Servlet – Internationalization with Examples
Last Updated :
25 Mar, 2022
By default, web pages are available in the English language. It can be provided in the user choice of languages by means of the “Internationalization” concept in Java technology. Internationalization (i18n) helps the users to view the pages according to their nationality or else visited location. Requester’s locale can be retrieved by a servlet depending on their nationality and it will return the Locale object.
java.util.Locale request.getLocale()
There are different methods available to detect the locale
S.N
|
Method
|
Description
|
1 |
getCountry() |
It helps to return “country/region” code in UPPER CASE for the locale in ISO 3166 2-letter format. |
2 |
getDisplayCountry() |
It helps to return a “NAME” for the locale’s country and it is appropriate for display to the user. |
3 |
getLanguage() |
It helps to return the “LANGUAGE CODE” in lower case for the locale in ISO 639 format. |
4 |
getDisplayLanguage() |
It helps to return a “NAME FOR LOCALE’s LANGUAGE” and it is appropriate for display to the user. |
5. |
getISO3Country() |
It helps to return a three-letter abbreviation for the locale’s country. |
6 |
getISO3Language() |
It helps to return a three-letter abbreviation for the locale’s language. |
Examples
Let us see different examples to display a language and associated country for a request
Example 1:
Java
import java.io.IOException;
import java.io.PrintWriter;
import java.util.Locale;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
@WebServlet ( "/getCurrentLocationInformation" )
public class GetLocationServlet extends HttpServlet {
private static final long serialVersionUID = 1L;
public GetLocationServlet() {
super ();
}
protected void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
Locale locale = request.getLocale();
String localeLanguage = locale.getLanguage();
String localeCountry = locale.getCountry();
response.setContentType( "text/html" );
PrintWriter printWriter = response.getWriter();
String title = "Locale detection" ;
String docType =
"<!doctype html public \"-//w3c//dtd html 4.0 " + "transitional//en\">\n" ;
printWriter.println(docType +
"<html>\n" +
"<head><title>" + title + "</title></head>\n" +
"<body bgcolor = \"#145A32\">\n" +
"<h1 align = \"Left\"> " + "<font color=\"white\">" + "Language : " + localeLanguage + "</font>" + "</h1>\n" +
"<h2 align = \"Left\">" + "<font color=\"white\">" + "Country : " + localeCountry + "</font>" + "</h2>\n" +
"</body>" +
"</html>"
);
}
}
|
By executing the above code, we can get the output as follows
Output:
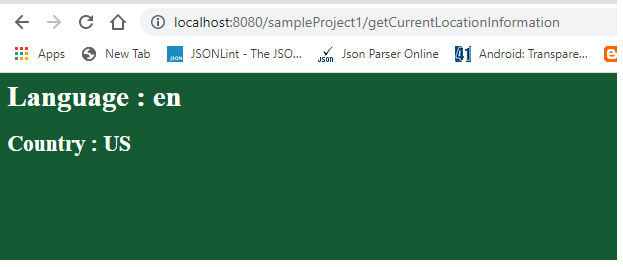
Default language setting
If we want to set the language as the different one, via language setting it can be done.
// In order to set spanish language code.
response.setHeader("Content-Language", "es");
// Similarly we can set different language codes in this way
// http://www.mathguide.de/info/tools/languagecode.html provides various language code
Example 2:
Java
protected void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
response.setContentType( "text/html" );
PrintWriter printWriter = response.getWriter();
response.setHeader( "Content-Language" , "es" );
String title = "En Español" ;
String docType =
"<!doctype html public \"-//w3c//dtd html 4.0 " + "transitional//en\">\n" ;
printWriter.println(docType +
"<html>\n" +
"<head><title>" + title + "</title></head>\n" +
"<body bgcolor = \"#145A32\">\n" +
"<h1>" + "<font color=\"white\">" + "En Español:" + "</font>" + "</h1>\n" +
"<h1>" + "<font color=\"white\">" + "Bienvenido a GeeksForGeeks!" + "</font>" + "</h1>\n" +
"</body>" +
"</html>" );
}
|
Output:
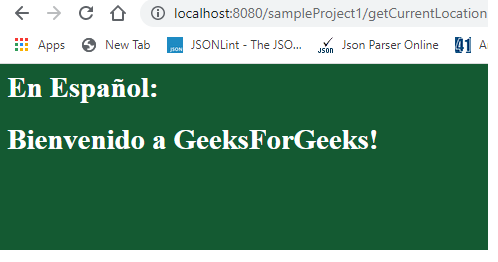
setting to spanish language
Example 3:
Specific to locale, by using java.text.DateFormat class and getDateTimeInstance() method, we can format date and time
Java
protected void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
response.setContentType( "text/html" );
PrintWriter printWriter = response.getWriter();
Locale locale = request.getLocale( );
String date = DateFormat.getDateTimeInstance(DateFormat.FULL,
DateFormat.SHORT, locale).format( new Date( ));
String title = "Displaying Locale Specific Dates" ;
String docType =
"<!doctype html public \"-//w3c//dtd html 4.0 " + "transitional//en\">\n" ;
printWriter.println(docType +
"<html>\n" +
"<head><title>" + title + "</title></head>\n" +
"<body bgcolor = \"#145A32\">\n" +
"<h1 align = \"Left\">" + "<font color=\"white\">" + "Locale specific date : " + date + "</font>" + "</h1>\n" +
"</body>" +
"</html>"
);
}
|
Output:
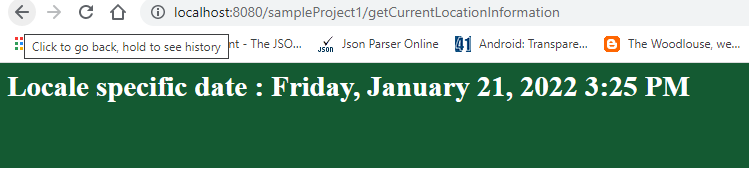
Displaying locale date
Example 4:
Specific to locale, by using java.txt.NumberFormat class and getCurrencyInstance() method, we can format currency
Java
protected void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
response.setContentType( "text/html" );
PrintWriter printWriter = response.getWriter();
Locale currentLocale = request.getLocale( );
NumberFormat numberFormat = NumberFormat.getCurrencyInstance(currentLocale);
String formattedCurrency = numberFormat.format( 100000 );
String title = "Displaying Locale Specific Currency" ;
String docType =
"<!doctype html public \"-//w3c//dtd html 4.0 " + "transitional//en\">\n" ;
printWriter.println(docType +
"<html>\n" +
"<head><title>" + title + "</title></head>\n" +
"<body bgcolor = \"#145A32\">\n" +
"<h1 align = \"Left\">" + "<font color=\"white\">" + "Currency : " +formattedCurrency + "</font>" + "</h1>\n" +
"</body>" +
"</html>"
);
}
|
Output:
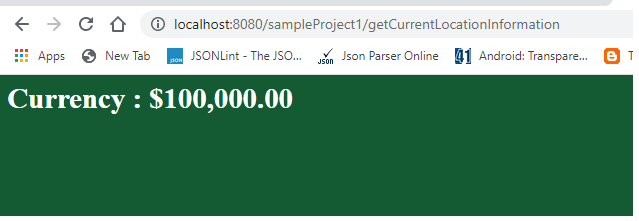
Advantages of Internationalization
- Foreign marketplaces capturing is easier.
- Rendering a locale-neutral result
- In the beginning, if it is implemented, the expansion of software across global markets is easier.
Conclusion
Any software that aims to be developed in the global market, has to be prepared in an international way only. In java, we can use the above-mentioned methodologies to achieve internationalization.
Share your thoughts in the comments
Please Login to comment...