Getting Started with VueJS
Last Updated :
22 Mar, 2024
VueJS is a JavaScript framework for creating user interfaces. Vue.js, often simply referred to as Vue, is known for its simplicity and flexibility. Vue has gained widespread popularity among developers for its ease of integration. Vuejs is also used for single-page web applications, the same as ReactJS.
What is VueJs?
It’s a JavaScript framework that makes it easy to build web interfaces. With VueJS, you can create dynamic and interactive elements on your website without hard work. It’s user-friendly and flexible, making it a great choice for both beginners and experienced developers. This can be used in many different ways:
- Creating dynamic HTML
- Single page application
- Server-side rendering
- Used for mobile, desktop or terminal
How to set up VueJS and create a VueJS project?
You can follow the below steps to setup and create a VueJS project:
Step 1: Install nodejs from nodejs.org
Step 2: Install dependencies using the below npm command.
npm install -g @vue/cli
Step 3: Create and visit your VueJS project
vue create your_project_name
cd your-project-name
Step 4: Run the node server to run the code
npm run serve
Important concepts of VueJS
Data Binding
It will make sure that your data whenever changes, will make visible to user interface. Whenever anyone type anything in the input box it will refleceted on the screen and visible to the user.
Javascript
<template>
<div>
<h1 style="color: green">
GeeksforGeeks
</h1>
<input v-model="userInput"
placeholder="Type your message" />
<p>{{ userInput }}</p>
</div>
</template>
<script>
export default {
data() {
return {
userInput: '',
};
},
};
</script>
Component and props
Your web page is created using many different components. It is piece of code, which can be used multiple time and not have to define again and again.
Javascript
<!-- ParentComponent.vue -->
<template>
<div>
<h1>
{{ parentMessage }}
</h1>
<ChildComponent :childProp="parentMessage" />
</div>
</template>
<script>
import ChildComponent from
'./ChildComponent.vue';
export default {
components: {
ChildComponent,
},
data() {
return {
parentMessage:
'Hello from Parent!',
};
},
};
</script>
JavaScript
<!-- ChildComponent.vue -->
<template>
<div>
<p>
{{ childProp }}
</p>
</div>
</template>
<script>
export default {
props: ['childProp'],
};
</script>
Directives
These are like commands for VueJS. It start from ‘v-‘ and tell VueJS to do something. Example v-if decides whether to show or hide an element.
Javascript
<template>
<div>
<p v-if="showMessage">
This message is visible if showMessage is true.
</p>
<ul>
<li v-for="item in items">
{{ item }}
</li>
</ul>
</div>
</template>
<script>
export default {
data() {
return {
showMessage: true,
items: ['Item 1', 'Item 2', 'Item 3'],
};
},
};
</script>
Computed Properties
Computed properties in Vue.js enable the creation of reactive properties based on other data properties.
Javascript
<template>
<div>
<input v-model="inputText"
placeholder="Type your message" />
<p>
Message length:
{{ messageLength }}
</p>
</div>
</template>
<script>
export default {
data() {
return {
inputText: '',
};
},
computed: {
messageLength() {
return this.
inputText.length;
},
},
};
</script>
Vue Routes
Vue Router facilitates navigation and the creation of Single Page Applications (SPAs). Also run a command to install the vue-router.
npm install vue-router
Javascript
// main.js file
import { createApp } from 'vue';
import App from './App.vue';
import { createRouter, createWebHistory } from 'vue-router';
import HomePage from './HomePage.vue';
import AboutPage from './AboutPage.vue';
const router = createRouter({
history: createWebHistory(),
routes: [
{
path: '/',
component: HomePage
},
{
path: '/about',
component: AboutPage
}
]
});
createApp(App).use(router).mount('#app');
JavaScript
<!-- HomePage.vue -->
<template>
<div>
<h1>
Welcome to the Home Page
</h1>
<p>
This is the home page content.
</p>
</div>
</template>
<script>
export default {
name: 'HomePage',
};
</script>
<style scoped>
/* Add scoped styles here if needed */
</style>
JavaScript
<!-- AboutPage.vue -->
<template>
<div>
<h1>
About Us
</h1>
<p>
This is the about page content.
</p>
</div>
</template>
<script>
export default {
name: 'AboutPage',
};
</script>
<style scoped>
/* Add scoped styles here if needed */
</style>
JavaScript
<!-- App.vue -->
<template>
<div id="app">
<router-link to="/">Home</router-link> |
<router-link to="/about">About</router-link>
<router-view></router-view>
</div>
</template>
<script>
export default {
name: 'App',
};
</script>
<style>
#app {
font-family: Arial, sans-serif;
text-align: center;
margin-top: 20px;
}
</style>
Creating a simple notes app using VueJS
It is a simple notes taking or ToDo application which allows you to add as well as delete the items. You can create an task you have to do in future and delete it once you have complete it.
Example: The below VueJS code will help you in creating the simple notes taking app.
Javascript
<!-- App.vue -->
<template>
<div id="app">
<h2 style="text-align: center;">
A Simple Notes App using VueJS
</h2>
<div class="input-container">
<input v-model="newNote"
placeholder="Type your note" />
<button @click="addNote">
Add Note
</button>
</div>
<NoteTaking v-for="note in notes" :key="note.id"
:note="note" @delete="deleteNote" />
</div>
</template>
<script>
import NoteTaking from './NoteTaking.vue';
export default {
components: {
NoteTaking,
},
data() {
return {
newNote: '',
notes: [],
nextNoteId: 1,
};
},
methods: {
addNote() {
if (this.newNote.trim() === '') return;
this.notes.push({
id: this.nextNoteId++,
text: this.newNote
});
this.newNote = '';
},
deleteNote(noteId) {
this.notes = this.notes.
filter((note) => note.id !== noteId);
},
},
};
</script>
<style>
#app {
max-width: 600px;
margin: 0 auto;
padding: 20px;
}
.input-container {
margin-bottom: 20px;
display: flex;
justify-content: space-between;
align-items: center;
}
.input-container input {
flex-grow: 1;
padding: 10px;
margin-right: 10px;
border: 1px solid #ddd;
border-radius: 5px;
}
.input-container button {
background-color: #3498db;
color: #fff;
border: none;
padding: 10px 20px;
border-radius: 3px;
cursor: pointer;
}
.input-container button:hover {
background-color: #2980b9;
}
</style>
Javascript
<!-- NoteTaking.vue -->
<template>
<div class="note">
<p>{{ note.text }}</p>
<button @click="deleteNote">
Delete
</button>
</div>
</template>
<script>
export default {
props: ['note'],
methods: {
deleteNote() {
this.$emit('delete', this.note.id);
},
},
};
</script>
<style scoped>
.note {
display: flex;
align-items: center;
justify-content: space-between;
background-color: #f9f9f9;
margin-bottom: 15px;
padding: 15px;
border-radius: 5px;
box-shadow: 0 2px 4px rgba(0, 0, 0, 0.1);
}
.note p {
margin: 0;
}
.note button {
background-color: #e74c3c;
color: #fff;
border: none;
padding: 8px 15px;
border-radius: 3px;
cursor: pointer;
}
.note button:hover {
background-color: #c0392b;
}
</style>
Output:
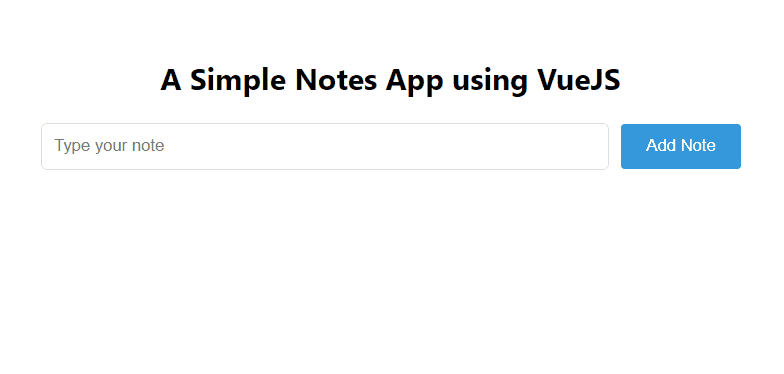
FAQs
1. What is Vue used for?
Vue is used for building user interfaces in web applications, providing a flexible and reactive framework for creating interactive and dynamic frontend experiences.
2. Why use Vue instead of React?
The choice between Vue and React depends on preference and project requirements. Vue is often preferred for its simplicity and ease of integration, while React is chosen for its larger ecosystem and adoption by major companies like Facebook.
3. Is VueJS a programming language?
No, VueJS is not a programming language. It is a JavaScript framework for building user interfaces.
4. Is VueJS a frontend framework?
Yes, VueJS is a frontend framework. It focuses on the view layer of web applications, allowing developers to build interactive and dynamic user interfaces.
Share your thoughts in the comments
Please Login to comment...