Different ways to render a list of items in React
Last Updated :
13 Mar, 2024
This article explains the various approaches for rendering a list of items in React. Render List means displaying various items present in the list. It is a very commonly used operation in applications. React can help in the dynamic rendering of list items.
We will discuss the following approaches to render a list of items in React:
Approach 1: Using Array.map():
- It is an inbuilt javascript function
- It iterates over list of items in array and returns a list of React components
- map() function called over the array and a callback function is accepted as an argument
- callback function will take item and index as argument
- index should be used a key of the item
Syntax:
arrayList.map((item, index) => (
// write your code here
))
Example: Below is the code example using Array.map():
Javascript
// Array.map() function
import React from 'react';
const arrayList = ['List Item 1', 'List Item 2', 'List Item 3'];
const UsingArrayMap = () => (
<div>
{
arrayList.map((item, index) => (
<div key={index}>{item}</div>
))
}
</div>
);
const App = () => (
<div>
<h2>Using Array.map()</h2>
<UsingArrayMap />
</div>
);
export default App;
Steps to Run the App:
npm start
Output:
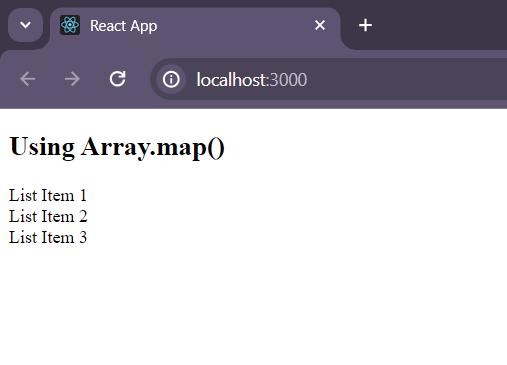
OUTPUT IMAGE OF Array.map() FUNCTION
Approach 2: Using for loop:
- for loop is a control flow statement in Javascript
- It is used to iterate over the items in a array
- In this iterator or loop variable will be used to access the array elements
Syntax:
for (let i = 0; i < arrayList.length; i++) {
// write your code here
}
Example: Below is the example of using for loop:
Javascript
// USING for Loop
import React from 'react';
const arrayList = ['List Item 1', 'List Item 2', 'List Item 3'];
const UsingForLoop = () => {
const arrayListComponents = [];
for (let i = 0; i < arrayList.length; i++) {
arrayListComponents.push(<div key={i}>{arrayList[i]}</div>);
}
return <div>{arrayListComponents}</div>;
};
const App = () => (
<div>
<h2>Using for loop</h2>
<UsingForLoop />
</div>
);
export default App;
Output:
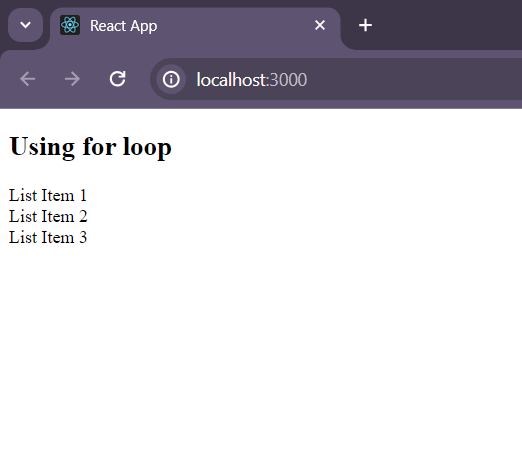
OUTPUT IMAGE – FOR LOOP
Approach 3: Using Array.forEach():
- forEach() function takes a callback function as an argument
- That callback function will be executed for each array item
- callback function takes item and index as argument
Syntax:
arrayList.forEach((item, index) => {
// write your code here
});
Example: Below is the example of using Array.forEach():
Javascript
import React from 'react';
const arrayList =
['List Item 1', 'List Item 2', 'List Item 3'];
const UsingArrayForEach = () => {
const arrayListComponents = [];
arrayList.forEach(
(item, index) => {
arrayListComponents.push(
<div key={index}>
{item}
</div>
);
}
);
return <div>{arrayListComponents}</div>;
};
const App = () => (
<div>
<h2>
Using Array.forEach()
</h2>
<UsingArrayForEach />
</div>
);
export default App;
Output:
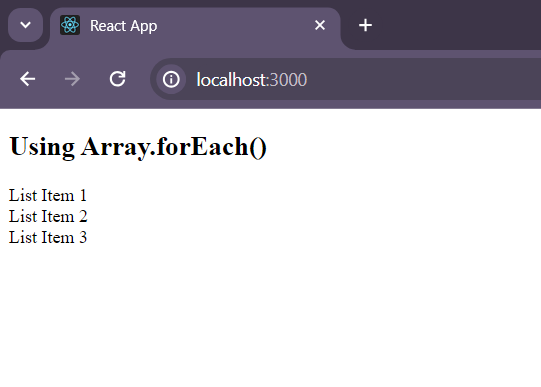
OUTPUT IMAGE – Array.forEach() function
Approach 4: Using React.Children.map():
- It is a utility function in React
- It is used to iterate over the child component
- It takes a callback function as an argument
- The callback functions takes child and index as arguments to iterate over the child components and modify it
Syntax:
React.Children.map(children, (child, index) => (
// write your code here
))
Example: Below is the example of using React.Children.map():
Javascript
import React from 'react';
const arrayList = ['List Item 1', 'List Item 2', 'List Item 3'];
const UsingReactChildrenMap = ({ children }) => {
const modifiedChildren =
React.Children.map(children, (child, index) => (
<div key={index}>Modified {child}</div>
));
return <div>{modifiedChildren}</div>;
};
const App = () => (
<div>
<h2>
Using React.Children.map()
</h2>
<UsingReactChildrenMap>
<div style={
{
display: 'inline-block',
marginRight: '10px'
}}>
{arrayList[0]}
</div>
<div style={
{
display: 'inline-block',
marginRight: '10px'
}}>
{arrayList[1]}
</div>
<div style={
{
display: 'inline-block',
marginRight: '10px'
}}>
{arrayList[2]}
</div>
</UsingReactChildrenMap>
</div>
);
export default App;
Output:
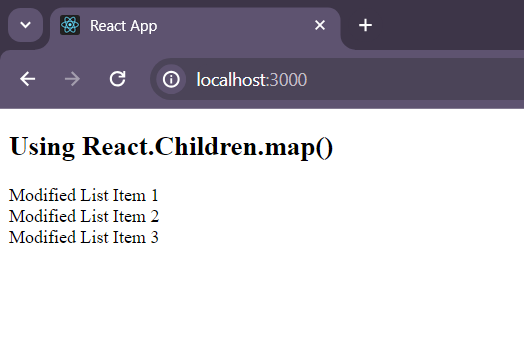
OUTPUT IMAGE – React.Children.map() Method
Approach 5: Using map() and JSX Spread Attributes:
- This method is used to render the components with dynamic props
- In the callback function while rendering the components props will be passed to it
- The callback function take item and index as the argument
Syntax:
const arrayListWithProps = {
prop1: 'value1',
prop2: 'value2',
prop3: 'value3'
};
arrayListWithProps.map(item => (
// write your code here
))
Example: Below is the example of using map() and JSX Spread attributes:
Javascript
import React from 'react';
const araayListWithProps = [
{
id: 1,
name: 'Item 1',
color: 'lightcoral'
},
{
id: 2,
name: 'Item 2',
color: 'cadetblue'
},
{
id: 3,
name: 'Item 3',
color: 'cyan'
}
];
const ArrayListComponent = ({ name, color }) => (
<div style={
{
backgroundColor: color,
padding: '5px',
margin: '5px',
color: 'white'
}
}>
{name}
</div>
);
const App = () => (
<div>
<h2>
Using map() with JSX Spread Props
</h2>
{
araayListWithProps.map(item => (
<ArrayListComponent
key={item.id} {...item} />
))
}
</div>
);
export default App;
Output:
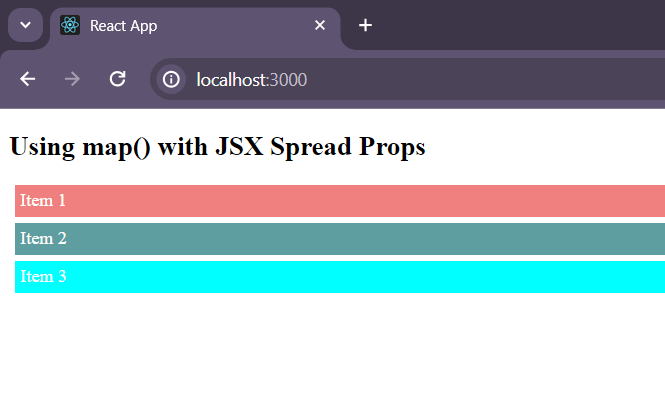
OUTPUT IMAGE FOR map() with JSX props
Share your thoughts in the comments
Please Login to comment...