Contact Us Page using ReactJS and Tailwind
Last Updated :
11 Mar, 2024
The Contact Us page is crucial for user interaction, allowing users to connect with the website owner or customer support, submit feedback, and suggestions, and access departmental contact information.
Preview of final output: Let us have a look at how the final output will look like.
.jpg)
Prerequisites:
Basic features of Contact Us Page:
- Address of the Organisation: Usually represented in a card and contains the basic information to reach the organisation
- Contact Information: Information such as email address and phone number is shown in this block
- Additional Information: It contains the contact information of other departments of the organisation
- Feedback Form: A feedback form using which the users can submit their suggestions and queries
- Map: A map using which user can be provided the physical location of the organisation
- Social Media Links: Display social media pages link of the organisation
Approach to create a Contact Us page:
- Set up a basic react project and install the required dependencies.
- Create the basic layout consisting of a Navbar and Welcome slide.
- Style the components using Tailwind.
- Add fixed icons to provide link to social media pages of the organisation
Steps to Create React Application And Installing Module:
Step 1: Set up the project using the command
npx create-react-app <<Project_Name>>
Step 2: Navigate to the folder using the command
cd <<Project_Name>>
Step 3: Install the required dependencies using the command
npm install -D tailwindcss
Step 4: Create the tailwind config file using the command
npx tailwindcss init
Step 5: Rewrite the tailwind.config.js file as follows
/** @type {import('tailwindcss').Config} */
module.exports = {
content: [
"./src/**/*.{js,jsx,ts,tsx}",
],
theme: {
extend: {},
},
plugins: [],
}
Project Structure:
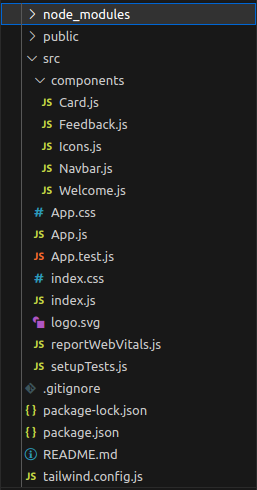
The updated dependencies in package.json file will look like:
"dependencies": {
"@testing-library/jest-dom": "^5.17.0",
"@testing-library/react": "^13.4.0",
"@testing-library/user-event": "^13.5.0",
"react": "^18.2.0",
"react-dom": "^18.2.0",
"react-scripts": "5.0.1",
"web-vitals": "^2.1.4"
}
"devDependencies": {
"tailwindcss": "^3.3.3",
}
Example: Add the following code in respective files:
- App.js: This file imports all the component and displays map on the page
- Card.js: Two cards providing details to contact are shown using this component
- Feedback.js: A form to submit user queries is displayed in this component
- Icons.js: The link to social media pages is displayed.
- Navbar.js: Navgiation is provided using this component
- Welcome.js: A welcome slide is displayed in this component
CSS
@tailwind base;
@tailwind components;
@tailwind utilities;
body {
margin : 0 ;
font-family : -apple-system, BlinkMacSystemFont, 'Segoe UI' , 'Roboto' , 'Oxygen' ,
'Ubuntu' , 'Cantarell' , 'Fira Sans' , 'Droid Sans' , 'Helvetica Neue' ,
sans-serif ;
-webkit-font-smoothing: antialiased;
-moz-osx-font-smoothing: grayscale;
height : 100 vh;
}
code {
font-family : source-code-pro, Menlo, Monaco, Consolas, 'Courier New' ,
monospace ;
}
|
Javascript
import './App.css' ;
import Icons from './components/Icons' ;
import Welcome from './components/Welcome' ;
import Card from './components/Card' ;
import Feedback from './components/Feedback' ;
function App() {
return (
<div >
<Welcome/>
<Icons/>
<Card/>
<Feedback/>
<img src=
style={{marginTop: "14%" , marginLeft: "44%" }}/>
</div>
);
}
export default App;
|
Javascript
export default function Card() {
return (
<div >
<div className= "h-52 ml-48 float-left
-mt-10 w-96 flex-col
rounded-xl bg-white bg-clip-border
text-gray-700 shadow-2xl" >
<div className= "p-6" >
<h5 className= "text-center mr-4 mb-2
block font-sans text-xl
font-semibold text-blue-gray-900
antialiased" >
Reach Us At
</h5>
<ul>
<li className= "mt-2" >
<span><i className= "fa fa-phone mr-2" ></i> </span>
+91-9998887776
</li>
<li className= "mt-2" >
<span><i className= "fa fa-envelope mr-2" ></i> </span>
<span>feedback@geeksforgeeks.org</span>
</li>
<li className= "mt-2" >
<span><i className= "fa-solid fa-map-pin mr-2" ></i>
</span>
A-143, 9th Floor, Sovereign Corporate
<span className= "pl-4" >
Tower, Sector-136, Noida, Uttar Pradesh
</span>
</li>
</ul>
</div>
</div>
<div class= " mr-36 w-96 text-center float-right
-mt-11 w-1/3 flex-col rounded-xl
bg-white text-gray-700 shadow-2xl" >
<div className= "p-6 " >
<h5 className= "mb-2 block font-sans
text-xl font-semibold
text-blue-gray-900 antialiased" >
Branding & Collaboration
</h5>
<i className= "fa fa-handshake fa-2xl" ></i>
<div className= "text-left mt-4" >
<span><i className= "fa fa-envelope mr-2" ></i> </span>
<span>branding@geeksforgeeks.org</span>
</div>
<div className= "mt-2 text-left" >
<span><i className= "fa-solid fa-map-pin mr-2" ></i> </span>
A-143, 9th Floor, Sovereign Corporate
Tower, Sector-<span className= "pl-5" >136,
Noida, Uttar Pradesh</span>
</div>
</div>
</div>
</div>
)
}
|
Javascript
export default function Feedback() {
return (
<div className= "absolute mt-52 ml-48
w-80 float-left border-2 p-2
rounded-xl shadow-xl text-xl" >
<form>
<p className= "text-2xl" >Feedback & Queries</p>
<div>
<label className= "text-sm" >Select Issue*</label>
<br></br>
<select className= "bg-gray-50 border border-gray-300
text-gray-600 text-sm rounded-lg
focus:border-blue-500 w-full p-2.5" >
<option value= "Feedback" >
-- Select Your Query --
</option>
<option value= "Feedback" >
Feedback
</option>
<option value= "Feedback" >
Course Related Queries
</option>
<option value= "Feedback" >
Payment Related Issue
</option>
<option value= "Feedback" >
Hiring Related Queries
</option>
<option value= "Feedback" >
Advertise With Us
</option>
</select>
<br></br>
<label className= "text-sm" >Email Address*</label>
<br></br>
<input className= "bg-gray-50 border border-gray-300
text-sm rounded-lg focus:border-blue-500
w-full p-2.5"
type= "email"
placeholder= "abc@geeksforgeeks.org" />
<br></br>
<label className= "text-sm" >Contact No.</label>
<br></br>
<input className= "bg-gray-50 border border-gray-300
text-sm rounded-lg focus:border-blue-500
w-full p-2.5"
type= "text"
placeholder= "1324567890" />
<br></br>
<label className= "text-sm" >
Drop Your Query
</label>
<br></br>
<textarea className= "bg-gray-50 border border-gray-300
text-sm rounded-lg
focus:border-blue-500
w-full p-2.5"
rows= "4"
cols= "25"
maxlength= "300"
placeholder= "Max Allowed Characters: 300" >
</textarea>
<br></br>
<button className= "bg-blue-500 hover:bg-blue-700
text-white font-bold
py-2 px-4 rounded"
type= "button" >
Submit
</button>
</div>
</form>
</div>
)
}
|
Javascript
export default function Icons() {
return (
<div className= "fixed left-0 top-2/4 z-50
bg-transparent flex flex-col space-y-3" >
<button
type= "button"
data-te-ripple-init
data-te-ripple-color= "light"
className= "flex px-4
py-1.5 text-xs font-medium
uppercase leading-normal text-white
rounded-r-xl "
style={{ backgroundColor: "#1da1f2" }}>
<svg
className= "mr-2 h-3.5 w-3.5"
fill= "currentColor"
viewBox= "0 0 24 24" >
<path d=
M24 4.557c-.883.392-1.832.656-2.828.775 1.017-.609 1.798-1.574 2.165-2.724-.951.564-2.005.974-3.127 1.195-.897-.957-2.178-1.555-3.594-1.555-3.179 0-5.515 2.966-4.797 6.045-4.091-.205-7.719-2.165-10.148-5.144-1.29 2.213-.669 5.108 1.523 6.574-.806-.026-1.566-.247-2.229-.616-.054 2.281 1.581 4.415 3.949 4.89-.693.188-1.452.232-2.224.084.626 1.956 2.444 3.379 4.6 3.419-2.07 1.623-4.678 2.348-7.29 2.04 2.179 1.397 4.768 2.212 7.548 2.212 9.142 0 14.307-7.721 13.995-14.646.962-.695 1.797-1.562 2.457-2.549z " />
</svg>
Twitter
</button>
<button
type=" button "
data-te-ripple-init
data-te-ripple-color=" light "
className=" flex px-2
py-1.5 text-xs font-medium
uppercase leading-normal text-white
rounded-r-xl "
style={{ backgroundColor: " #1877f2" }}>
<svg
xmlns="http:
className="mr-2 h-3.5 w-3.5 "
fill=" currentColor "
viewBox=" 0 0 24 24 ">
<path d=
" M9 8h-3v4h3v12h5v-12h3.642l.358-4h-4v-1.667c0-.955.192-1.333 1.115-1.333h2.885v-5h-3.808c-3.596 0-5.192 1.583-5.192 4.615v3.385z " />
</svg>
Facebook
</button>
<button
type=" button "
data-te-ripple-init
data-te-ripple-color=" light "
className=" flex px-4
py-1.5 text-xs font-medium
uppercase leading-normal text-white
rounded-r-xl "
style={{ backgroundColor: " #c13584" }}>
<svg
xmlns="http:
className="mr-2 h-3.5 w-3.5 "
fill=" currentColor "
viewBox=" 0 0 24 24 ">
<path d=
" M12 2.163c3.204 0 3.584.012 4.85.07 3.252.148 4.771 1.691 4.919 4.919.058 1.265.069 1.645.069 4.849 0 3.205-.012 3.584-.069 4.849-.149 3.225-1.664 4.771-4.919 4.919-1.266.058-1.644.07-4.85.07-3.204 0-3.584-.012-4.849-.07-3.26-.149-4.771-1.699-4.919-4.92-.058-1.265-.07-1.644-.07-4.849 0-3.204.013-3.583.07-4.849.149-3.227 1.664-4.771 4.919-4.919 1.266-.057 1.645-.069 4.849-.069zm0-2.163c-3.259 0-3.667.014-4.947.072-4.358.2-6.78 2.618-6.98 6.98-.059 1.281-.073 1.689-.073 4.948 0 3.259.014 3.668.072 4.948.2 4.358 2.618 6.78 6.98 6.98 1.281.058 1.689.072 4.948.072 3.259 0 3.668-.014 4.948-.072 4.354-.2 6.782-2.618 6.979-6.98.059-1.28.073-1.689.073-4.948 0-3.259-.014-3.667-.072-4.947-.196-4.354-2.617-6.78-6.979-6.98-1.281-.059-1.69-.073-4.949-.073zm0 5.838c-3.403 0-6.162 2.759-6.162 6.162s2.759 6.163 6.162 6.163 6.162-2.759 6.162-6.163c0-3.403-2.759-6.162-6.162-6.162zm0 10.162c-2.209 0-4-1.79-4-4 0-2.209 1.791-4 4-4s4 1.791 4 4c0 2.21-1.791 4-4 4zm6.406-11.845c-.796 0-1.441.645-1.441 1.44s.645 1.44 1.441 1.44c.795 0 1.439-.645 1.439-1.44s-.644-1.44-1.439-1.44z " />
</svg>
Instagram
</button>
<button
type=" button "
data-te-ripple-init
data-te-ripple-color=" light "
className=" flex px-4
py-1.5 text-xs font-medium
uppercase leading-normal text-white
rounded-r-xl "
style={{backgroundColor: " #ff0000"}}>
<svg
xmlns="http:
className="mr-2 mt-0.5 h-4.5 w-3.5 "
fill=" currentColor "
viewBox=" 0 0 24 24 ">
<path d=
" M19.615 3.184c-3.604-.246-11.631-.245-15.23 0-3.897.266-4.356 2.62-4.385 8.816.029 6.185.484 8.549 4.385 8.816 3.6.245 11.626.246 15.23 0 3.897-.266 4.356-2.62 4.385-8.816-.029-6.185-.484-8.549-4.385-8.816zm-10.615 12.816v-8l8 3.993-8 4.007z" />
</svg>
Youtube
</button>
</div>
)
}
|
Javascript
export default function Navbar() {
return (
<div>
<nav classNameName= "fixed w-full
z-20 top-0 left-0" >
<div className= "flex flex-wrap items-center
justify-between mx-auto p-4" >
className= "flex items-center" >
<img src=
className= "mr-2"
alt= "GFG Logo" />
<span className= "self-center text-2xl font-semibold " >
GeeksforGeeks
</span>
</a>
<div className= "items-center justify-between hidden
w-full md:flex md:w-auto md:order-1"
id= "navbar-sticky" >
<ul className= "flex flex-col p-4
md:p-0 font-medium
md:flex-row md:space-x-8" >
<li>
<a href= "#"
className= "block py-2 pl-3
pr-4 text-white bg-blue-700
rounded md:bg-transparent
md:text-blue-700 md:p-0" >
Home
</a>
</li>
<li>
<a href= "#"
className= "block py-2 pl-3
pr-4 text-gray-900 rounded
hover:bg-gray-100
md:hover:bg-transparent
md:hover:text-blue-700 md:p-0" >
Posts
</a>
</li>
<li>
<a href= "#"
className= "block py-2 pl-3
pr-4 text-gray-900 rounded
hover:bg-gray-100
md:hover:bg-transparent
md:hover:text-blue-700 md:p-0" >
About us
</a>
</li>
</ul>
</div>
</div>
</nav>
</div>
)
}
|
Javascript
import Navbar from "./Navbar" ;
export default function Welcome(){
return (
<div className= "h-48 bg-gray-200 px-24" >
<Navbar/>
<h1 className= "pt-4 text-center text-slate
font-semibold text-3xl" >
GeeksforGeeks Contact US Page
</h1>
</div>
)
}
|
Steps to run the application:
Step 1: Type the following command in terminal
npm run start
Output:
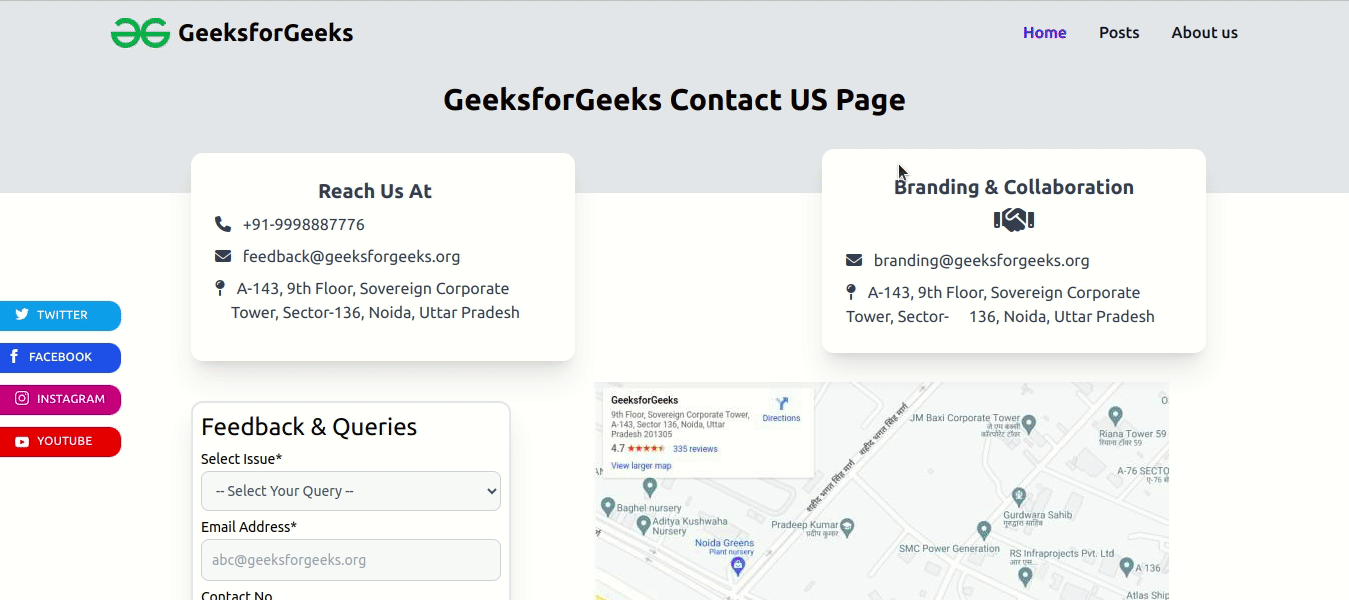
Share your thoughts in the comments
Please Login to comment...