Bootstrap is a free, open-source, potent CSS framework and toolkit used to create modern and responsive websites and web applications. It is the most popular HTML, CSS, and JavaScript framework for developing responsive, mobile-first websites. Nowadays, websites are perfect for all browsers and all sizes of screens.
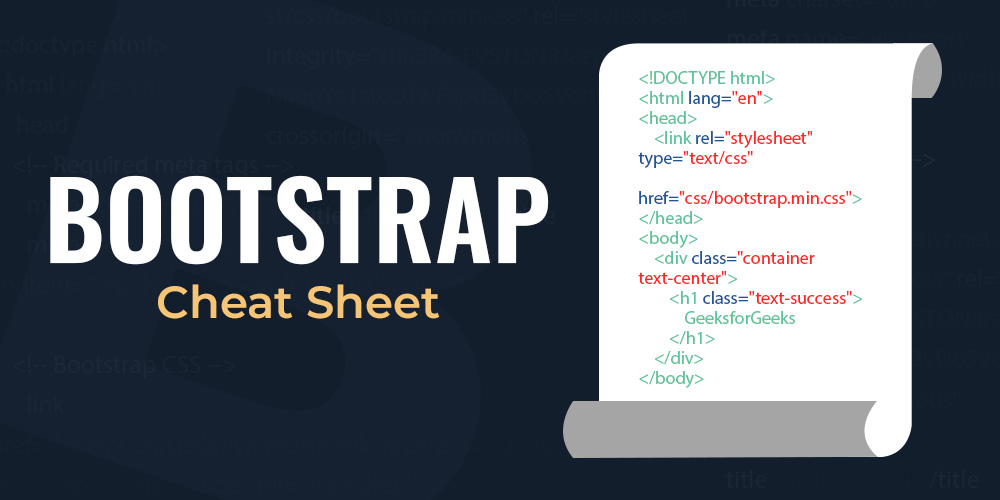
What is Bootstrap Cheat Sheet?
A Bootstrap Cheat Sheet is a comprehensive, quick-reference guide that provides web developers with an easy way to access the various classes, components, and utilities available in the Bootstrap framework. It’s a valuable tool that can significantly enhance the efficiency and productivity of web development.
Table of Content
Bootstrap provides ready-to-use form styles, including input fields, checkboxes, radio buttons, and more. Easily create user-friendly forms with minimal effort.
Element | Description | Syntax
|
---|
Form control | Default class for styling elements.
| .form-control
|
Select | Shows a collapsible list of values that can be selected.
| .form-select
|
Checks and radio | Used for radio-buttons and checkboxes
| .form-check
|
Range | Defines the range
| .form-control-range
|
Layout | Two major layout options. Fluid and fixed layout
|
|
Validation | Used to apply validation.
| :valid|invalid
|
Datalist | Style the data list using form-control classes.
|
|
Input group | Added as a wrapper around each input type or form control.
| .input-group
|
Example Code:
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<title>Bootstrap Form</title>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<link rel="stylesheet" href=
"https://maxcdn.bootstrapcdn.com/bootstrap/4.3.1/css/bootstrap.min.css">
<script src=
"https://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js">
</script>
<script src=
"https://cdnjs.cloudflare.com/ajax/libs/popper.js/1.14.7/umd/popper.min.js">
</script>
<script src=
"https://maxcdn.bootstrapcdn.com/bootstrap/4.3.1/js/bootstrap.min.js">
</script>
</head>
<body>
<h1 class="text-success text-center">
GeeksforGeeks
</h1>
<h2 class="text-center">Stacked form</h2>
<div class="container">
<form action="#">
<div class="form-group">
<label for="fname">First Name:</label>
<input type="text" class="form-control" id="fname"
placeholder="Enter First Name" name="fname">
</div>
<div class="form-group">
<label for="lname">Last Name:</label>
<input type="text" class="form-control" id="lname"
placeholder="Enter Last Name" name="lname">
</div>
<div class="form-group">
<label for="email">Email Id:</label>
<input type="email" class="form-control" id="email"
placeholder="Enter Email Id" name="email">
</div>
<div class="form-group">
<label for="contact">Contact No:</label>
<input type="text" class="form-control" id="contact"
placeholder="Enter Contact Number" name="contact">
</div>
<div class="form-group form-check">
<label class="form-check-label">
<input class="form-check-input" type="checkbox" name="remember">
Remember me
</label>
</div>
<button type="submit" class="btn bg-success">
Submit
</button>
</form>
</div>
</body>
</html>
Input Groups
Input Groups in Bootstrap are used to combine form controls with text, buttons, or other elements using input groups. Useful for search bars, currency inputs, and more.
Class | Description | Syntax |
---|
Prepend | Adds group to the front of the input.
| .input-group-prepend
|
Append | Add group behind the input.
| .input-group-append
|
Text | Styles the text that is displayed inside the group.
| .input-group-text
|
Small | Makes input groups smaller.
| .input-group-sm
|
Large | Makes input groups larger.
| .input-group-lg
|
Group | Makes size of the input group default.
| .input-group
|
Example Code:
HTML
<!DOCTYPE html>
<html>
<head>
<!-- Include Bootstrap CSS -->
<link rel="stylesheet" href=
"https://maxcdn.bootstrapcdn.com/bootstrap/4.0.0/css/bootstrap.min.css">
<title>Input Groups in Bootstrap</title>
</head>
<body>
<div class="container">
<h5>Sizing</h5>
<div class="input-group input-group-sm mb-3">
<!-- Prepend the following content to the input box -->
<div class="input-group-prepend">
<span class="input-group-text" id="small">Small</span>
</div>
<input type="text" class="form-control">
</div>
<div class="input-group mb-3">
<!-- Prepend the following content to the input box -->
<div class="input-group-prepend">
<span class="input-group-text" id="medium">Default</span>
</div>
<input type="text" class="form-control">
</div>
<div class="input-group input-group-lg">
<!-- Prepend the following content to the input box -->
<div class="input-group-prepend">
<span class="input-group-text" id="large">Large</span>
</div>
<input type="text" class="form-control">
</div>
<br>
<h5>Prepend Group Example</h5>
<div class="input-group">
<div class="input-group-prepend">
<span class="input-group-text" id="username">@</span>
</div>
<input type="text" class="form-control" placeholder="Username">
</div>
<br>
<h5>Append Group Example</h5>
<div class="input-group">
<input type="text" class="form-control" placeholder="Email">
<!-- Prepend the following content to the input box -->
<div class="input-group-append">
<span class="input-group-text" id="email">@example.com</span>
</div>
</div>
</div>
</body>
</html>
Navbars
Design responsive navigation bars that adapt to different screen sizes. Customize menus, logos, and navigation links.
Navbars | Description | Syntax
|
---|
Basic | Creates default navbar
| .navbar
|
Inverted | Inverts the color of default navbar
| .navbar-inverse
|
Colored | Changes background color
| .bg-*
|
Right-aligned | Right aligns the navbar
| .navbar-right
|
Fixed | Fixes the navigation bar at the top of the webpage.
| .navbar-fixed-top
|
Dropdown | Makes a drop-down interface.
| .dropdown
|
Collapsible | Makes a collapsible navbar
| .navbar-collapse
|
Code:
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<title>Navbar Example</title>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<link rel="stylesheet" href=
"https://maxcdn.bootstrapcdn.com/bootstrap/3.4.0/css/bootstrap.min.css">
<script src=
"https://ajax.googleapis.com/ajax/libs/jquery/3.4.1/jquery.min.js">
</script>
<script src=
"https://maxcdn.bootstrapcdn.com/bootstrap/3.4.0/js/bootstrap.min.js">
</script>
</head>
<body>
<nav class="navbar navbar-inverse navbar-fixed-top">
<div class="container-fluid">
<div class="navbar-header">
<a class="navbar-brand" href="#">WebsiteName</a>
</div>
<ul class="nav navbar-nav">
<li class="active"><a href="#">Home</a></li>
<li class="dropdown">
<a class="dropdown-toggle" data-toggle="dropdown" href="#">
Page 1<span class="caret"></span></a>
<ul class="dropdown-menu">
<li><a href="#">Page 1.1</a></li>
<li><a href="#">Page 1.2</a></li>
<li><a href="#">Page 1.3</a></li>
</ul>
</li>
<li><a href="#">Page 2</a></li>
<li><a href="#">Page 3</a></li>
</ul>
</div>
</nav>
</body>
</html>
Alerts
Alerts provide contextual feedback messages for typical user actions with a handful of available and flexible alert messages. Alerts are available for any length of text, as well as an optional close button. There are eight types of alerts available in Bootstrap 5.
Each of the classes has different colors associated with them.
Class | Description | Syntax |
---|
Primary | Alert is blue in color..
| .alert-primary
|
Secondary | Shows alert in the secondary background color.
| .alert-secondary
|
Success | Shows the alert green color.
| .alert-success
|
Danger | Alert is red in color.
| .alert-danger
|
Warning | Shows the alert in the color yellow
| .alert-warning
|
Info | Shows the alert in the color light blue.
| .alert-info
|
Light | Alert is in light gray background color.
| .alert-light
|
Dark | Shows the alert in dark gray background color.
| .alert-dark
|
Dismissible | Shows alert with a close button.
| .alert-dismissible
|
Example Code:
HTML
<!DOCTYPE html>
<html>
<head>
<title>
Bootstrap 5 | Alerts
</title>
<!-- Load Bootstrap -->
<link rel="stylesheet" href=
"https://stackpath.bootstrapcdn.com/bootstrap/5.0.0-alpha1/css/bootstrap.min.css">
</head>
<body>
<div style="text-align: center;
width:600px;">
<h1 style="color: green;">
GeeksforGeeks
</h1>
</div>
<div id="canvas" style="width: 600px;
height: 200px; margin: 20px;">
<div class="alert alert-primary" role="alert">
Primary Alert
<div class="alert alert-secondary" role="alert">
Secondary Alert
</div>
<div class="alert alert-success" role="alert">
Success Alert
</div>
<div class="alert alert-danger" role="alert">
Danger Alert
</div>
<div class="alert alert-warning" role="alert">
Warning Alert
</div>
<div class="alert alert-info" role="alert">
Info Alert
</div>
<div class="alert alert-light" role="alert">
Light Alert
</div>
<div class="alert alert-dark" role="alert">
Dark Alert
</div>
</div>
</body>
</html>
Badges
Badges are numerical indicators to highlight the number of items associated with a link or new or unread item. The class. badge within the <span> element is used to create badges.
We can have some variations in badges using the following classes:
Class | Description | Syntax
|
---|
Contextual variations | Used to change the appearance of a badge.
| .badge .bg-*
|
Pill Badges | Used to make badges more rounded
| .rounded-pill
|
Example Code:
HTML
<!DOCTYPE html>
<html>
<head>
<!-- Load Bootstrap -->
<link rel="stylesheet" href=
"https://maxcdn.bootstrapcdn.com/bootstrap/4.3.1/css/bootstrap.min.css">
<script src=
"https://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js">
</script>
<script src=
"https://cdnjs.cloudflare.com/ajax/libs/popper.js/1.14.7/umd/popper.min.js">
</script>
<script src=
"https://maxcdn.bootstrapcdn.com/bootstrap/4.3.1/js/bootstrap.min.js">
</script>
</head>
<body>
<div style="text-align: center;width: 600px;">
<h5 style="color: green;">
GeeksforGeeks
</h5>
</div>
<div id="canvas" style="width: 600px;
height: 200px;margin:20px;">
<h5>
Primary
<span class="badge bg-primary">
GeeksforGeeks
</span>
</h5>
<h5>
Secondary
<span class="badge bg-secondary">
GeeksforGeeks
</span>
</h5>
<h5>
Success
<span class="badge bg-success">
GeeksforGeeks
</span>
</h5>
<h5>
Danger
<span class="badge bg-danger">
GeeksforGeeks
</span>
</h5>
<h5>
Light
<span class="badge bg-light text-dark">
GeeksforGeeks
</span>
</h5>
<h5>
Dark
<span class="badge bg-dark">
GeeksforGeeks
</span>
</h5>
<h5>Pill Badge
<span class="badge badge-warning badge-pill">
2
</span>
</h5>
<h5>Badge inside an element
<button type="button" class="btn btn-primary">
Notifications
<span class="badge bg-secondary">
10
</span>
</button
></h5>
</div>
</body>
</html>
Breadcrumbs
Create breadcrumb trails to indicate the user’s location within a website’s hierarchy. Useful for navigation and context. It provides a backlink to each previous page the user navigates through.
Syntax:
<ol class="breadcrumb">
<li class="breadcrumb-item"> Content .. </li>
</ol>
Example Code:
HTML
<!DOCTYPE html>
<html>
<head>
<title>
Breadcrumbs Example
</title>
<link rel="stylesheet"
href=
"https://stackpath.bootstrapcdn.com/bootstrap/5.0.0-alpha1/css/bootstrap.min.css"
integrity=
"sha384-r4NyP46KrjDleawBgD5tp8Y7UzmLA05oM1iAEQ17CSuDqnUK2+k9luXQOfXJCJ4I"
crossorigin="anonymous">
</head>
<body>
<div style="text-align: center;width: 600px;">
<h1 style="color: green;">
GeeksforGeeks
</h1>
</div>
<div style="width: 600px;height: 200px;
margin:20px;">
<nav aria-label="breadcrumb">
<ol class="breadcrumb">
<li class="breadcrumb-item active">
GeeksforGeeks
</li>
</ol>
</nav>
</div>
</body>
</html>
Bootstrap offers versatile button styles, sizes, and states. Use them for calls to action, form submissions, or toggling functionality. Bootstrap also provides classes that can be used for changing the state and size of buttons, and also, provides classes for applying toggle,checkbox, and radio buttons-like effects.
Button type | Description | Syntax
|
---|
Solid | Used to create solid button styles.
| .btn-*
|
Outlined | Creates outlined buttons.
| .btn-outline-*
|
Changing Size | Changes the size of button.
| .btn-lg|sm|block
|
Toggle | Toggles the state of the button
| <button data-toggle=”button”>
|
Radio | Creates radio styled buttons
| <input type=”checkbox”>
|
Checkbox | Creates checkbox styled buttons
| <input type= “radio”>
|
Example Code:
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<!-- Bootstrap CSS -->
<link rel="stylesheet"
href=
"https://stackpath.bootstrapcdn.com/bootstrap/4.2.1/css/bootstrap.min.css"
integrity=
"sha384-GJzZqFGwb1QTTN6wy59ffF1BuGJpLSa9DkKMp0DgiMDm4iYMj70gZWKYbI706tWS"
rossorigin="anonymous">
<title>Button Example</title>
<!-- Custom CSS -->
<style>
h1 {
color: green;
text-align: center;
}
div.one {
margin-top: 40px;
text-align: center;
}
a,
button {
margin-top: 10px;
}
</style>
</head>
<body>
<div class="container">
<h1>GeeksForGeeks</h1>
<!-- Bootstrap Button Classes -->
<div class="one">
<button type="button" class="btn btn-primary">
Primary</button>
<button type="button" class="btn btn-secondary">
Secondary</button>
<button type="button" class="btn btn-success">
Success</button>
<button type="button" class="btn btn-danger">
Danger</button>
<button type="button" class="btn btn-warning">
Warning</button>
<button type="button" class="btn btn-info">
Info</button>
<button type="button" class="btn btn-light">
Light</button>
<button type="button" class="btn btn-dark">
Dark</button>
<button type="button" class="btn btn-link">
Link</button>
</div>
<div class="one">
<button type="button" class="btn btn-outline-primary">
outline</button>
<button type="button" class="btn btn-secondary btn-lg">
Large</button>
<button type="button" class="btn btn-success btn-sm">
Small</button>
<a href="#" class="btn btn-danger active" role="button" aria-pressed="true">
Active</a>
<a href="#" class="btn btn-warning disabled" role="button" aria-disabled="true" tabindex="-1">
Disabled</a>
<button type="button" class="btn btn-info" data-toggle="button"
aria-pressed="false" autocomplete="off">
Toggle
</button>
<div class="btn-group-toggle" data-toggle="buttons">
<label class="btn btn-light active">
<input type="checkbox" checked autocomplete="off">
Checked</label>
<label class="btn btn-dark">
<input type="radio" name="options" id="option1" autocomplete="off">
Radio</label>
</div>
</div>
</div>
</body>
</html>
Button groups
Bootstrap allows us to group a series of buttons together in a single line vertically or horizontally(without spaces) using the button group class. These classes are as follows:
Class | Description | Syntax
|
---|
Style | Add styles to buttons
| .btn-primary|default|success|info|link
|
Size | Changes the sizing of buttons.
| .btn-group-*
|
Nesting | Stacks the button in vertical manner
| .btn-group-vertical
|
Vertical | Creates a dropdown menu within the buttons
| .btn-group
|
Button toolbar | Combine button groups into button toolbars
| .btn-toolbar
|
Example Code:
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<title>Bootstrap Example</title>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<link rel="stylesheet" href=
"https://maxcdn.bootstrapcdn.com/bootstrap/4.5.2/css/bootstrap.min.css">
<script src=
"https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js">
</script>
<script src=
"https://cdnjs.cloudflare.com/ajax/libs/popper.js/1.16.0/umd/popper.min.js">
</script>
<script src=
"https://maxcdn.bootstrapcdn.com/bootstrap/4.5.2/js/bootstrap.min.js">
</script>
</head>
<body>
<p>Styling Button Groups</p>
<div class="btn-group">
<button type="button" class="btn btn-danger">Click</button>
<button type="button" class="btn btn-warning">Click</button>
<button type="button" class="btn btn-success">Click</button>
</div>
<br />
<p>Sizing Button Groups</p>
<div>
<div class="btn-group btn-group-lg">
<button type="button" class="btn btn-danger">Click</button>
<button type="button" class="btn btn-warning">Click</button>
<button type="button" class="btn btn-success">Click</button>
</div>
<div class="btn-group">
<button type="button" class="btn btn-danger">Click</button>
<button type="button" class="btn btn-warning">Click</button>
<button type="button" class="btn btn-success">Click</button>
</div>
<div class="btn-group btn-group-sm">
<button type="button" class="btn btn-danger">Click</button>
<button type="button" class="btn btn-warning">Click</button>
<button type="button" class="btn btn-success">Click</button>
</div>
<div class="btn-group btn-group-xs">
<button type="button" class="btn btn-danger">Click</button>
<button type="button" class="btn btn-warning">Click</button>
<button type="button" class="btn btn-success">Click</button>
</div>
</div>
<br>
<p>Vertical Button groups</p>
<div class="btn-group-vertical">
<button type="button" class="btn btn-danger">Click</button>
<button type="button" class="btn btn-warning">Click</button>
<button type="button" class="btn btn-success">Click</button>
</div>
<p>Nesting of button groups</p>
<div class="btn-group">
<button type="button" class="btn btn-danger">Click</button>
<button type="button" class="btn btn-warning">Click</button>
<div class="btn-group">
<button type="button" class="btn btn-success
dropdown-toggle" data-bs-toggle="dropdown" aria-expanded="false">
Dropdown</button>
<ul class="dropdown-menu" aria-labelledby="btnGroupDrop1">
<li><a class="dropdown-item" href="#">Item 1</a></li>
<li><a class="dropdown-item" href="#">Item 2</a></li>
</ul>
</div>
</div>
</body>
</html>
Cards
A card is a flexible and extensible content container. It includes options for headers and footers, a wide variety of content, contextual background colors, and powerful display options.
Type | Description | Syntax
|
---|
Basic | The building block of a card is the card-body and card.
| .card .card-body
|
Headers and footers | Provides header and footer to the card.
| .card-header|footer
|
Title and Links | Gives a title and link to the card.
| .card-title|link
|
Images | Used to add images to the card.
| .card-img
|
Overlays | Adds overlay to the card image
| .card-img-overlay
|
Card Group | Used to add groups to the card
| .card-deck
|
List group | Creates a list of content in card.
| .card
|
Example Code:
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<title>Bootstrap Card</title>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<link rel="stylesheet" href=
"https://maxcdn.bootstrapcdn.com/bootstrap/4.3.1/css/bootstrap.min.css">
<script src=
"https://ajax.googleapis.com/ajax/libs/jquery/3.4.1/jquery.min.js">
</script>
<script src=
"https://cdnjs.cloudflare.com/ajax/libs/popper.js/1.14.7/umd/popper.min.js">
</script>
<script src=
"https://maxcdn.bootstrapcdn.com/bootstrap/4.3.1/js/bootstrap.min.js">
</script>
</head>
<body>
<div class="container">
<h4>Basic Card</h4>
<h2 style="color:green;">GeeksforGeeks</h2>
<div class="card">
<div class="card-body">
A computer science portal for geeks
</div>
</div>
<br />
<h4>Header and Footer card</h4>
<div class="card">
<div class="card-header">Header</div>
<div class="card-body">Content</div>
<div class="card-footer">Footer</div>
</div>
<br />
<h4>Title and links card</h4>
<div class="card">
<div class="card-body">
<h4 class="card-title">Card title</h4>
<p class="card-text">
Some example text. Some example text.
</p>
<a href="#" class="card-link">Card link</a>
<a href="#" class="card-link">Another link</a>
</div>
</div>
<br />
<h4>Card with images</h4>
<div class="card" style="width:200px">
<img class="card-img-top"
src=
"https://media.geeksforgeeks.org/wp-content/cdn-uploads/20190530183756/Good-Habits-for-developers-programmers.png"
alt="Card image" style="width:100%">
<div class="card-body">
<h4 class="card-title">Developer Guy</h4>
<p class="card-text">
Developer Guy love to develop
front-end and back-end
</p>
</div>
</div>
<br />
<h4>Card group</h4>
<div class="card-deck">
<div class="card-text-white bg-primary">
<div class="card-body">
<h4 class="card-title">PRIMARY</h4>
</div>
</div>
<div class="card-text-white bg-success">
<div class="card-body">
<h4 class="card-title">SUCCESS</h4>
</div>
</div>
<div class="card-text-white bg-danger">
<div class="card-body">
<h4 class="card-title">DANGER</h4>
</div>
</div>
</div>
<br />
<h4>List Groups</h4>
<div class="card">
<ul class="list-group list-group-flush">
<li class="list-group-item">Bootstrap</li>
<li class="list-group-item">HTML</li>
<li class="list-group-item">JavaScript</li>
</ul>
</div>
</div>
</body>
</html>
Carousel
Carousal is used in bootstrap to create slideshows of images on a website. It is used to cycle through a series of images and text. Significant parts of a carousal are:
Type | Description | Syntax
|
---|
Slide | The content and image of each slide is defined here
| .carousel .slide
|
Fade | Used to add a fading effect to the transition.
| .carousel-fade
|
Indicators | The indicators are the little dots at the bottom of each slide.
| .carousel-indicators
|
Captions | Adds text associated with image to the carousal.
| .carousel-captions
|
Controls | Alows us to go back and forth.
| .carousel-control-next|previous-icon
|
Example Code:
HTML
<!DOCTYPE html>
<html>
<head>
<title>Bootstrap Carousel</title>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<link rel="stylesheet" href=
"https://maxcdn.bootstrapcdn.com/bootstrap/3.4.0/css/bootstrap.min.css">
<script src=
"https://ajax.googleapis.com/ajax/libs/jquery/3.4.1/jquery.min.js">
</script>
<script src=
"https://maxcdn.bootstrapcdn.com/bootstrap/3.4.0/js/bootstrap.min.js">
</script>
</head>
<body>
<center>
<h1 class="text-success">GeeksforGeeks</h1>
<h4>Bootstrap | Carousel</h4>
<div id="myCarousel" class="carousel slide" data-ride="carousel">
<!-- Indicators -->
<ol class="carousel-indicators">
<li data-target="#myCarousel" data-slide-to="0" class="active"></li>
<li data-target="#myCarousel" data-slide-to="1"></li>
</ol>
<!-- Wrapper for slides -->
<div class="carousel-inner">
<div class="item active">
<img src=
"https://media.geeksforgeeks.org/wp-content/cdn-uploads/20190603152813/ml_gaming.png">
</div>
<div class="item">
<img src=
"https://media.geeksforgeeks.org/wp-content/cdn-uploads/20190528184201/gateexam.png">
</div>
</div>
<!-- Left and right controls -->
<a class="left carousel-control" href="#myCarousel" data-slide="prev">
<span class="glyphicon glyphicon-chevron-left"></span>
<span class="sr-only">Previous</span>
</a>
<a class="right carousel-control" href="#myCarousel" data-slide="next">
<span class="glyphicon glyphicon-chevron-right"></span>
<span class="sr-only">Next</span>
</a>
</div>
</center>
</body>
</html>
Grids
Bootstrap’s grid system allows up to 12 columns across the page. The grid system helps align page elements based on sequenced columns and rows. We use this column-based structure to place text, images, and functions in a consistent way throughout the design.
Components
| Description | Syntax
|
---|
Container | It is a container of row elements
| .container
|
Row | Container for column elements
| .row
|
Column | Used to specify the number of available columns.
| .col
|
column reset | Used to resolve issues regarding viewport.
| .clearfix
|
column offset | Moves the columns to the right by x columns.
| .col-md|lg|cs-offset-value
|
Extra Small | Used for portrait mobile phones.
| .col-xs
|
Small | Used for Landscapes phones
| .col-sm
|
Medium | Used for Tablets/Phablets
| .col-md
|
large | Used for Small-sized Desktops/Laptops
| .col-lg
|
Extra large | Used for Larger-sized Desktops/Laptops
| .col-xl
|
Example Code:
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<link rel="stylesheet" href=
"https://maxcdn.bootstrapcdn.com/bootstrap/4.3.1/css/bootstrap.min.css">
<script src=
"https://ajax.googleapis.com/ajax/libs/jquery/3.4.0/jquery.min.js">
</script>
<script src=
"https://cdnjs.cloudflare.com/ajax/libs/popper.js/1.14.7/umd/popper.min.js">
</script>
<script src=
"https://maxcdn.bootstrapcdn.com/bootstrap/4.3.1/js/bootstrap.min.js">
</script>
</head>
<body>
<header>
<div class="container">
<h1 style="color: green">GeeksforGeeks</h1>
<strong>
A computer Science portal for Geeks
</strong>
</div>
</header>
<div class="container">
<div class="row">
<div class="bg bg-secondary w-100">
First Row
</div>
</div>
<div class="row">
<div class="bg bg-primary w-100">
Second row
</div>
</div>
</div>
<br />
<div class="container">
<div class="row">
<div class="col-xs-2 col-sm-6 col-md-3
col-lg-4 col-xl-6">
<div class="well bg bg-success">
First Column
</div>
</div>
<div class="col-xs-2 col-sm-6 col-md-3
col-lg-4 col-xl-6">
<div class="well bg bg-danger">
Second Column
</div>
</div>
<div class="col-xs-2 col-sm-6 col-md-3
col-lg-4 col-xl-6">
<div class="well bg bg-warning">
Third Column
</div>
</div>
<div class="col-xs-2 col-sm-6 col-md-3
col-lg-4 col-xl-6">
<div class="well bg bg-primary">
Fourth Column
</div>
</div>
</div>
</div>
<footer>
<hr />
<div class="container">
<p>
<a href="https://www.geeksforgeeks.org/">
Visit
</a>
our website
</p>
<p>
<a href="https://www.geeksforgeeks.org/">
Like
</a>
us on facebook
</p>
</div>
</footer>
</body>
</html>
Pagination is used to enable navigation between pages on a website. The pagination used in Bootstrap has a large block of connected links that are hard to miss and easily scalable.
Type | Description | Syntax
|
---|
Basic | Specifies pagination on a list group.
| .pagination
|
Disabled | Makes pagination appear unclickable.
| .disabled
|
Active | Highlights the currently active page
| .active
|
Sizing | Changes the sizing of the pagination group
| .pagination-sm|md|lg
|
Alignment | Sets the alignement of pagination group
| .justify-content-center|end
|
Example Code:
HTML
<!DOCTYPE html>
<html>
<head>
<title>Pagination</title>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<link rel="stylesheet" href=
"https://maxcdn.bootstrapcdn.com/bootstrap/4.3.1/css/bootstrap.min.css">
<script src=
"https://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js">
</script>
<script src=
"https://cdnjs.cloudflare.com/ajax/libs/popper.js/1.14.7/umd/popper.min.js">
</script>
<script src=
"https://maxcdn.bootstrapcdn.com/bootstrap/4.3.1/js/bootstrap.min.js">
</script>
</head>
<body>
<div class="container">
<h5>Basic Pagination</h5>
<ul class="pagination">
<!-- Declare the item in the group -->
<li class="page-item">
<!-- Declare the link of the item -->
<a class="page-link" href="#">Previous</a>
</li>
<!-- Rest of the pagination items -->
<li class="page-item">
<a class="page-link" href="#">1</a>
</li>
<li class="page-item">
<a class="page-link" href="#">2</a>
</li>
<li class="page-item">
<a class="page-link" href="#">3</a>
</li>
<li class="page-item">
<a class="page-link" href="#">Next</a>
</li>
</ul>
<br />
<h5>Disabled and active</h5>
<!-- Declare the pagination class -->
<ul class="pagination">
<!-- Specify the disabled class to style this item disabled -->
<li class="page-item disabled">
<!-- Specify tabindex to make the link non navigatable -->
<a class="page-link" href="#" tabindex="-1">
Previous
</a>
</li>
<li class="page-item">
<a class="page-link" href="#">
1
</a>
</li>
<li class="page-item">
<a class="page-link" href="#">2</a>
</li>
<li class="page-item active">
<a class="page-link" href="#">3</a>
</li>
<li class="page-item">
<a class="page-link" href="#">Next</a>
</li>
</ul>
<h5>Pagination Sizing</h5>
<b>Large Pagination</b>
<!-- Specify pagination-lg for large pagination group -->
<ul class="pagination pagination-lg">
<li class="page-item">
<a class="page-link" href="#">Previous</a>
</li>
<li class="page-item">
<a class="page-link" href="#">1</a>
</li>
<li class="page-item">
<a class="page-link" href="#">2</a>
</li>
<li class="page-item">
<a class="page-link" href="#">3</a>
</li>
<li class="page-item">
<a class="page-link" href="#">Next</a>
</li>
</ul>
<b>Small Pagination</b>
<!-- Specify pagination-sm for small pagination group -->
<ul class="pagination pagination-sm">
<li class="page-item">
<a class="page-link" href="#">Previous</a>
</li>
<li class="page-item">
<a class="page-link" href="#">1</a>
</li>
<li class="page-item">
<a class="page-link" href="#">2</a>
</li>
<li class="page-item">
<a class="page-link" href="#">3</a>
</li>
<li class="page-item">
<a class="page-link" href="#">Next</a>
</li>
</ul>
<b>Normal Pagination</b>
<!-- Normal size pagination -->
<ul class="pagination">
<li class="page-item">
<a class="page-link" href="#">Previous</a>
</li>
<li class="page-item">
<a class="page-link" href="#">1</a>
</li>
<li class="page-item">
<a class="page-link" href="#">2</a>
</li>
<li class="page-item">
<a class="page-link" href="#">3</a>
</li>
<li class="page-item">
<a class="page-link" href="#">Next</a>
</li>
</ul>
</div>
</body>
</html>
Dropdowns
Dropdowns are toggleable, contextual overlays for displaying lists of links and more. They’re made interactive with the included Bootstrap dropdown JavaScript plugin.
Class | Description | Syntax
|
---|
Divider | Creates a horizontal list to divide dropdown menu
| .dropdown
|
Header | Adds header section inside the dropdown list.
| .dropdown-header
|
Disable and active items | Used to diable and enable list of items.
| .active|disabled
|
Dropdown position | Sets the position of dropdown list.
| .dropright|dropleft
|
Dropdown Menu Right Aligned | Right aligns the dropdown menu.
| .dropdown-menu-right
|
Dropup | Expands the menu list upwards.
| .dropup
|
Dropdown text | Adds plain text in the dropdown menu list.
| .dropdown-item-text
|
Grouped Buttons with a Dropdown | Creates a group of buttons in the dropdown list.
| .btn-group
|
Split Button Dropdowns | Splits the dropdown buttons.
| .dropdown-toggle-split
|
Vertical Button Group Dropdown List | Creates a vertical button group.
| .btn-group-vertical
|
Example Code:
HTML
<!DOCTYPE html>
<html>
<head>
<!-- Load Bootstrap -->
<link rel="stylesheet"
href=
"https://stackpath.bootstrapcdn.com/bootstrap/5.0.0-alpha1/css/bootstrap.min.css"
integrity=
"sha384-r4NyP46KrjDleawBgD5tp8Y7UzmLA05oM1iAEQ17CSuDqnUK2+k9luXQOfXJCJ4I"
crossorigin="anonymous">
<script src=
"https://cdn.jsdelivr.net/npm/popper.js@1.16.0/dist/umd/popper.min.js"
integrity=
"sha384-Q6E9RHvbIyZFJoft+2mJbHaEWldlvI9IOYy5n3zV9zzTtmI3UksdQRVvoxMfooAo"
crossorigin="anonymous">
</script>
<script src=
"https://stackpath.bootstrapcdn.com/bootstrap/5.0.0-alpha1/js/bootstrap.min.js"
integrity=
"sha384-oesi62hOLfzrys4LxRF63OJCXdXDipiYWBnvTl9Y9/TRlw5xlKIEHpNyvvDShgf/"
crossorigin="anonymous">
</script>
</head>
<body style="text-align: center;">
<div class="container mt-3" style="width: 700px;">
<h1 style="color: green;">
GeeksforGeeks
</h1>
<!-- Dropdown position-->
<div class="dropdown dropright">
<button type="button" class="btn btn-success dropdown-toggle"
data-toggle="dropdown">
Select Subjects
</button>
<div class="dropdown-menu">
<!-- header and divider -->
<strong class="dropdown-header ">
CS Subjects
</strong>
<a class="dropdown-item" href="#">
Data Structure
</a>
<a class="dropdown-item" href="#">
Algorithm
</a>
<a class="dropdown-item" href="#">
Operating System
</a>
<!--Active and Disabled -->
<a class="dropdown-item active" href="#">
Computer Networks
</a>
<div class="dropdown-divider "></div>
<strong class="dropdown-header">
Other Subjects
</strong>
<a class="dropdown-item" href="#">
Physics
</a>
<a class="dropdown-item disabled" href="#">
Mathematics
</a>
<a class="dropdown-item" href="#">
Chemistry
</a>
</div>
</div>
</div>
</body>
</html>
List group
List Groups are used to display a series of content. We can modify them to support any content as per our needs. The use of List-Groups is just to display a series or list of content in an organized way.
Class | Description | Syntax
|
---|
Disabled | Makes the list group disabled.
| .disabled
|
Active | Indicates the currently active item.
| .active
|
Hyperlinks and Buttons | Creates actionable list items with multiple states.
| .list-group-item-action
|
Flush | Flush class to remove some borders and rounded corners data
| .list-group-flush
|
Contextual | Style list items with suitable backgrounds and colors.
| .list-group-item-primary|secondary|info|light
|
Badges | Adds badges to list group items.
| .badge
|
Example Code:
HTML
<!DOCTYPE html>
<html>
<head>
<title>List Groups example</title>
<!-- Link Bootstrap Files -->
<link rel="stylesheet"
href=
"https://stackpath.bootstrapcdn.com/bootstrap/4.2.1/css/bootstrap.min.css"
integrity=
"sha384-GJzZqFGwb1QTTN6wy59ffF1BuGJpLSa9DkKMp0DgiMDm4iYMj70gZWKYbI706tWS"
crossorigin="anonymous">
<script src=
"https://code.jquery.com/jquery-3.3.1.slim.min.js"
integrity=
"sha384-q8i/X+965DzO0rT7abK41JStQIAqVgRVzpbzo5smXKp4YfRvH+8abtTE1Pi6jizo"
crossorigin="anonymous">
</script>
<script src=
"https://stackpath.bootstrapcdn.com/bootstrap/4.2.1/js/bootstrap.min.js"
integrity=
"sha384-B0UglyR+jN6CkvvICOB2joaf5I4l3gm9GU6Hc1og6Ls7i6U/mkkaduKaBhlAXv9k"
crossorigin="anonymous">
</script>
</head>
<body>
<p>To do list</p>
<ul class="list-group">
<!-- active list group item -->
<li class="list-group-item active">study</li>
<!-- disabled list group item -->
<li class="list-group-item disabled">pay bills</li>
<li class="list-group-item">call mom</li>
<!-- Contextual list group item -->
<li class="list-group-item
list-group-item-info">
Info list group item
</li>
</ul>
<div>
<!-- hyperlink list group item -->
<a href="#" class="list-group-item list-group-item-action">drop an email</a>
</div>
<!-- badge list group item -->
<button type="button" class="list-group-item list-group-item-action">
UPDATES
<span class="badge badge-pill badge-danger">2</span>
</button>
</body>
</html>
Popovers
Popovers are used to display additional information about any element and are displayed with a click of a mouse pointer over that element.
Syntax:
data-toggle="popover"
title="Popover Header"
data-content="Some content inside the box"
The data-toggle attribute defines the Popover, the title attribute defines the Tile for the Popover and the data-content attribute is used to store the content to be displayed in the respective Popover.
Class | Description | Syntax
|
---|
Right-alignment | Displays the popover at right of the element.
| data-placement= “right”
|
Left-alignment | Displays the popover at left of the element.
| data-placement= “left”
|
Bottom-alignment | Displays the popover at bottom of the element.
| data-placement= “bottom”
|
Top-alignment | Displays the popover at top of the element.
| data-placement= “top”
|
Example Code:
HTML
<!DOCTYPE html>
<html>
<head>
<title>Bootstrap Example</title>
<!-- Link Bootstrap CSS -->
<link rel="stylesheet" href=
"https://maxcdn.bootstrapcdn.com/bootstrap/3.4.0/css/bootstrap.min.css">
<script src=
"https://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js">
</script>
<script src=
"https://maxcdn.bootstrapcdn.com/bootstrap/3.4.0/js/bootstrap.min.js">
</script>
</head>
<body>
<div class="container">
<h3>Popover Example</h3>
<a href="#" data-toggle="popover" title="Popover Header"
data-content="Some content inside the popover">
GeeksforGeeks</a>
</div>
<script>
$(document).ready(function () {
$('[data-toggle="popover"]').popover();
});
</script>
</body>
</html>
Spinners
The spinners are used to specify the loading state of a component or webpage. Bootstrap facilitates the various classes for creating different styles of spinners by modifying their appearance, size, and placement.
Class | Description | Syntax
|
---|
Border | Used to rotate the spinner
| .spinner-border
|
Grow | Used for growing spinners.
| .spinner-grow
|
Margin | Adds margins to the spinners.
| .m-
value |
Placement | Places spinners at different positions
| .d-flex
|
Size | Changes the size of the spinners.
| .spinner-border-sm|md|lg|xl
|
Example Code:
HTML
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport"
content="width=device-width">
<link href=
"https://cdn.jsdelivr.net/npm/bootstrap@5.1.3/dist/css/bootstrap.min.css"
rel="stylesheet">
</head>
<body>
<h2 class="text-success text-center">
GeeksforGeeks
</h2>
<h5 class="text-info text-center">
Bootstrap Spinner Border
</h4>
<div class="d-flex justify-content-center">
<div class="spinner-border text-secondary"
role="status">
</div>
<span>Please Wait </span>
</div>
<br/>
<h5 class="text-info text-center">
Bootstrap Spinner Grow
</h5>
<div class="d-flex justify-content-center">
<span>
<h5>Processing</h5>
</span>
<div class="spinner-grow text-primary"
role="status">
</div>
</div>
<br/>
<h5 class="text-info text-center">
Spinner Placement using Bootstrap classes
</h5>
<br/>
<h5 class=" text-center">
Using the Text Placement Classes
</h5>
<div class="text-center">
<div class="spinner-border m-5 " role="status">
</div>
</div>
<h5 class="text-center">
Using the Flex Box Classes
</h5>
<div class="d-flex justify-content-center">
<div class="spinner-border" role="status">
</div>
</div>
</body>
</html>
Bootstrap Scrollspy targets the navigation bar contents automatically on scrolling the area. Scrollspy is used to update the navigation links when scrolling the page. The following attributes are added with the elements for the implementation of this feature.
Attribute | Description | Syntax
|
---|
Data-spy | This element is added to the scrollable area.
| data-spy=”scroll”
|
Data-target | Connects the navigation bar with the scrollable area.
| data-target = “.id”
|
Data-offset | Specifies the number of pixels to offset from the top.
| data-offset = “value”
|
Example Code:
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<title>Bootstrap Example</title>
<meta charset="utf-8">
<meta name="viewport"
content="width=device-width, initial-scale=1">
<link rel="stylesheet" href=
"https://maxcdn.bootstrapcdn.com/bootstrap/3.4.0/css/bootstrap.min.css">
<script src=
"https://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js">
</script>
<script src=
"https://maxcdn.bootstrapcdn.com/bootstrap/3.4.0/js/bootstrap.min.js">
</script>
<style>
body {
position: relative;
}
ul.nav-pills {
top: 20px;
position: fixed;
}
div.col-sm-9 div {
height: 250px;
font-size: 18px;
}
a {
color: green;
}
#section1 {
color: white;
background-color: green;
}
#section2 {
color: green;
background-color: white;
}
#section3 {
color: white;
background-color: green;
}
#section4 {
color: green;
background-color: white;
}
@media screen and (max-width: 810px) {
#section1,
#section2,
#section3,
#section4 {
margin-left: 150px;
}
}
</style>
</head>
<body data-spy="scroll" data-target="#myScrollspy" data-offset="20">
<div class="container">
<div class="row">
<nav class="col-sm-3" id="myScrollspy">
<ul class="nav nav-pills nav-stacked">
<li class="active"><a href="#section1">
Section 1</a>
</li>
<li><a href="#section2">Section 2</a></li>
<li><a href="#section3">Section 3</a></li>
<li><a href="#section4">Section 4</a></li>
</ul>
</nav>
<div class="col-sm-9">
<div id="section1">
<center>
<h1>Section 1</h1>
<h3>GeeksforGeeks</h3>
<h5>A computer science portal for geeks.</h5>
<h6> This portal has been created to
provide well written, well thought
and well explained solutions for
selected questions related to
programming, algorithms, other
computer science subjects.
</h6>
<p>Scroll this section and see the
navigation list while scrolling.
</p>
</center>
</div>
<div id="section2">
<center>
<h1>Section 2</h1>
<h3>GeeksforGeeks</h3>
<h5>A computer science portal for geeks.</h5>
<h6> This portal also provide GATE
previous year papers, short notes
for the aspirants.
</h6>
<p>Scroll this section and see the
navigation list while scrolling.
</p>
</center>
</div>
<div id="section3">
<center>
<h1>Section 3</h1>
<h3>GeeksforGeeks</h3>
<h5>A computer science portal for geeks.</h5>
<h6>This portal also provide interview
questions asked by private and
public sectors.
</h6>
<p>Scroll this section and see the
navigation list while scrolling.
</p>
</center>
</div>
<div id="section4">
<center>
<h1>Section 4</h1>
<h3>GeeksforGeeks</h3>
<h5>A computer science portal for geeks.</h5>
<h6>It also provide Internship aor
contributor program for students.
</h6>
<p>Scroll this section and see the
navigation list while scrolling.
</p>
</center>
</div>
</div>
</div>
</div>
</body>
</html>
A tooltip is quite useful for showing the description of different elements in the webpage. The tooltip can be invoked on any element in a webpage. Tooltips on bootstrap depend on the 3rd party library Tether for positioning. We can even customize this tooltip according to our requirements. Some of them are:
Class | Description | Syntax
|
---|
Placement | Used to place a tooltip.
| data-placement = “top|bottom|right|left”
|
Html | Adds HTML to a tooltip.
| data-html = “true”
|
Offset | Sets the offset of the tool-tip relative to target.
| data-offset = “number|string”
|
Animation | Adds or removes animation to tooltip.
| data-animation = “true|false”
|
Trigger | Adds the event which will trigger tooltip.
| data-trigger = “hover focus”
|
Example Code:
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<title>Tooltip</title>
<meta charset="utf-8">
<meta name="viewport"
content="width=device-width, initial-scale=1">
<link rel="stylesheet" href=
"https://maxcdn.bootstrapcdn.com/bootstrap/4.3.1/css/bootstrap.min.css">
<script src=
"https://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script>
<script src=
"https://cdnjs.cloudflare.com/ajax/libs/popper.js/1.14.7/umd/popper.min.js"></script>
<script src=
"https://maxcdn.bootstrapcdn.com/bootstrap/4.3.1/js/bootstrap.min.js"></script>
</head>
<body style="text-align:center;">
<div class="container">
<h1 style="color:green;">
GeeksforGeeks
</h1>
<h2>Positioning Tooltip</h2>
<button data-toggle="tooltip" data-placement="top"
title="Top tooltip">Top
</button>
<button data-toggle="tooltip" data-placement="bottom"
title="Bottom tooltip">Bottom
</button>
<button data-toggle="tooltip" data-placement="left"
title="Left tooltip">Left
</button>
<button data-toggle="tooltip" data-placement="right"
title="Right tooltip">Right
</button>
</div>
<script>
$(document).ready(function () {
$('[data-toggle="tooltip"]').tooltip();
});
</script>
</body>
</html>
Jumbotron
Bootstrap Jumbotron is a responsive component whose main goal is to draw the visitor’s attention or highlight a special piece of information. Inside a Jumbotron, you can make use of almost any other Bootstrap code to further increase its engagement value.
Fluid Jumbotron: To create a full-width Jumbotron we use the jumbotron-fluid class along with the Jumbotron class.
Example Code:
HTML
<!DOCTYPE html>
<!DOCTYPE html>
<html>
<head>
<link rel="stylesheet"
href=
"https://maxcdn.bootstrapcdn.com/bootstrap/4.0.0/css/bootstrap.min.css"
integrity=
"sha384-Gn5384xqQ1aoWXA+058RXPxPg6fy4IWvTNh0E263XmFcJlSAwiGgFAW/dAiS6JXm"
crossorigin="anonymous">
</head>
<body>
<div class="jumbotron jumbotron-fluid">
<div class="container">
<h1 class="display-4">Jumbotron Example</h1>
<p class="lead">
This is a modified jumbotron that
occupies the entire horizontal
space of its parent.
</p>
</div>
</div>
</body>
</html>
Toasts
Toast is used to create something like an alert box which is shown for a short time like a couple of seconds when something happens. Like when the user clicks on a button or submits a form and many other actions.
- .toast: It helps to create a toast.
- .toast-header: It helps to create the toast header.
- .toast-body: It helps to create toast body
- .toast(“show”): It shows the toast
- .toast(“hide”): It hides the toast
- .toast(“dispose”): It disposes of the toast
Some of the events of toast are:
Event | Description |
---|
show.bs.toast | Occurs when the toast is about to be shown.
|
shown.bs.toast | Occurs when the toast is shown.
|
hide.bs.toast | Happens when the toast is about to be hidden.
|
hidden.bs.toast | Happens when the toast is fully hidden.
|
Example Code:
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<title>Toast Example</title>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<link rel="stylesheet" href=
"https://maxcdn.bootstrapcdn.com/bootstrap/4.3.1/css/bootstrap.min.css">
<script src=
"https://ajax.googleapis.com/ajax/libs/jquery/3.4.0/jquery.min.js">
</script>
<script src=
"https://cdnjs.cloudflare.com/ajax/libs/popper.js/1.14.7/umd/popper.min.js">
</script>
<script src=
"https://maxcdn.bootstrapcdn.com/bootstrap/4.3.1/js/bootstrap.min.js">
</script>
</head>
<body style="text-align: center">
<h1 style="color:green">GeeksforGeeks</h1>
<div class="container mt-3">
<h3>.toast(options)</h3>
<p>
When we click the button below there
would be delay of the toast to 8000
milliseconds.
</p>
<button type="button" class="btn btn-primary"
id="myBtn">Show Toast</button>
<div class="toast mt-3">
<div class="toast-header">
Toast Header
</div>
<div class="toast-body">
Some text inside the toast body
</div>
</div>
</div>
<script>
$(document).ready(function () {
$('#myBtn').click(function () {
$('.toast').toast({
animation: false,
delay: 2000
});
$('.toast').toast('show');
});
});
</script>
</body>
</html>
Modal
Modals are used to add dialogs to your site for lightboxes, user notifications, or completely custom content. Modals are built with HTML, CSS, and JavaScript. They’re positioned over everything else in the document and remove scroll from the <body> so that modal content scrolls instead.
Syntax:
<div class="modal"> Contents... <div>
Class | Description | Syntax
|
---|
Popovers | Added inside the modal.
| .modal
|
Content | Triggers same modal with different data
| .modal-content
|
Extra Large Modal | Provides the largest modal size with a max-width of 1140px.
| .modal-xl
|
Large Modal | Provides a large modal size with a max-width of 800px.
| .modal-lg
|
Small Modal | Provides a small modal size with a max-width of 300px.
| .modal-sm
|
Example Code:
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<!-- Required meta tags -->
<meta charset="utf-8">
<meta name="viewport"
content="width=device-width, initial-scale=1, shrink-to-fit=no">
<!-- Bootstrap CSS -->
<script src="https://code.jquery.com/jquery-3.2.1.slim.min.js"
integrity=
"sha384-KJ3o2DKtIkvYIK3UENzmM7KCkRr/rE9/Qpg6aAZGJwFDMVNA/GpGFF93hXpG5KkN"
crossorigin="anonymous"></script>
<script src=
"https://cdnjs.cloudflare.com/ajax/libs/popper.js/1.12.9/umd/popper.min.js"
integrity=
"sha384-ApNbgh9B+Y1QKtv3Rn7W3mgPxhU9K/ScQsAP7hUibX39j7fakFPskvXusvfa0b4Q"
crossorigin="anonymous"></script>
<script src="https://maxcdn.bootstrapcdn.com/bootstrap/4.0.0/js/bootstrap.min.js"
integrity=
"sha384-JZR6Spejh4U02d8jOt6vLEHfe/JQGiRRSQQxSfFWpi1MquVdAyjUar5+76PVCmYl"
crossorigin="anonymous"></script>
<link rel="stylesheet"
href=
"https://maxcdn.bootstrapcdn.com/bootstrap/4.0.0/css/bootstrap.min.css"
integrity=
"sha384-Gn5384xqQ1aoWXA+058RXPxPg6fy4IWvTNh0E263XmFcJlSAwiGgFAW/dAiS6JXm"
crossorigin="anonymous">
<title>bootstrap | Modal</title>
<style>
h1,h6 {
margin: 2%;
}
.btn {
margin-left: 2%;
}
</style>
</head>
<body>
<center>
<h1 style="color:green;">GeeksforGeeks</h1>
<!-- Button trigger modal -->
<button type="button" class="btn btn-primary" data-toggle="modal"
data-target="#exampleModal">Launch Modal</button>
<!-- Modal -->
<div class="modal fade" id="exampleModal" tabindex="-1" role="dialog"
aria-labelledby="exampleModalLabel" aria-hidden="true">
<div class="modal-dialog" role="document">
<div class="modal-content">
<div class="modal-header">
<h6 class="modal-title" id="exampleModalLabel" style="color:green;">
GeeksforGeeks
</h6>
<!-- The title of the modal -->
<button type="button" class="close" data-dismiss="modal"aria-label="Close">
<span aria-hidden="true">×</span>
</button>
</div>
<div class="modal-body">
<!-- The content inside the modal box -->
<p>The Content inside the modal </p>
</div>
<div class="modal-footer">
<button type="button" class="btn btn-secondary"
data-dismiss="modal">Close</button>
<!-- The close button in the bottom of the modal -->
<button type="button" class="btn btn-primary">okay</button>
<!-- The save changes button in the bottom of the modal -->
</div>
</div>
</div>
</div>
</body>
</html>
Borders
Borders are generally used to display an outline around a box or table cell or any other HTML element. In Bootstrap, there are different classes available to add or remove borders. The classes that are used to add borders are referred to as Additive classes and those that are used to remove borders are referred to as subtractive classes.
Class | Description | Syntax
|
---|
Border | Adds a border all around the element.
| .border
|
Border top | Adds a border on top edge of the element.
| .border-top
|
Border right | Adds a border on the right of the element.
| .border-right
|
Border bottom | Adds a border on the bottom of the element.
| .border-bottom
|
Border left | Adds a border on the left of the element.
| .border-left
|
Radius | Makes the corner of the border curved.
| .rounded
|
Color | Used to add color to the border.
| .border-*
|
Example Code:
HTML
<!DOCTYPE html>
<html>
<head>
<title>Borders Example</title>
<link rel="stylesheet" href=
"https://maxcdn.bootstrapcdn.com/bootstrap/4.1.3/css/bootstrap.min.css">
<!-- Link Bootstrap JS and JQuery -->
<script src=
"https://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script>
<script src=
"https://cdnjs.cloudflare.com/ajax/libs/popper.js/1.14.3/umd/popper.min.js"></script>
<script src=
"https://maxcdn.bootstrapcdn.com/bootstrap/4.1.3/js/bootstrap.min.js"></script>
<style>
/* CSS for Square boxes */
span {
display: inline-block;
width: 70px;
height: 70px;
margin: 6px;
background-color: #DCDCDC;
}
</style>
</head>
<body>
<div class="container">
<h4>Additive Border Classes</h4>
<span class="border border-dark"></span>
<span class="border-top border-dark"></span>
<span class="border-right border-dark"></span>
<span class="border-bottom border-dark"></span>
<span class="border-left border-dark"></span>
</div>
<div class="container">
<h4>Subtractive Border Classes</h4>
<span class="border border-dark"></span>
<span class="border border-0 border-dark"></span>
<span class="border border-top-0 border-dark"></span>
<span class="border border-right-0 border-dark"></span>
<span class="border border-bottom-0 border-dark"></span>
<span class="border border-left-0 border-dark"></span>
</div>
<div>
<h4>Color Border:</h4>
<span class="border border-primary"></span>
<span class="border border-secondary"></span>
<span class="border border-success"></span>
<span class="border border-danger"></span>
<span class="border border-warning"></span>
<span class="border border-info"></span>
<span class="border border-light"></span>
<span class="border border-dark"></span>
</div>
<div>
<h4>Border Radius</h4>
<span class="rounded"></span>
<span class="rounded-top"></span>
<span class="rounded-right"></span>
<span class="rounded-bottom"></span>
<span class="rounded-left"></span>
<span class="rounded-circle"></span>
<span class="rounded-0"></span>
</div>
</body>
</html>
Benefits of Bootstrap Cheat Sheet
- Efficient Web Development: A Bootstrap Cheat Sheet provides a quick reference guide for web developers, enabling faster and more efficient coding. It helps in reducing the time spent on searching for classes or components, thereby increasing productivity.
- Comprehensive Component Reference: The cheat sheet includes an extensive collection of Bootstrap components, classes, and utilities, covering everything from basic typography and buttons to complex components like modals and carousels. This makes it a valuable resource for both beginners and experienced developers.
- Responsive Design: With the inclusion of Bootstrap classes in the cheat sheet, developers can create more responsive and mobile-first web content. It aids in enhancing the user experience and engagement on web pages across different devices.
- Interoperability: Bootstrap is a popular front-end framework. A Bootstrap Cheat Sheet can be beneficial when working with other web technologies like HTML, CSS, JavaScript, and various web development frameworks.
- Optimized for Performance: A well-structured Bootstrap code using the correct classes and components can significantly improve the performance of web applications. The cheat sheet can guide developers in creating performance-optimized web pages.
- Consistency: Bootstrap provides a consistent framework that supports major of all browsers and CSS compatibility fixes. It’s also lightweight and customizable. The cheat sheet can guide developers in creating consistent and uniform web pages.
Remember, using a Bootstrap Cheat Sheet can greatly enhance your web development process, making it a must-have tool for every web developer.
We have Complete tutorial post with the proper roadmap of Bootstrap that includes Bootstrap 4 and Bootstrap 5. This tutorial contains each and every classes of any component with multiple possible examples.
Like Article
Suggest improvement
Share your thoughts in the comments
Please Login to comment...