Create a Button Group using HTML and CSS
Last Updated :
29 Nov, 2023
This article will show you how to create a Button Group with the help of HTML and CSS. To create the Button Group, first, we create buttons using the HTML <button> element and then apply some CSS styles to make all buttons in a group.
First, we create a div container with the class name .button-group that contains all buttons. Then we apply CSS styles, like – padding, margin, background, etc to make the button group.
Example: Here, we will create a Horizontal Button Group.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport" content =
"width=device-width, initial-scale=1.0" >
< title >Button Group</ title >
< style >
body {
display: flex;
justify-content: center;
align-items: center;
}
.button-group .button {
padding: 10px 20px;
border: 1px solid black;
background-color: #136a13;
color: #fff;
text-align: center;
text-decoration: none;
font-size: 16px;
cursor: pointer;
float: left;
}
.button-group .button:not(:last-child) {
border-right: none;
}
.button:hover {
background-color: #2980b9;
}
</ style >
</ head >
< body >
< div class = "button-group" >
< button class = "button" >HTML</ button >
< button class = "button" >CSS</ button >
< button class = "button" >JavaScript</ button >
< button class = "button" >Bootstrap</ button >
</ div >
</ body >
</ html >
|
Output:

Example 2: Here, we will create a Vertical Button Group.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport" content =
"width=device-width, initial-scale=1.0" >
< title >Vertical Button Group</ title >
< style >
body {
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
margin: 0;
}
.button-group {
display: flex;
flex-direction: column;
align-items: center;
}
.button-group .button {
margin: 0;
width: 150px;
padding: 10px;
border: 1px solid black;
background-color: #136a13;
color: #fff;
text-align: center;
text-decoration: none;
font-size: 16px;
cursor: pointer;
}
.button-group .button:not(:last-child) {
border-bottom: none;
}
.button-group .button:hover {
background-color: #2980b9;
}
</ style >
</ head >
< body >
< div class = "button-group" >
< button class = "button" >HTML</ button >
< button class = "button" >CSS</ button >
< button class = "button" >JavaScript</ button >
< button class = "button" >Bootstrap</ button >
</ div >
</ body >
</ html >
|
Output:
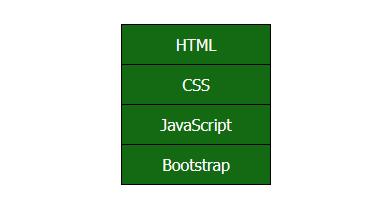
Share your thoughts in the comments
Please Login to comment...