List all ways to render a list of items in React
Last Updated :
22 Apr, 2024
In React applications, rendering lists of items is a fundamental task. You will often need to display collections of data, such as product listings, to-do items, or user comments. There is well-established approach that combines JavaScript’s array methods and React’s component structure to achieve this efficiently.
Pre-requisites:
Steps to Create an React Application
Step 1: Create a React application using the following command and navigate to it.
npx create-react-app foldername
Step 2:  After creating your project folder i.e. foldername, move to it using the following command:
cd foldername
Step 2: Install required dependencies.
npm i react react-dom
Updated dependencies in package.json file
"dependencies": {
"react": "^18.2.0",
"react-dom": "^18.2.0",
"react-scripts": "5.0.1",
},
Project Structure:
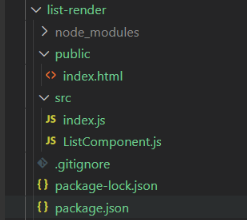
Project Structure
The following are the ways to implement or render list of items using React component.
Common Code: The following “app.js” code will be common for all other codes from where the other codes are included as <ListComponent>
JavaScript
// app.js
import React from 'react';
import ReactDOM from 'react-dom/client';
import ListComponent from './ListComponent';
const root = ReactDOM.createRoot(document.getElementById('root'));
root.render(
<div style={{ display:'flex', flexDirection:'column', alignItems:'center' }}>
<div>
<img src=
'https://media.geeksforgeeks.org/gfg-gg-logo.svg' alt='gfg_logo' />
</div>
<ListComponent />
</div>
);
1. Using Array.map
This approach is the most common and idiomatic method for rendering lists in React. It leverages the map() method available on JavaScript arrays to iterate over each item and generate corresponding JSX elements. Each item in the array is transformed into a React component or element, facilitating the rendering process. This <ListComponent> is included in the above common code and it is run to give the output.
Example:
JavaScript
import React from 'react';
function ListComponent() {
// Sample array of items
const items = ['Apple', 'Banana',
'Orange', 'Papaya', 'Guava',
'Grapes', 'Date'];
// Rendering the list using Array.map()
const itemList = items.map((item, index) => (
<li key={index}>{item}</li>
));
// Rendering the list within an unordered list element
return (
<div>
<h2>Fruit Name</h2>
<ul>{itemList}</ul>
</div>
);
}
export default ListComponent;
Output:
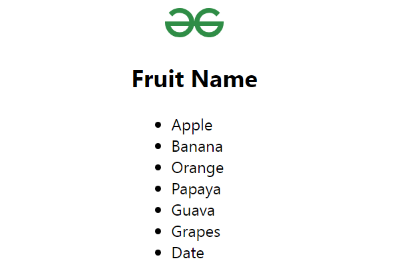
List By Array.map
2. Using a for loop
Using a for loop to render a list in React involves manually iterating over the array of items and creating JSX elements for each item within the loop. This approach provides more control over the rendering process compared to using Array.map() but may result in more verbose code.
Example:
JavaScript
import React from 'react';
function ListComponent() {
// Sample array of items
const items = ['Sunflower', 'Marigold', 'Rose', 'Jasmine', 'Hibiscus'];
// Array to store JSX elements
const itemList = [];
// Using a for loop to iterate over the array of items
for (let i = 0; i < items.length; i++) {
// Creating JSX element for each item and pushing it into the array
itemList.push(<li key={i}>{items[i]}</li>);
}
// Rendering the list within an unordered list element
return (
<div>
<h2>Flower Name</h2>
<ul>{itemList}</ul>
</div>
);
}
export default ListComponent;
Output:
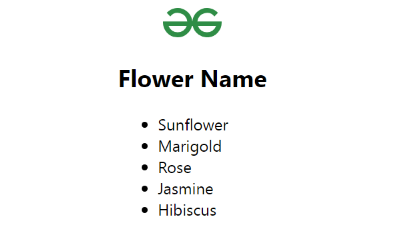
List By For Loop
3. Using a forEach loop
Using a forEach loop to render a list in React involves iterating over the array of items using on JavaScript arrays. Within the loop, JSX elements are created for each item, and these elements can be processed as required, such as pushing them into an array or directly rendering them.
Example:
JavaScript
import React from 'react';
function ListComponent() {
// Sample array of items
const items = ['Tomato', 'Beans',
'Pumpkin', 'Cauliflower',
'Broccoli'];
// Array to store JSX elements
const itemList = [];
// Using a forEach loop to iterate over the array of items
items.forEach((item, index) => {
// Creating JSX element for each item
// and pushing it into the array
itemList.push(<li key={index}>{item}</li>);
});
// Rendering the list within an unordered list element
return (
<div>
<h2>Vegetable Names </h2>
<ul>{itemList}</ul>
</div>
);
}
export default ListComponent;
Output:
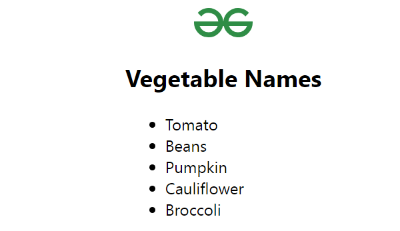
List By For Each Loop
4. Using JSX directly in the render() method
Using JSX directly in the render() method involves embedding JSX elements directly within the return statement of a React component without using any iteration or loop. This approach is suitable for rendering static lists or a small number of items where manually creating JSX elements is feasible.
Include the ListComponent in the above common code.
Example:
JavaScript
import React from 'react';
function ListComponent() {
// Rendering the list directly within the return statement
return (
<div>
<h2>Animal Name </h2>
<ul>
<li>Horse</li>
<li>Ass</li>
<li>Lion</li>
<li>Dog</li>
<li>Wolf</li>
<li>Bear</li>
<li>Tiger</li>
</ul>
</div>
);
}
export default ListComponent;
Output:
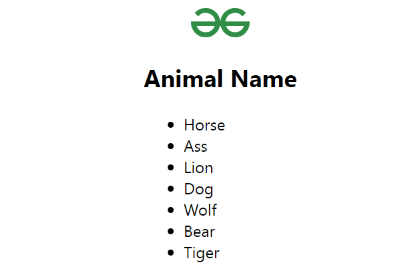
List By Directly JSX
5. Using React.Children.map
Using React.Children.map() with React Fragments allows developers to iterate over children elements within a React Fragment. This approach is useful for rendering multiple elements without introducing an extra DOM element, such as a <div>, as a container. It provides flexibility in organizing and rendering complex UI structures while maintaining a clean and concise JSX syntax.
Include the ListComponent in the above common “App.js” code.
Example:
JavaScript
import React from 'react';
function ListComponent() {
// Sample array of items
const items = ['Item 1', 'Item 2', 'Item 3', 'Item 4', 'Item 5'];
// Rendering the list using React.Children.map() with React Fragments
return (
<div>
<h2>Item List </h2>
{React.Children.map(items, (item, index) => (
<li key={index}>{item}</li>
))}
</div>
);
}
export default ListComponent;
Output:
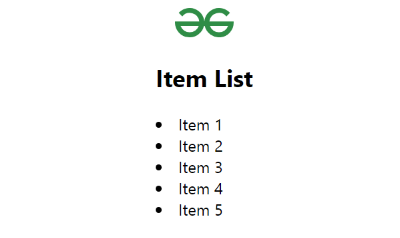
List By React.Children.Map
6. Using a custom component
Creating a custom component in React involves defining a reusable function or class component to encapsulate specific functionality. This custom component can accept props to customize its behavior and appearance. Using custom components enhances code readability, promotes reusability, and simplifies the maintenance of React applications.
Example:
JavaScript
import React from 'react';
// Custom List
function List({ name }) {
return (
<li>{name}</li>
);
}
function ListComponent({ items }) {
const birds = ['Peacock', 'Bird', 'Pigeons',
'Hummingbird', 'Flamingo', 'Crow',
'Eagle', 'Parrot']
return (
<div>
<h2>Bird Name</h2>
<ul>
{birds.map((name, index) => (
<List name={name} key={index} />
))}
</ul>
</div>
);
}
export default ListComponent
Output:
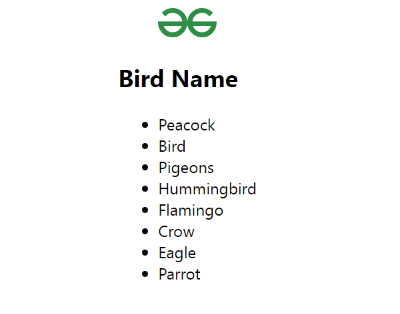
List By Custom Component
Share your thoughts in the comments
Please Login to comment...