Explain the concept of “lifting up in React Hooks”
Last Updated :
26 Feb, 2024
“Lifting State Up” is a concept in React that involves moving the state of a component higher up in the component tree. In React, the state is typically managed within individual components, but there are situations where multiple components need to share and synchronize the same state. When this happens, you can lift the state to a common ancestor component that is higher in the component hierarchy.
Concept of Lifting State Up:
- Identify Shared State: Determine which components need to share the same state. If two or more components need access to the same piece of state data, it might be a good candidate for lifting the state.
- Move State to a Common Ancestor: Identify a common ancestor component that is higher in the component tree and is an ancestor to all the components that need access to the state. Lift the state up to this common ancestor component.
- Pass State Down as Props: Once the state is moved to the common ancestor, pass it down to the components that need access to it as props. This allows child components to receive and use the shared state.
- Handle State Changes in Common Ancestor: If any component needs to modify the shared state, pass down callback functions as props to allow child components to notify the common ancestor about state changes. The common ancestor can then update the state and propagate the changes downward.
Example: Below is an example of lifting state up in React Hooks.
Javascript
import React, {
useState
} from 'react' ;
const CounterDisplay = ({ counter }) => {
return <p>Counter: {counter}</p>;
};
const CounterButton = ({ onIncrement }) => {
return <button
onClick={onIncrement}>
Increment Counter
</button>;
};
const App = () => {
const [counter, setCounter] = useState(0);
const handleIncrement = () => {
setCounter(counter + 1);
};
return (
<div>
<CounterDisplay counter={counter} />
<CounterButton
onIncrement={handleIncrement} />
</div>
);
};
export default App;
|
Output:
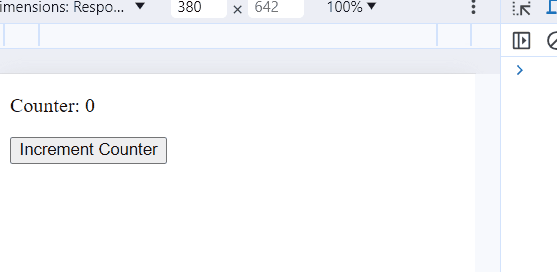
Output
Share your thoughts in the comments
Please Login to comment...