Adding Internationalization (i18n) in Next.js Application
Last Updated :
05 Apr, 2024
Implementing internationalization (i18n) in a NextJS application enhances its accessibility and usability by catering to users who speak different languages. With i18n, your app becomes multilingual, ensuring that users from diverse linguistic backgrounds can navigate and understand its content effortlessly.
Approach to Implement Internationalization in Next.js Application:
- NextJS project Structure: We’ll create a next JS named App to manage internationalization using the react-intl library. This component will serve as the entry point for our Next.js application.
- Importing Message Files: We’ll import message files for different languages (e.g., en.json, fr.json, sp.json, hindi.json, and rus.json) from a local directory within the Next.js project.
- Defining Messages Object: The messages object will be defined with keys representing language codes and values containing the corresponding message objects extracted from the JSON files.
- Initializing Locale State: The App component will have a state named locale, initialized with the default language ‘en’. This state will determine the currently selected language.
- Handling Language Change: We’ll implement a method named handleLanguageChange to update the locale state based on the language selected from a dropdown menu. This dropdown menu will allow users to switch between different languages.
- Rendering JSX with IntlProvider: The render method of the App component will return JSX wrapped in an IntlProvider component. This component will receive locale and messages as props, providing internationalization context to its child components.
- Displaying Translated Messages: Inside the IntlProvider, we’ll include a div containing an h1 tag with a title message, a select dropdown for language selection, and a p tag with a description message. These messages will be displayed using the FormattedMessage component, which retrieves them by their unique IDs defined in the message files for each language.
Steps to Create a Next JS App and Installing Module:
Step 1: Create a nextjs application
npx create-next-app <-foldername->
Step 2: Move to the project directory
cd <-foldername->
Step 3: Install necessary dependencies in next application
npm install react-intl
Project Structure:
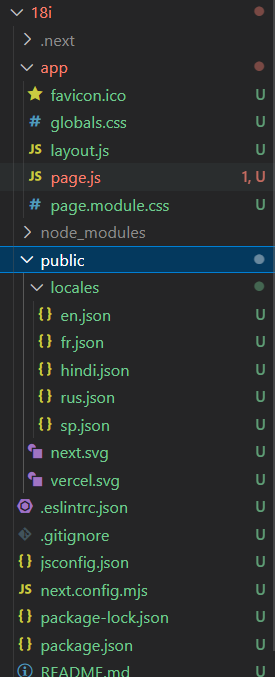
Updated dependencies in package.json:
"dependencies": {
"next": "14.1.4",
"react": "^18",
"react-dom": "^18",
"react-intl": "^6.6.4"
},
Example: Below is an example of Implementing Internationalization in Next JS Application.
JavaScript
// Page.js Entry point
'use client'
import React, {
useState
} from 'react';
import {
FormattedMessage,
IntlProvider
} from 'react-intl';
import messages_en from '../public/locales/en.json';
import messages_fr from '../public/locales/fr.json';
import messages_sp from '../public/locales/sp.json';
import messages_hi from '../public/locales/hindi.json';
import messages_rs from '../public/locales/rus.json';
const messages = {
en: messages_en,
fr: messages_fr,
sp: messages_sp,
hi: messages_hi,
rs: messages_rs,
};
const App = () => {
const [locale, setLocale] = useState('en');
const handleLanguageChange = (e) => {
setLocale(e.target.value);
};
return (
<IntlProvider locale={locale} messages={messages[locale]}>
<div>
<h1><FormattedMessage id="app.title" /></h1>
<select onChange={handleLanguageChange} value={locale}>
<option value="en">English</option>
<option value="fr">French</option>
<option value="sp">Spanish</option>
<option value="hi">Hindi</option>
<option value="rs">Russian</option>
</select>
<p><FormattedMessage id="app.description" /></p>
</div>
</IntlProvider>
);
};
export default App;
JavaScript
//en.json
{
"app.title": "Next internationalization (i18n)",
"app.description": "This is a simple example of internationalization in Next using react-intl."
}
JavaScript
// fr.json
{
"app.title": "Internationalisation de Next (i18n)",
"app.description": "Ceci est un exemple simple d'internationalisation dans Next en utilisant react-intl."
}
JavaScript
//sp.json
{
"app.title": "Internacionalización en Next (i18n)",
"app.description": "Este es un ejemplo simple de internacionalización en Next usando react-intl."
}
JavaScript
//hindi.json
{
"app.title": "Next में अंतर्राष्ट्रीयकरण (i18n)",
"app.description": "यह एक सरल उदाहरण है Next में अंतर्राष्ट्रीयकरण का उपयोग करते हुए react-intl का प्रयोग करने का।"
}
JavaScript
//rus.json
{
"app.title": "Международная локализация в Next (i18n)",
"app.description": "Это простой пример международной локализации в Next с использованием react-intl."
}
Start your application suing the following command:
npm run dev
Output:
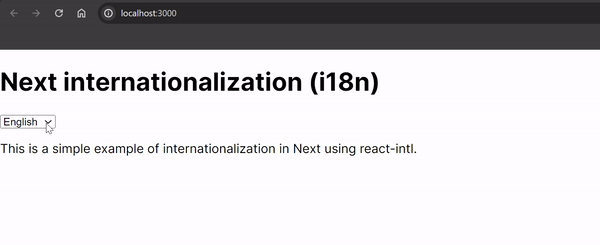
Conclusion:
In conclusion, internationalization remains a fundamental aspect of Next.js development, empowering developers to create applications that transcend borders and embrace diversity. With Next.js, incorporating support for multiple languages becomes not only achievable but also seamless, allowing developers to cater to a global audience effortlessly. By implementing internationalization in Next.js applications, developers can ensure that users from various linguistic backgrounds feel included and valued. Through the utilization of tools like react-intl, Next.js developers can efficiently manage translations and provide localized content, thus enhancing accessibility and user experience.
Frequently Asked Questions on Internationalization in Next.js -FAQs:
Why is internationalization important for NextJS apps?
Internationalization is essential for Next.js apps to cater to a global audience, providing a seamless user experience for users who speak different languages. It helps in breaking down language barriers and making the app accessible to a wider range of users.
How can I add internationalization to my NextJS app?
You can add internationalization to your Next.js app by using libraries like react-intl or next-i18next. These libraries provide tools and utilities to manage translations, handle locale switching, and format localized content.
Can I support multiple languages in my NextJS app?
Yes, Next.js allows you to support multiple languages by organizing translations into separate language JSON files and dynamically loading the appropriate translations based on the user’s locale.
Is there a preferred approach for routing in multilingual NextJS apps?
While Next.js supports various routing approaches, including file-based routing and dynamic routing, there isn’t a one-size-fits-all solution for multilingual apps. The preferred approach depends on factors like the complexity of your app and your specific requirements for language handling and SEO.
Share your thoughts in the comments
Please Login to comment...