Creating a Chessboard Pattern with Bootstrap & jQuery
Last Updated :
27 Feb, 2024
A chessboard pattern can be created very easily using jQuery. Using jQuery, you can create a chessboard with any number of rows and columns you desire by just tweaking a few parameters. Also, the lines of code written using this method will be way less than creating the same using pure HTML and Bootstrap especially when the number of rows and columns is very large.
Prerequisites
Approach
The application creates a simple chessboard using HTML, CSS, Bootstrap, and jQuery. It dynamically generates an 8×8 grid representing the chessboard, with alternating black and white cells. Each cell is styled using CSS to resemble a chessboard square. Bootstrap is utilized for layout and styling, providing responsiveness and a modern look. jQuery is employed to efficiently manipulate the DOM, dynamically adding the cells to the table. The result is a visually appealing and functional chessboard suitable for display in web applications or games. This application serves as a basic demonstration of using web technologies to create interactive and visually appealing interfaces.
Example: This example shows the implementation of the above-explained approach.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport" content=" width = device -width,
initial-scale = 1 .0">
< title >Chess board</ title >
< link href =
< style >
.cell {
height: 30px;
width: 30px;
border: 1.5px solid grey;
border-style: inset;
}
.blackcell {
background-color: black;
}
.whitecell {
background-color: white;
}
</ style >
</ head >
< body >
< div class = "container text-center" >
< h1 class = "text-success" >GeeksforGeeks</ h1 >
< br >
< div class = "row justify-content-center" >
< div class = "col-auto" >
< table id = "chessboard" ></ table >
</ div >
</ div >
</ div >
< script src =
< script src =
< script >
$(document).ready(function () {
let chessBoard = $('< table ></ table >');
for (let i = 0; i < 8 ; i++) {
let row = $('<tr></ tr >');
for (let j = 0; j < 8 ; j++) {
let cell = $('<td class = "cell" ></ td >');
if ((i + j) % 2 == 0) {
cell.addClass('whitecell');
} else {
cell.addClass('blackcell');
}
row.append(cell);
}
chessBoard.append(row);
}
$('#chessboard').append(chessBoard);
});
</ script >
</ body >
</ html >
|
Output:
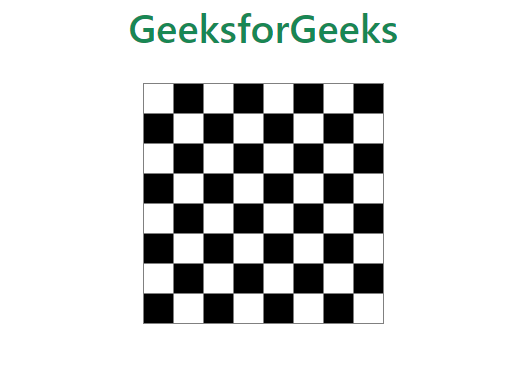
Share your thoughts in the comments
Please Login to comment...