Bootstrap 5 Validation
Last Updated :
16 Apr, 2024
Bootstrap 5 Validation refers to the process of enhancing form input accuracy in web development. It utilizes HTML5 validation features, providing feedback to users regarding input correctness. This feature supports customization with custom styles and JavaScript for improved user experience.
Bootstrap 5 Validation:
Term | Description |
---|
Custom styles | Add custom Bootstrap form validation messages by adding the novalidate boolean attribute to <form> an element. Disables default browser feedback tooltips. |
Browser defaults | No pre-defined class for feedback styles; cannot be styled with CSS. Feedback text can be customized via JavaScript. |
Server-side | Indicate invalid and valid form fields with .is-invalid and .is-valid . Use .invalid-feedback and .valid-feedback for feedback messages. |
Supported elements | .form-control for <input> and <textarea> , .form-select for <select> , .form-check for checkboxes and radios. |
Tooltips | Validation tooltips provide interactive textual hints about form submission requirements. |
SASS | Bootstrap 5 provides various SASS variables to validate form fields, ensuring accurate user-provided details. |
Example 1: In this example, a form is created where the “Student Name”, “Roll no” and “Mobile Number” of the student needs to be submitted and by default this form will get validated by the browser using the required attribute.
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<link href=
"https://cdn.jsdelivr.net/npm/bootstrap@5.1.3/dist/css/bootstrap.min.css"
rel="stylesheet">
</head>
<body class="m-3">
<h1 class=text-success>
GeeksforGeeks
</h1>
<form>
<div>
<label for="StudentName"
class="form-label">
Student Name
</label>
<input class="form-control"
type="text"
id="StudentName" required>
</div>
<div>
<label for="rollNo"
class="form-label">
Roll No
</label>
<input class="form-control"
type="text"
id="rollNo" required>
</div>
<div>
<label for="mobileNumber"
class="form-label">
Mobile Number
</label>
<input class="form-control"
type="number"
id="mobileNumber" required /><br>
</div>
<div>
<button class="btn btn-success"
type="submit">
Submit
</button>
</div>
</form>
</body>
</html>
Output:
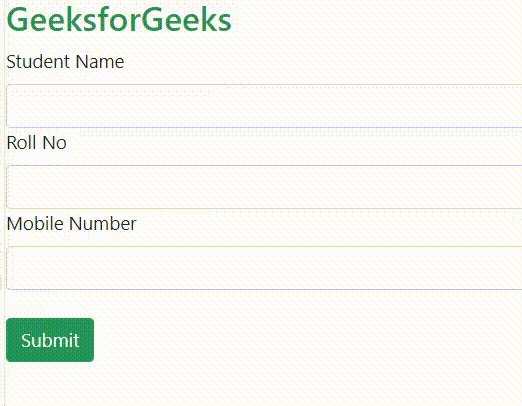
Example 2: In this example, we create Bootstrap form includes fields for name, email, password, gender, date of birth, and address. JavaScript validates password match, email format, gender selection, and address presence before submission.
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta
name="viewport"
content="width=device-width, initial-scale=1.0"
/>
<title>Bootstrap Form</title>
<link
href=
"https://cdn.jsdelivr.net/npm/bootstrap@5.3.0/dist/css/bootstrap.min.css"
rel="stylesheet"
/>
</head>
<body>
<div class="container">
<div
class="row justify-content-center mt-5"
>
<div class="col-md-6">
<form id="userForm">
<fieldset
class="border p-4 rounded mb-4 border-dark"
>
<legend
class="fs-5 mb-4"
>
User Personal
Information
</legend>
<div class="mb-3">
<label
for="name"
class="form-label"
>Enter your
full
name</label
>
<input
type="text"
class="form-control"
id="name"
name="name"
required
/>
</div>
<div class="mb-3">
<label
for="email"
class="form-label"
>Enter your
email</label
>
<input
type="email"
class="form-control"
id="email"
name="email"
required
/>
</div>
<div class="mb-3">
<label
for="password"
class="form-label"
>Enter your
password</label
>
<input
type="password"
class="form-control"
id="password"
name="password"
required
/>
</div>
<div class="mb-3">
<label
for="confirmPassword"
class="form-label"
>Confirm your
password</label
>
<input
type="password"
class="form-control"
id="confirmPassword"
name="confirmPassword"
required
/>
</div>
<div class="mb-3">
<label
class="form-label"
>Enter your
gender</label
>
<div class="row">
<div
class="col-md-4"
>
<div
class="form-check"
>
<input
class="form-check-input"
type="radio"
name="gender"
id="male"
value="male"
required
/>
<label
class="form-check-label"
for="male"
>Male</label
>
</div>
</div>
<div
class="col-md-4"
>
<div
class="form-check"
>
<input
class="form-check-input"
type="radio"
name="gender"
id="female"
value="female"
required
/>
<label
class="form-check-label"
for="female"
>Female</label
>
</div>
</div>
<div
class="col-md-4"
>
<div
class="form-check"
>
<input
class="form-check-input"
type="radio"
name="gender"
id="others"
value="others"
required
/>
<label
class="form-check-label"
for="others"
>Others</label
>
</div>
</div>
</div>
</div>
<div class="mb-3">
<label
for="dob"
class="form-label"
>Enter your
Date of
Birth</label
>
<input
type="date"
class="form-control"
id="dob"
name="dob"
required
/>
</div>
<div class="mb-3">
<label
for="address"
class="form-label"
>Enter your
Address</label
>
<textarea
class="form-control"
id="address"
name="address"
rows="3"
required
></textarea>
</div>
<button
type="submit"
class="btn btn-primary"
>
Submit
</button>
</fieldset>
</form>
</div>
</div>
</div>
<script src=
"https://cdn.jsdelivr.net/npm/bootstrap@5.3.0/dist/js/bootstrap.bundle.min.js">
</script>
<script>
document
.getElementById("userForm")
.addEventListener(
"submit",
function (event) {
const password =
document.getElementById(
"password"
).value;
const confirmPassword =
document.getElementById(
"confirmPassword"
).value;
const email =
document.getElementById(
"email"
).value;
const gender =
document.querySelector(
'input[name="gender"]:checked'
);
const address =
document.getElementById(
"address"
).value;
if (
password !==
confirmPassword
) {
alert(
"Passwords do not match"
);
event.preventDefault();
}
// Simple email validation
if (
!email.includes("@")
) {
alert(
"Invalid email"
);
event.preventDefault();
}
// Gender validation
if (!gender) {
alert(
"Please select a gender"
);
event.preventDefault();
}
// Address validation
if (
address.trim() === ""
) {
alert(
"Address field is required"
);
event.preventDefault();
}
}
);
</script>
</body>
</html>
Output:
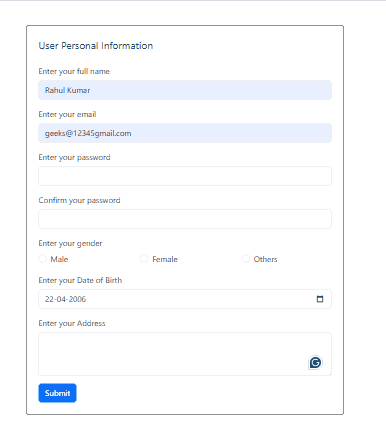
Bootstrap 5 Validation Example Output
Share your thoughts in the comments
Please Login to comment...