Why should keys be unique in a React list?
Last Updated :
13 Feb, 2024
In React, keys should be unique within a list to help React efficiently identify and update elements during rendering. Unique keys enable React to distinguish between different list items and optimize re-rendering, ensuring accurate component state management and preventing unexpected behaviour or errors.
Prerequisites:
Steps to Create React Application And Installing Module:
Step 1: Create a React application using the following command:
npx create-react-app foldername
Step 2: After creating your project folder i.e. foldername, move to it using the following command:
cd foldername
Step 3: After setting up react environment on your system, we can start by creating an App.js file and create a directory by the name of components in which we will write our desired function.
Project Structure:
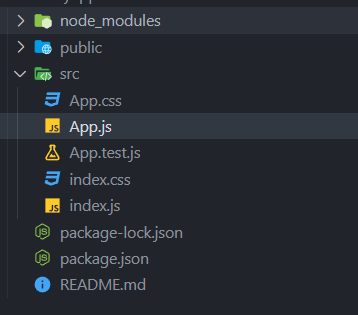
Directory Structure
The updated dependencies in package.json file will look like.
"dependencies": {
"react": "^18.2.0",
"react-dom": "^18.2.0",
"react-router-dom": "^6.22.0",
"react-scripts": "5.0.1",
"web-vitals": "^2.1.4"
}
Importance of Unique Keys in React Lists
- Identification and Efficient Rendering: Unique keys allow React to identify which items in the list have changed, been added, or removed. This identification facilitates efficient updates and re-rendering of the list, minimizing unnecessary changes.
- Optimizing Performance: React can optimize rendering performance with unique keys. Without unique keys, React may re-render more components than necessary, leading to slower performance, especially in complex applications.
- Consistent Component State: Unique keys ensure proper maintenance of component state across renders. Non-unique keys can result in bugs and unpredictable behavior due to incorrect component state management.
Example: When we don’t use the unique key for each element in the list, we get the following error in the console.
Javascript
export default function App() {
const names = [ 'HTML' , 'CSS' , 'JS' , 'React' ];
return (
<main>
<div>
<h2>Name List</h2>
<ul>
{names.map(name => {
return <li>{name}</li>;
})}
</ul>
</div>
</main>
)
}
|
Output:

Error we get when we don’t use the unique key for each element in the LIst.
How to fix this?
To resolve the unique key error in React, follow these steps:
Step 1: Identify the List Rendering Code
Locate the part of your code where you are rendering a list. This is typically where you use the .map() function to iterate over an array and return JSX elements.
Step 2: Ensure Each Element Has a Unique Key
Modify the .map() function to include a unique key prop for each element in the list. The key should be a string that uniquely identifies each list item.
Step 3 : Suppose you have an array of user objects, and each user has a unique ID. Your code might look something like this:
const users = [
{ id: 1, name: 'Alice' },
{ id: 2, name: 'Bob' },
{ id: 3, name: 'Charlie' }
];
function UserList() {
return (
<ul>
{users.map(user => (
<li key={user.id}>{user.name}</li>
))}
</ul>
);
}
Step 4 : Use IDs as Keys If Possible
If your data items have unique IDs, use them as keys. This is the most reliable way to ensure keys are unique.
Step 5: Avoid Using Indexes as Keys If Possible
Only use indexes as keys if there are no unique identifiers and the list is static (i.e., items are not added, removed, or reordered). Using indexes can lead to performance issues and bugs in dynamic lists.
Note: Either we can use indexes as unique key or id as the key.
Example:
Javascript
import React from 'react' ;
function App() {
const users = [
{ id: 1, name: 'Alice' },
{ id: 2, name: 'Bob' },
{ id: 3, name: 'Charlie' }
];
return (
<div>
<h1>User List</h1>
<ul>
{users.map(user => (
<li key={user.id}>{user.name}</li>
))}
</ul>
</div>
);
}
export default App;
|
Then run the application using the following command:
npm start
Output:
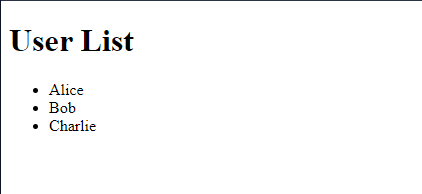
Output of the List.
Conclusion
Using unique keys is crucial for optimizing the rendering process in React, especially in lists. It helps React identify which items have changed, need to be added, or need to be removed, and thus only makes necessary updates. This is not only about avoiding errors or warnings but also about ensuring your application is efficient and bug-free.
Share your thoughts in the comments
Please Login to comment...