Environment Protection Website using React
Last Updated :
14 Feb, 2024
Imagine building a website that champions environmental protection. That’s exactly what we’re aiming for with this project. We’re crafting a space using React where people can learn, share, and engage in all things related to safeguarding our planet.
Output Preview: Let us have a look at how the final output will look like.
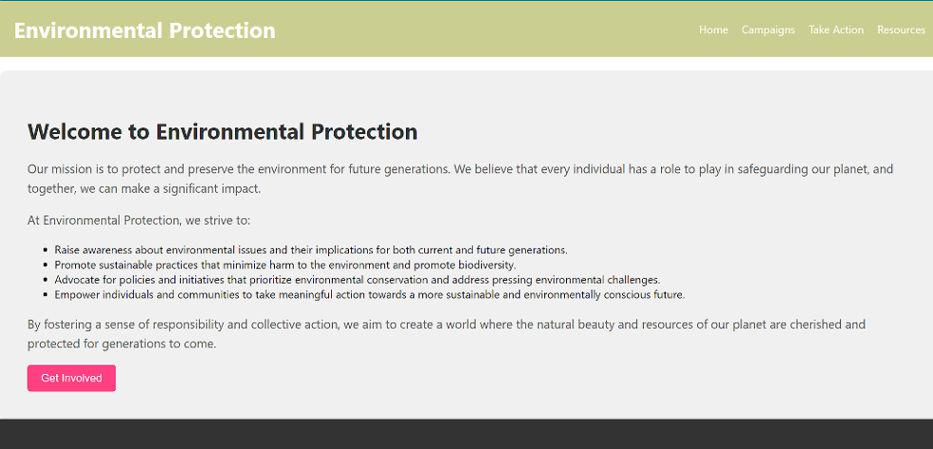
Prerequisites:
Approach to create Environment Protection Website:
- Create a new React project using Create React App to kickstart development.
- Component Structure: Organize the project by defining components for different sections:
- Home (home.js): Introduce visitors to the website’s mission and purpose.
- Header (header.js): Design a consistent header for easy navigation across pages.
- Footer (footer.js): Develop a footer section for additional information and links.
- Resources (resources.js): Compile articles, videos, and tools for environmental protection.
- Take Action (takeaction.js): Encourage visitors to take action through petitions or volunteering.
- Campaign (campaign.js): Highlight ongoing campaigns or initiatives related to environmental protection.
- Apply CSS or a framework like Bootstrap to style each component consistently and enhance visual appeal.
- Implement React Router to enable seamless navigation between different sections of the website.
Steps to Create React App and Installing the module
Step 1: Begin by setting up a new React project using Create React App.
npx create-react-app environmental-protection-website
Step 2: Navigate to the root directory of your project using the following command.
cd environmental-protection-website
Step 3: Install the necessary package in your application using the following command.
npm install react-router-dom react-transition-group
Step 4: Designate components for each page (Home, Resources, TakeAction, Header, Footer).
Project structure:
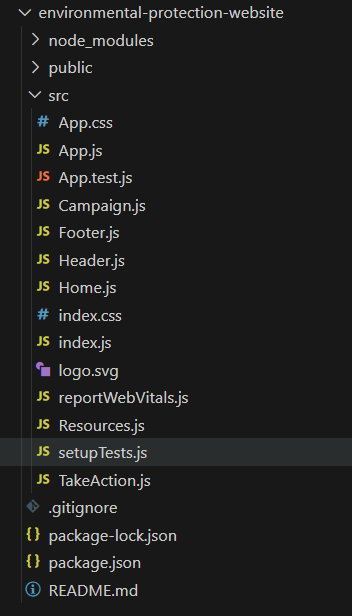
The updated dependencies in package.json file will look like:
"dependencies": {
"@testing-library/jest-dom": "^5.17.0",
"@testing-library/react": "^13.4.0",
"@testing-library/user-event": "^13.5.0",
"react": "^18.2.0",
"react-dom": "^18.2.0",
"react-router-dom": "^6.22.0",
"react-scripts": "5.0.1",
"react-transition-group": "^4.4.5",
"web-vitals": "^2.1.4"
}
Example: Write the following code in respective files.
- Home.js :Displays a welcome message and the organization’s mission, goals, and actions.
- Header.js:Represents the header section with a logo and navigation links.
- Campaigns.js :Displays current campaigns or initiatives with titles and descriptions.
- Footer.js: Represents the footer section with contact information and social media links.
- Resources.js: Displays educational resources related to environmental protection.
- TakeAction.js: Encourages visitors to take action for environmental causes.
Javascript
import React from 'react' ;
import './App.css' ;
const Home = () => {
return (
<div className= "home" >
<div className= "container" >
<h2>Welcome to Environmental Protection</h2>
<p>Our mission is to protect and preserve the
environment for future generations. We believe
that every individual has a role to play in
safeguarding our planet, and together, we can
make a significant impact.
</p>
<p>At Environmental Protection, we strive to:</p>
<ul>
<li>Raise awareness about environmental issues
and their implications for both current and
future generations.
</li>
<li>Promote sustainable practices that minimize
harm to the environment and promote biodiversity.
</li>
<li>Advocate for policies and initiatives that
prioritize environmental conservation and address
pressing environmental challenges.
</li>
<li>Empower individuals and communities to take
meaningful action towards a more sustainable
and environmentally conscious future.
</li>
</ul>
<p>By fostering a sense of responsibility and
collective action, we aim to create a world
where the natural beauty and resources of
our planet are cherished and protected for
generations to come.
</p>
<button className= "action-button" >Get Involved</button>
</div>
</div>
);
}
export default Home;
|
Javascript
import React from 'react' ;
import { Link } from 'react-router-dom' ;
import { CSSTransition }
from 'react-transition-group' ;
const Header = () => {
return (
<CSSTransition in ={ true } appear={ true }
timeout={500} classNames= "fade" >
<header className= "header" >
<div className= "container" >
<h1 className= "logo" >
Environmental Protection</h1>
<nav className= "nav" >
<ul>
<li><Link to= "/"
className= "link" >Home</Link></li>
<li><Link to= "/campaigns"
className= "link" >Campaigns</Link></li>
<li><Link to= "/take-action"
className= "link" >Take Action</Link></li>
<li><Link to= "/resources"
className= "link" >Resources</Link></li>
</ul>
</nav>
</div>
</header>
</CSSTransition>
);
}
export default Header;
|
Javascript
import React from 'react' ;
import './App.css' ;
const Campaigns = () => {
return (
<div className= "campaigns" >
<div className= "container" >
<h2>Current Campaigns</h2>
<div className= "campaign" >
<h3>Campaign Title 1</h3>
<p>Description of Campaign 1...</p>
</div>
<div className= "campaign" >
<h3>Campaign Title 2</h3>
<p>Description of Campaign 2...</p>
</div>
</div>
</div>
);
}
export default Campaigns;
|
Javascript
import React from 'react' ;
import './App.css' ;
const Footer = () => {
return (
<footer className= "footer" >
<div className= "container" >
<div className= "footer-content" >
<div className= "contact-info" >
<h3>Contact Us</h3>
<p>Email: info@example.com</p>
<p>Phone: 123-456-7890</p>
</div>
<div className= "social-media" >
<h3>Connect With Us</h3>
<ul>
<li>
</li>
<li>
</li>
<li>
</a></li>
</ul>
</div>
</div>
<div className= "copyright" >
<p>© 2024 Environmental Protection.
All rights reserved.</p>
</div>
</div>
</footer>
);
}
export default Footer;
|
Javascript
import React from 'react' ;
import './App.css' ;
const Resources = () => {
return (
<div className= "resources" >
<div className= "container" >
<h2>Educational Resources</h2>
<div className= "resource" >
<h3>Resource Title 1</h3>
<p>Description of Resource 1...</p>
</div>
<div className= "resource" >
<h3>Resource Title 2</h3>
<p>Description of Resource 2...</p>
</div>
</div>
</div>
);
}
export default Resources;
|
Javascript
import React from 'react' ;
import './App.css' ;
const TakeAction = () => {
return (
<div className= "take-action" >
<div className= "container" >
<h2>Take Action</h2>
<p>Here are some ways you can get involved:</p>
<ul>
<li>Sign petitions</li>
<li>Volunteer for events</li>
<li>Donate to support our cause</li>
</ul>
</div>
</div>
);
}
export default TakeAction;
|
Javascript
import React from 'react' ;
import { BrowserRouter as Router, Routes, Route } from 'react-router-dom' ;
import Header from './Header' ;
import Home from './Home' ;
import Campaigns from './Campaign' ;
import TakeAction from './TakeAction' ;
import Resources from './Resources' ;
import Footer from './Footer' ;
import './App.css' ;
function App() {
return (
<Router>
<div className= "App" >
<Header />
<Routes>
<Route path= "/"
element={<Home />} />
<Route path= "/campaigns"
element={<Campaigns />} />
<Route path= "/take-action"
element={<TakeAction />} />
<Route path= "/resources"
element={<Resources />} />
</Routes>
<Footer />
</div>
</Router>
);
}
export default App;
|
CSS
.header {
background-color : #CBCE91 FF;
color : #FFFFFF ;
padding : 20px ;
}
.header .container {
display : flex;
justify- content : space-between;
align-items: center ;
}
.header .logo {
margin : 0 ;
}
.header .nav ul {
list-style-type : none ;
margin : 0 ;
padding : 0 ;
}
.header .nav ul li {
display : inline ;
margin-right : 20px ;
}
.header .nav ul li:last-child {
margin-right : 0 ;
}
.header .nav ul li a {
color : #FFFFFF ;
text-decoration : none ;
}
.header .nav ul li a:hover {
text-decoration : underline ;
}
.home {
background-color : #F0F0F0 ;
padding : 40px ;
margin-top : 20px ;
border-radius: 10px ;
box-shadow: 0 4px 8px rgba( 0 , 0 , 0 , 0.1 );
}
.home h 2 {
color : #333333 ;
font-size : 32px ;
margin-bottom : 20px ;
}
.home p {
color : #666666 ;
font-size : 18px ;
line-height : 1.6 ;
}
.action-button {
background-color : #EA738D FF;
color : #FFFFFF ;
padding : 10px 20px ;
border : none ;
border-radius: 5px ;
font-size : 16px ;
cursor : pointer ;
transition: background-color 0.3 s ease;
}
.action-button:hover {
background-color : #FF4081 FF;
}
.footer {
background-color : #333333 ;
color : #FFFFFF ;
padding : 40px ;
}
.footer .container {
display : flex;
justify- content : space-between;
align-items: center ;
}
.footer .footer-content {
display : flex;
}
.footer .footer-content .contact-info,
.footer .footer-content .social-media {
margin-right : 40px ;
}
.footer h 3 {
font-size : 20px ;
}
.footer p {
font-size : 16px ;
}
.footer ul {
list-style-type : none ;
padding : 0 ;
}
.footer ul li {
margin-bottom : 10px ;
}
.footer ul li a {
color : #FFFFFF ;
text-decoration : none ;
}
.footer ul li a:hover {
text-decoration : underline ;
}
.footer .copyright {
font-size : 14px ;
}
.campaigns {
background-color : #F0F0F0 ;
padding : 40px 0 ;
}
.campaigns .container {
max-width : 800px ;
margin : 0 auto ;
}
.campaigns h 2 {
color : #333333 ;
font-size : 28px ;
margin-bottom : 20px ;
}
.campaign {
background-color : #FFFFFF ;
padding : 20px ;
margin-bottom : 20px ;
border-radius: 5px ;
box-shadow: 0 2px 4px rgba( 0 , 0 , 0 , 0.1 );
}
.campaign h 3 {
color : #333333 ;
font-size : 20px ;
}
.campaign p {
color : #666666 ;
}
.resources {
background-color : #F0F0F0 ;
padding : 40px 0 ;
}
.resources .container {
max-width : 800px ;
margin : 0 auto ;
}
.resources h 2 {
color : #333333 ;
font-size : 28px ;
margin-bottom : 20px ;
}
.resource {
background-color : #FFFFFF ;
padding : 20px ;
margin-bottom : 20px ;
border-radius: 5px ;
box-shadow: 0 2px 4px rgba( 0 , 0 , 0 , 0.1 );
}
.resource h 3 {
color : #333333 ;
font-size : 20px ;
}
.resource p {
color : #666666 ;
}
.take-action {
background-color : #F0F0F0 ;
padding : 40px 0 ;
}
.take-action .container {
max-width : 800px ;
margin : 0 auto ;
}
.take-action h 2 {
color : #333333 ;
font-size : 28px ;
margin-bottom : 20px ;
}
.take-action p {
color : #666666 ;
margin-bottom : 20px ;
}
.take-action ul {
padding : 0 ;
}
.take-action li {
color : #333333 ;
font-size : 16px ;
margin-bottom : 10px ;
}
|
Start your application using the following command.
npm start
Output: Open your web browser and visit http://localhost:3000 to see the website in action.
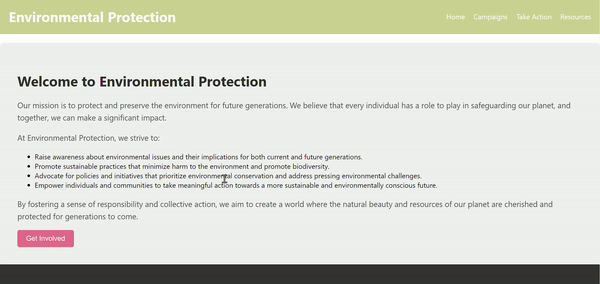
Share your thoughts in the comments
Please Login to comment...