Create Contact Form Template in Tailwind CSS
Last Updated :
27 Mar, 2024
A Contact Form Template is a pre-designed layout that simplifies the process of creating a contact form for a website. It typically includes input fields for the user’s name, email address, subject, and message, along with a submit button. The form is styled using Tailwind CSS, a utility-first CSS framework, which allows for easy customization and a modern, responsive design.
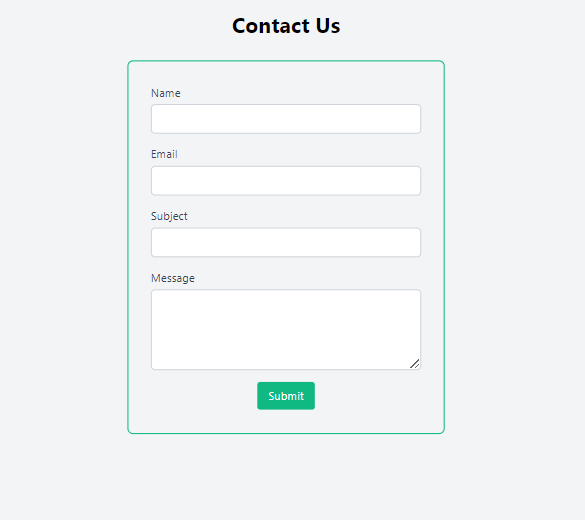
Approach:
- Begin with a standard HTML5 structure, including the
<!DOCTYPE html>
declaration, <html>
, <head>
, and <body>
tags. Import the Tailwind CSS library for styling. - Inside the container, include a title for the contact form and a form element and inside the form, include input fields for the user’s name, email, subject, and message. Use Tailwind CSS classes for styling. Set the “required” attribute on each input field to make them mandatory.
- Add a submit button inside the form with Tailwind CSS classes for styling. This button will trigger the form submission when clicked.
- Use JavaScript to handle form validation. Add an event listener to the form’s “submit” event that calls a function to validate the form inputs. Inside this function, retrieve the values from the input fields and check if they are empty. If any field is empty, display an alert message and return false to prevent form submission.
- Use a regular expression to validate the email address format. If the email address is not in the correct format, display an alert message and return false to prevent form submission.
- Use Tailwind CSS classes to style the container, form, input fields, submit button, and alert messages. Customize the colors, fonts, and layout to create an appealing visual design for the contact form.
- If all validations pass, display an alert and submit the form.
Example: The below example illustrates the implementation to Create Contact Form Template in Tailwind CSS.
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Contact Us</title>
<link href="https://cdn.jsdelivr.net/npm/tailwindcss@2.2.19/dist/tailwind.min.css" rel="stylesheet">
</head>
<body class="bg-gray-100">
<section id="contact" class="py-12 bg-gray-100 flex justify-center items-center">
<div class="container mx-auto px-4 w-full md:w-2/3 lg:w-1/2">
<div class="bg-white shadow-md rounded border-2 border-solid border-green-600 rounded-lg p-5 w-3/4">
<h3 class="text-3xl mb-8 text-green-400 p-4 justify-center">Contact Us</h3>
<form id="contactForm" action="#" method="POST" class="grid grid-cols-1 sm:grid-cols-2 gap-6">
<div>
<label for="name" class="block text-gray-700 font-semibold mb-2">Name</label>
<input type="text" id="name" name="name" placeholder="Enter your name"
class="w-full border border-gray-300 rounded-md px-4 py-2 focus:outline-none focus:ring-blue-500 focus:border-blue-500">
<div id="nameValidation" class="hidden text-red-600 text-sm">Please enter your name.</div>
</div>
<div>
<label for="email" class="block text-gray-700 font-semibold mb-2">Email</label>
<input type="email" id="email" name="email" placeholder="Enter your email"
class="w-full border border-gray-300 rounded-md px-4 py-2 focus:outline-none focus:ring-blue-500 focus:border-blue-500">
<div id="emailValidation" class="hidden text-red-600 text-sm">Please enter a valid email address.</div>
</div>
<div class="sm:col-span-2">
<label for="subject" class="block text-gray-700 font-semibold mb-2">Subject</label>
<input type="text" id="subject" name="subject" placeholder="Enter the subject"
class="w-full border border-gray-300 rounded-md px-4 py-2 focus:outline-none focus:ring-blue-500 focus:border-blue-500">
<div id="subjectValidation" class="hidden text-red-600 text-sm">Please enter the subject.</div>
</div>
<div class="sm:col-span-2">
<label for="message" class="block text-gray-700 font-semibold mb-2">Message</label>
<textarea id="message" name="message" placeholder="Enter your message" rows="5"
class="w-full border border-gray-300 rounded-md px-4 py-2 focus:outline-none focus:ring-blue-500 focus:border-blue-500"></textarea>
<div id="messageValidation" class="hidden text-red-600 text-sm">Please enter your message.</div>
</div>
<div class="sm:col-span-2">
<button type="submit"
class="w-full bg-green-500 text-white font-semibold py-2 px-4 rounded-md hover:bg-green-600 transition duration-300">Submit</button>
</div>
</form>
</div>
</div>
</section>
<script>
let contactForm = document.getElementById('contactForm');
let nameInput = document.getElementById('name');
let nameValidation = document.getElementById('nameValidation');
let emailInput = document.getElementById('email');
let emailValidation = document.getElementById('emailValidation');
let subjectInput = document.getElementById('subject');
let subjectValidation = document.getElementById('subjectValidation');
let messageInput = document.getElementById('message');
let messageValidation = document.getElementById('messageValidation');
const validateInput = (input, validationElement, validationFunction) => {
if (!validationFunction(input.value.trim())) {
validationElement.classList.remove('hidden');
return false;
} else {
validationElement.classList.add('hidden');
return true;
}
};
const validateName = () => validateInput(nameInput, nameValidation, (name) => name !== '');
const validateEmail = () => validateInput(emailInput, emailValidation, (email) => /^[^\s@]+@[^\s@]+\.[^\s@]+$/.test(email));
const validateSubject = () => validateInput(subjectInput, subjectValidation, (subject) => subject !== '');
const validateMessage = () => validateInput(messageInput, messageValidation, (message) => message !== '');
const validateForm = () => {
return validateName() && validateEmail() && validateSubject() && validateMessage();
};
contactForm.addEventListener('submit', function (event) {
event.preventDefault();
if (validateForm()) {
alert('Form submitted successfully!');
this.submit();
}
});
const inputs = [nameInput, emailInput, subjectInput, messageInput];
inputs.forEach(input => {
input.addEventListener('input', function () {
switch (input.id) {
case 'name':
validateName();
break;
case 'email':
validateEmail();
break;
case 'subject':
validateSubject();
break;
case 'message':
validateMessage();
break;
default:
break;
}
});
});
</script>
</body>
</html>
Output:
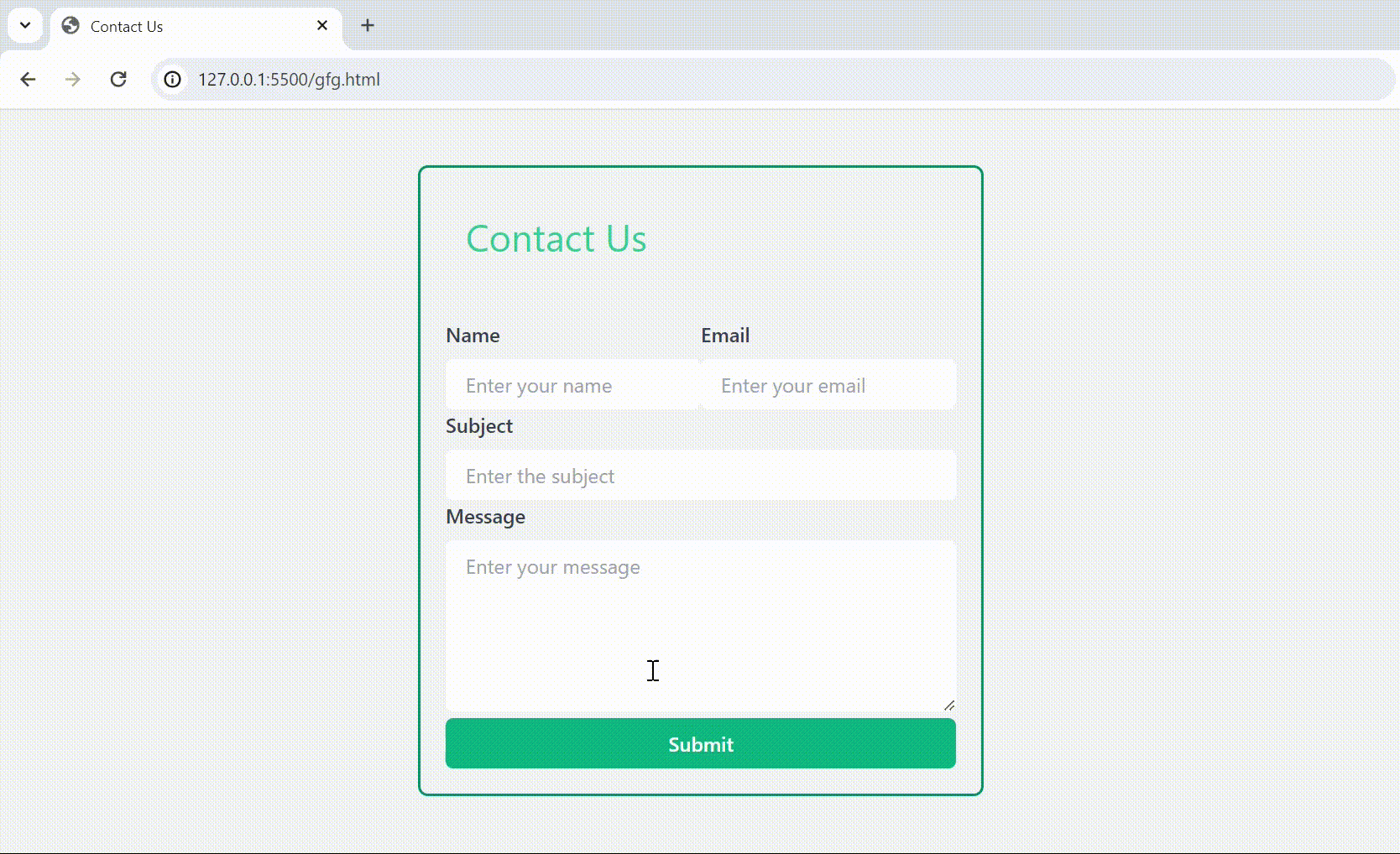
Output : Validate all the fields
Share your thoughts in the comments
Please Login to comment...