Create a Chessboard Pattern in React.js
Last Updated :
24 Nov, 2023
In this article, we will create a chessboard pattern that can be created in React JS by using a 2-Dimensional array and mapping over it to display the chessboard pattern.
Prerequisite:
Steps to Create the React Application And Installing Module:
Step 1: You will start a new project using create-react-app so open your terminal and type.
npx create-react-app chessboard-react
Step 2: Switch to the chessboard-react folder using the following command.
cd chessboard-react
Project Structure:
.jpg)
Project structure
The updated dependencies in package.json file will look like:
"dependencies": {
"react": "^18.2.0",
"react-dom": "^18.2.0",
"react-scripts": "5.0.1",
"web-vitals": "^2.1.4",
}
Approach:
- We will Create a 2-dimensional array of size N*M(here we will take N and M both as 8). Now we will call the map Array method of javascript over each of the rows of the 2-dimensional array, inside each of the callback function of the map method for each of the rows, call again the map Array method(as each row contains M columns).
- Inside the callback function of this 2nd map, the method displays a div element. By doing so a N*M(total 64 as N and M both here are 8) cells will be created where N is the number of rows and M is the number of columns.
- Also, the index of each row will be represented by rIndex and each column’s index by cIndex. Also, we need to color the cells with an appropriate color.
- If cIndex+rIndex is an even number then the cell has to be colored black else it has to be colored white. This will create cells of alternative colors of white and black.
Example: Now let’s see how to create a chessboard in react.js
Javascript
import "./App.css" ;
import { useEffect, useState } from "react" ;
export default function App() {
const n = 8;
const m = 8;
const [chessBoard, setChessBoard] = useState([]);
useEffect(() => {
const result = [];
for (let i = 0; i < n; i++) {
const row = Array.from({ length: m });
result.push(row);
}
setChessBoard(result);
}, []);
return (
<>
{chessBoard.length > 0 &&
chessBoard.map((row, rIndex) => {
return (
<div className= "row" key={rIndex}>
{row.map((_, cIndex) => {
return (
<div
className={`box ${
(rIndex + cIndex) % 2 === 0
? "black" : "white"
}`}
key={cIndex}
></div>
);
})}
</div>
);
})}
</>
);
}
|
CSS
.row {
display : flex;
}
.box {
width : 50px ;
height : 50px ;
}
. black {
background-color : black ;
}
. white {
background-color : ghostwhite;
}
|
Step to Run Application: Run the application using the following command from the root directory of the project:
npm start
Output:
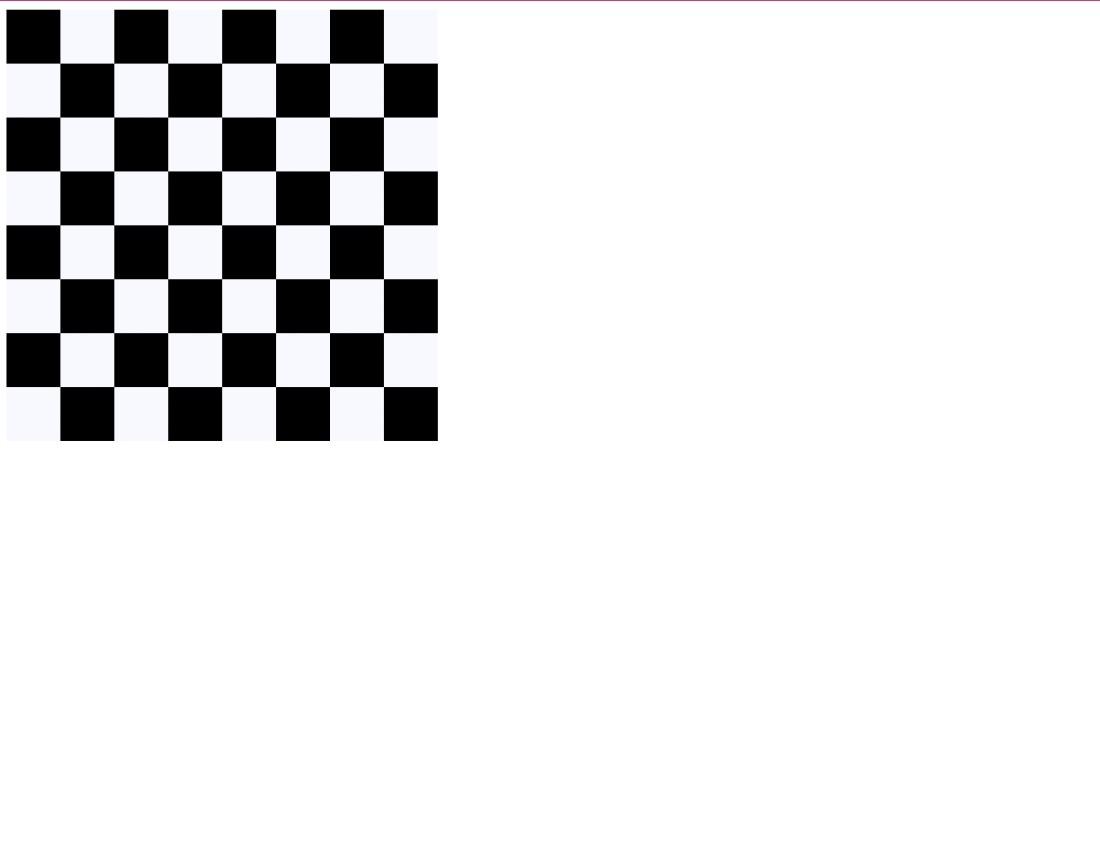
Output
Share your thoughts in the comments
Please Login to comment...