieval by minimizing the need to repeatedly fetch or compute the same information. By doing so, caching helps reduce latency, conserve resources, and ultimately improve overall performance.
Caching in Redux Application:
In Redux applications, caching involves storing previously fetched data or computed results in memory to avoid redundant operations and optimize performance. Redux, being a predictable state container, manages the application’s state in a single immutable store. While Redux provides a centralized approach to state management, incorporating caching mechanisms can further enhance its efficiency, especially when dealing with data that doesn’t frequently change or requires expensive computations.
Here we going to make simple counter application that reserve count in cache, and when we refresh the page, it fetch count from cache and display as it was.
Steps to implement cache in React Redux application:
Step 1: Make a simple react application
npx create-react-app <-foldername->
Step 2: Move to the application directory:
cd <-foldername->
Step 3: include following dependency to make redux Application
npm install redux react-redux // for redux application
npm install redux-persist // for implementing caching
The updated dependencies in package.json file will look like:
"dependencies": {
"react": "^18.2.0",
"react-redux": "^9.1.0",
"redux": "^5.0.1",
"redux-persist": "^6.0.0"
},
Folder Structure:
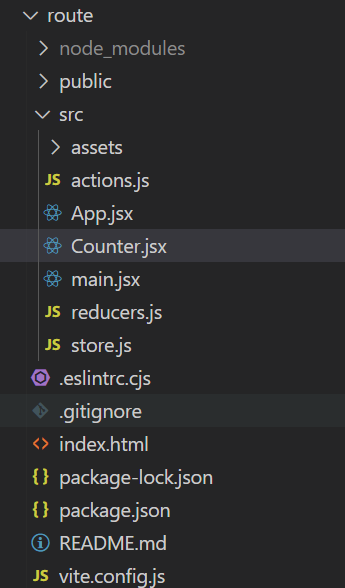
Step 4: Update index.js file (main.jsx file in case of react application with Vite)
Javascript
import React from "react";
import ReactDOM from "react-dom/client";
import App from "./App.jsx";
import { Provider } from "react-redux";
import { PersistGate } from "redux-persist/integration/react";
import { store, persistor } from "./store";
ReactDOM.createRoot(document.getElementById("root")).render(
<React.StrictMode>
<Provider store={store}>
<PersistGate loading={null} persistor={persistor}>
<App />
</PersistGate>
</Provider>
,
</React.StrictMode>
);
Step 5: Update App.jsx and make a component Counter.jsx
Javascript
// App.jsx
import Counter from "./Counter";
function App() {
return (
<div className="App">
<h1>Redux Counter App</h1>
<Counter />
</div>
);
}
export default App;
Javascript
// Counter.jsx
import { useSelector, useDispatch } from "react-redux";
import { increment, decrement, reset } from "./actions";
const Counter = () => {
const count = useSelector((state) => state.count);
const dispatch = useDispatch();
return (
<div>
<h2>Counter: {count}</h2>
<button onClick={() => dispatch(increment())}>
Increment
</button>
<button onClick={() => dispatch(decrement())}>
Decrement
</button>
<button onClick={() => dispatch(reset())}>
Reset
</button>
</div>
);
};
export default Counter;
Step 6: Make three more js file as ‘store.js’, ‘reducers.js‘ and ‘actions.js‘ in src
Javascript
// store.js
import { createStore } from 'redux';
import { persistStore, persistReducer } from 'redux-persist';
import storage from 'redux-persist/lib/storage';
import counterReducer from './reducers';
const persistConfig = {
key: 'root',
storage
};
const persistedReducer = persistReducer(persistConfig, counterReducer);
const store = createStore(persistedReducer);
const persistor = persistStore(store);
export { store, persistor };
Javascript
// actions.js
export const increment = () => {
return {
type: 'INCREMENT'
};
};
export const decrement = () => {
return {
type: 'DECREMENT'
};
};
export const reset = () => {
return {
type: 'RESET'
};
};
Javascript
// reducers.js
const initialState = {
count: 0
};
const counterReducer = (state = initialState, action) => {
switch (action.type) {
case 'INCREMENT':
return {
count: state.count + 1
};
case 'DECREMENT':
return {
count: state.count - 1
};
case 'RESET':
return {
count: 0
};
default:
return state;
}
};
export default counterReducer;
Step 7: Run application and update counter and then refresh page.
npm run start
In case of react with Vite
npm run dev
Output:
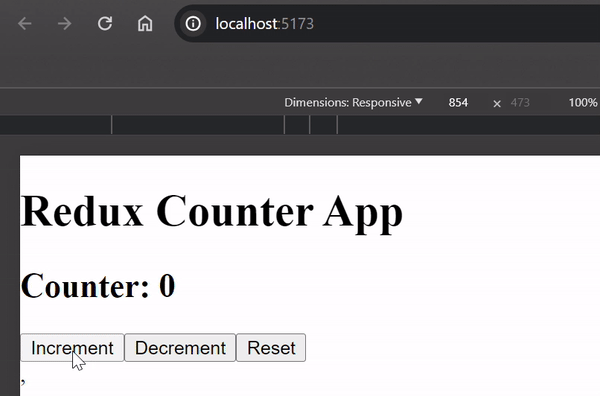
Share your thoughts in the comments
Please Login to comment...