Create Dynamic Table with Auto Increment Serial Number on Button Click Event
Last Updated :
13 Mar, 2024
We will see how we can auto increment the serial number of a table in html using JavaScript. We will build a button clicking on that will add a row to a table with an increasing number of rows. It will show the dynamic behavior, showing that we will use JavaScript and jQuery.
These are the following methods:
Using JavaScript
This approach dynamically adds rows to an HTML table using JavaScript. Upon clicking the “Add row” button, a new row is inserted into the table’s tbody. The serial number for each row is auto-incremented, ensuring uniqueness. The JavaScript function maintains a counter to track the serial numbers. Additionally, input fields for name and email are added to each row. Basic CSS styles are applied to maintain table structure. This method showcases efficient DOM manipulation for interactive table creation.
Example: This example shows the implementation of the above-explained approach.
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width,
initial-scale=1.0">
<title>Document</title>
</head>
<style>
table {
border-collapse: collapse;
width: 100%;
}
th,
td {
border: 1px solid #ddd;
padding: 8px;
text-align: left;
}
th {
background-color: #f2f2f2;
}
input[type="text"] {
border: none;
background: yellow;
}
</style>
<body>
<button onclick="addRow()"> Add row</button>
<table id="dynamicTable">
<thead>
<tr>
<th>Serial number</th>
<th>Name</th>
<th>Email</th>
</tr>
</thead>
<tbody>
</tbody>
</table>
<script>
let idCounter = 1;
function addRow() {
let table = document.getElementById("dynamicTable")
.getElementsByTagName('tbody')[0];
let newRow = table.insertRow();
let cell1 = newRow.insertCell(0);
let cell2 = newRow.insertCell(1);
let cell3 = newRow.insertCell(2);
cell1.textContent = idCounter++;
cell2.innerHTML = '<input type=\"text\" name=\"name[]\">';
cell3.innerHTML = '<input type=\"text\" name=\"email[]\">';
}
</script>
</body>
</html>
Ouptut:
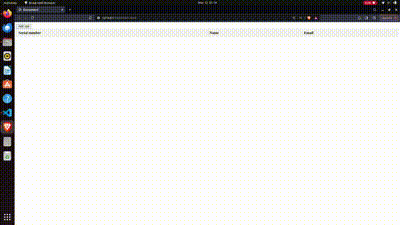
output gif
Using JQuery
This approach utilizes jQuery to dynamically add rows to an HTML table. When the “Click to add row” button is clicked, a new row is appended to the table’s tbody. The serial number increments with each addition, ensuring unique identifiers for each row. Input fields for name and email are included in each row. The jQuery append() function simplifies DOM manipulation, reducing the need for verbose JavaScript code. Basic CSS styles are applied to enhance table appearance. Overall, jQuery streamlines the process of dynamic table creation by simplifying the addition of rows and handling DOM manipulation efficiently.
Example: This example shows the implementation of the above-explained approach.
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width,
initial-scale=1.0">
<script src=
"https://code.jquery.com/jquery-3.7.1.min.js"
integrity=
"sha256-/JqT3SQfawRcv/BIHPThkBvs0OEvtFFmqPF/lYI/Cxo="
crossorigin="anonymous"></script>
<title>Document</title>
</head>
<style>
table {
border-collapse: collapse;
width: 100%;
}
th,
td {
border: 1px solid #ddd;
padding: 8px;
text-align: left;
}
th {
background-color: #f2f2f2;
}
input[type="text"] {
border: none;
background: yellow;
}
</style>
<body>
<button id="add"> Add row</button>
<table id="tab">
<thead>
<tr>
<th>Serial number</th>
<th>Name</th>
<th>Email</th>
</tr>
</thead>
<tbody>
</tbody>
</table>
<script>
$(document).ready(function () {
let rowNum = 1;
$("#add").click(function () {
$("#tab tbody").append("<tr><td>" +
rowNum++ +
"</td><td><input placeholder=name/></td><td><input placeholder=email/></td></tr>");
});
});
</script>
</body>
</html>
Ouptut:
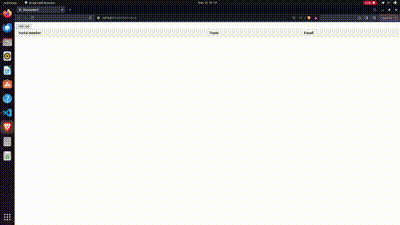
Output
Share your thoughts in the comments
Please Login to comment...