Create Tour and Travels website template using React-Native
Last Updated :
25 Dec, 2023
The tour and travel app template with React Native offers a user-friendly interface with various options required in any travel app. In this article, we will create a basic Tour and Travels website template using React Native.
Preview of final output: Let us have a look at how the final output will look like.
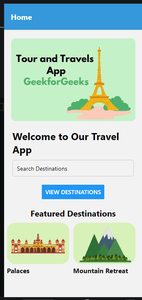
Preview
Prerequisite:
Approach to create Tour and Travels website template:
- The Tour and Travels website template app is a simple application that is a collection of destinations, famous places, etc.
- In this app, we created a beautiful home page with attractive user interface.
- we added a poster to the top of the app and added a search destination button by which users can search destinations.
- we also added a featured destination horizontal flatlist at the last of the app where we can add all popular places.
Steps to Create React Native Application:
Step 1: Create a react native application by using this command in the command prompt
React-native init TourApp
Step: 2 We have to download some dependencies which requires for our app. We use navigation in our app to go for one screen to another screen. so, firstly we install the navigation dependencies.
npm install @react-navigation/native
npm install react-native-screens react-native-safe-area-context
npm install @react-navigation/native-stack
Step 3: We will use some Icons in our app . so, we will install dependency for icon
npm i react-native-vector-icons
Project Structure:
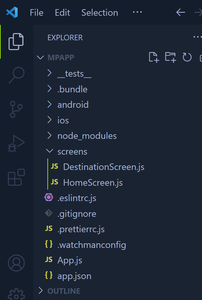
Structure of project
The updated dependencies in package.json file will look like:
"dependencies": {
"expo-constants": "~13.2.4",
"@expo/vector-icons": "^13.0.0",
"react-native-paper": "4.9.2",
"react-native-screens": "~3.15.0",
"@react-navigation/native": "*",
"@react-navigation/native-stack": "*",
"react-native-safe-area-context": "4.3.1"
}
Example: Write the below source code into the App.js file.
Javascript
import React from 'react' ;
import { NavigationContainer } from '@react-navigation/native' ;
import { createStackNavigator } from '@react-navigation/stack' ;
import HomeScreen from './screens/HomeScreen' ;
import DestinationScreen from './screens/DestinationScreen' ;
const Stack = createStackNavigator();
function App() {
return (
<NavigationContainer>
<Stack.Navigator
initialRouteName= "Home"
screenOptions={{
headerStyle: {
backgroundColor: '#3498db' ,
},
headerTintColor: '#fff' ,
headerTitleStyle: {
fontWeight: 'bold' ,
},
}}
>
<Stack.Screen name= "Home" component={HomeScreen} />
<Stack.Screen name= "Destination" component={DestinationScreen} />
</Stack.Navigator>
</NavigationContainer>
);
}
export default App;
|
Javascript
import React from 'react' ;
import { View, Text, Button, StyleSheet,
TextInput, Image, FlatList, TouchableOpacity }
from 'react-native' ;
const featuredDestinations = [
{
id: '1' ,
name: 'Palaces' ,
image:
},
{
id: '2' ,
name: 'Mountain Retreat' ,
image:
},
];
const HomeScreen = ({ navigation }) => {
return (
<View style={styles.container}>
<Image
source={{ uri:
style={styles.image}
/>
<Text style={styles.title}>Welcome to Our Travel App</Text>
<TextInput
style={styles.searchBar}
placeholder= "Search Destinations"
/>
<Button
title= "View Destinations"
onPress={() => navigation.navigate( 'Destination' )}
/>
<Text style={styles.featuredTitle}>Featured Destinations</Text>
<FlatList
data={featuredDestinations}
keyExtractor={(item) => item.id}
horizontal
showsHorizontalScrollIndicator={ false }
renderItem={({ item }) => (
<View style={styles.featuredCard}>
<Image source={{ uri: item.image }}
style={styles.featuredImage} />
<Text style={styles.featuredName}>{item.name}</Text>
</View>
)}
/>
</View>
);
};
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center' ,
alignItems: 'center' ,
padding: 20,
},
image: {
width: 300,
height: 200,
marginBottom: 20,
borderRadius: 10,
},
title: {
fontSize: 24,
fontWeight: 'bold' ,
marginBottom: 10,
},
searchBar: {
width: '100%' ,
height: 40,
borderColor: '#ccc' ,
borderWidth: 1,
borderRadius: 5,
paddingHorizontal: 10,
marginBottom: 20,
},
featuredTitle: {
fontSize: 20,
fontWeight: 'bold' ,
marginTop: 20,
marginBottom: 10,
},
featuredCard: {
marginRight: 10,
borderRadius: 10,
overflow: 'hidden' ,
},
featuredImage: {
width: 150,
height: 100,
borderRadius: 20,
},
featuredName: {
fontSize: 16,
fontWeight: 'bold' ,
marginTop: 5,
},
});
export default HomeScreen;
|
Javascript
import React, { useState, useEffect } from 'react' ;
import { View, Text, StyleSheet, FlatList, TouchableOpacity, Image, ActivityIndicator } from 'react-native' ;
const destinations = [
{
id: '1' ,
name: 'Paris' ,
},
{
id: '2' ,
name: 'New York' ,
},
];
const DestinationScreen = () => {
const [loading, setLoading] = useState( true );
const [destinationTours, setDestinationTours] = useState([]);
const fetchToursData = async (selectedDestination) => {
try {
await new Promise(resolve => setTimeout(resolve, 1000));
setDestinationTours(toursData[selectedDestination] || []);
} catch (error) {
console.error( 'Error fetching tours data:' , error);
} finally {
setLoading( false );
}
};
useEffect(() => {
const defaultDestination = destinations[0].name;
fetchToursData(defaultDestination);
}, []);
return (
<View style={styles.container}>
<Text style={styles.title}>Destinations</Text>
{loading ? (
<ActivityIndicator size= "large" color= "#3498db" />
) : (
<FlatList
data={destinations}
keyExtractor={(item) => item.id}
renderItem={({ item }) => (
<TouchableOpacity
style={styles.destinationCard}
onPress={() => {
fetchToursData(item.name);
}}
>
<Image source={{ uri: item.image }} style={styles.destinationImage} />
<Text style={styles.destinationName}>{item.name}</Text>
</TouchableOpacity>
)}
/>
)}
</View>
);
};
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center' ,
alignItems: 'center' ,
padding: 10,
},
title: {
fontSize: 24,
fontWeight: 'bold' ,
marginBottom: 20,
},
destinationCard: {
marginBottom: 20,
borderRadius: 10,
overflow: 'hidden' ,
elevation: 5,
},
destinationImage: {
width: '250' ,
height: 200,
borderRadius: 10,
},
destinationName: {
fontSize: 18,
fontWeight: 'bold' ,
padding: 10,
backgroundColor: '#3498db' ,
color: '#fff' ,
},
});
export default DestinationScreen;
|
Step to run the Project:
Step 1: Depending on your operating system, type the following command
React-native run-android
React-native run-ios
Output:
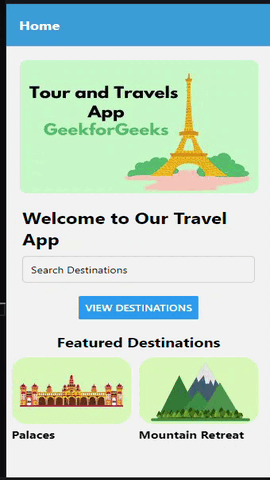
output
Share your thoughts in the comments
Please Login to comment...