Python is a versatile and beginner-friendly programming language that has become immensely popular for its readability and wide range of applications. Whether you’re aiming to start a career in programming or just want to expand your skill set, learning Python is a valuable investment of your time. This article guide to help you start your journey to mastering in Python.
Best Way to Learn Python
Below, are the TOC of Best Way to Learn Python Programming.
Introduction to Python Programming Language
Python is a high-level programming language known for its simplicity, readability, and versatility. Developed in the late 1980s by Guido van Rossum, Python has since become one of the most popular languages in the world, favored by developers for its ease of learning and wide range of applications. It’s easy to learn, has clear syntax, and is used across diverse fields like web development, data science, and automation. Python’s dynamic typing and extensive standard library further enhance its appeal.
Key Features of Python
Below, are the key features of Python Programming.
- Readability: Python uses indentation and clean syntax, making code easy to understand and maintain.
- Dynamically Typed: Python automatically assigns data types to variables, simplifying code and speeding up development.
- Interpreted: Python code is executed line by line by an interpreter, allowing for quick development and testing.
- Object-Oriented: Python supports object-oriented programming paradigms, allowing for the creation of reusable and modular code.
- Extensive Standard Library: Python comes with a rich standard library, providing modules and functions for common tasks without the need for additional installations.
- Cross-Platform: Python code can run on various operating systems, including Windows, macOS, and Linux, ensuring compatibility across different platforms.
Why Choose Python?
Below are the reasons of choosing Python language.
- Easy to Learn: Python’s syntax is clear and straightforward, making it accessible to beginners.
- Versatile: Python can be used for various purposes, including web development, data analysis, artificial intelligence, scientific computing, and more.
- Huge Ecosystem: Python has a vast ecosystem of libraries and frameworks that extend its functionality for different applications.
- Community Support: Python has a large and active community of developers who contribute to its growth and provide support through forums, tutorials, and resources.
How to Install Python?
Below, are the steps to install Python.
Step 1 : Install Python
Begin by downloading and installing Python from the official website (python.org). Choose the appropriate version for your operating system and follow the installation instructions.
Step 2: Check Installation
After installation, open your command prompt (Windows) or terminal (macOS/Linux) and type
python --version
to verify that Python has been installed correctly. You should see the installed version of Python printed in the console.
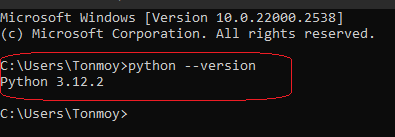
Check the version
Step 3: Install a Text Editor or IDE
Choose a text editor or integrated development environment (IDE) to write your Python code. Most used options include Visual Studio Code (Preffered) , PyCharm, Sublime Text .
Step 4: Create a Working Directory
Create a folder on your computer where you’ll store your Python projects. This will serve as your workspace for coding. Let’s write our first program ,
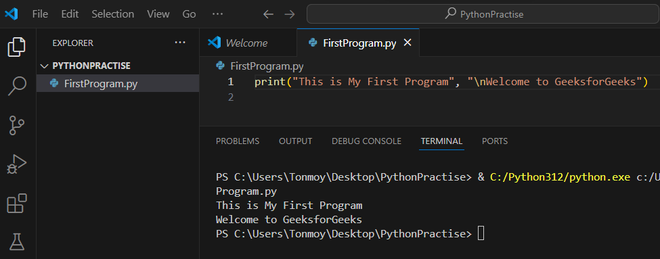
Using Visual Studio Print our first program
Step 5: Install Additional Packages (Optional)
Depending on the project requirements, you may need to install additional Python packages using the pip package manager. You can do this by running pip install package_name in your terminal.
Basics Concepts of Python
Python basics cover fundamental concepts like variables, data types, and basic operations.
1. Variables in Python
Variables are used to store data in Python. You can assign a value to a variable using the equals sign `=`.
Python
x = 5
name = "John"
print(x,name)
2. Data Types in Python
Python supports various data types, including integers, floats, strings, booleans, lists, tuples, dictionaries, and more.
Python
# Integers
age = 25
print("Age:", age)
# Floats
height = 5.11
print("Height:", height)
# Strings
message = "Hello, world!"
print("Message:", message)
# Booleans
is_student = True
print("Is Student:", is_student)
# Lists
numbers = [1, 2, 3, 4, 5]
print("Numbers:", numbers)
# Tuples
coordinates = (10, 20)
print("Coordinates:", coordinates)
# Dictionaries
person = {"name": "Alice", "age": 30}
print("Person:", person)
Output('Age:', 25)
('Height:', 5.11)
('Message:', 'Hello, world!')
('Is Student:', True)
('Numbers:', [1, 2, 3, 4, 5])
('Coordinates:', (10, 20))
('Person:', {'age': 30, 'name': 'Alice'})
3. Basic Operations in Python
Python allows you to perform arithmetic, comparison, and logical operations on data.
Python
# Arithmetic Operations
sum = 5 + 3
print("Sum:", sum)
difference = 10 - 4
print("Difference:", difference)
product = 6 * 2
print("Product:", product)
quotient = 12 / 3
print("Quotient:", quotient)
remainder = 15 % 4
print("Remainder:", remainder)
power = 2 ** 3
print("Power:", power)
# Comparison Operations
is_equal = (5 == 5)
print("Is Equal:", is_equal)
is_greater = (10 > 5)
print("Is Greater:", is_greater)
is_less_or_equal = (15 <= 20)
print("Is Less or Equal:", is_less_or_equal)
# Logical Operations
and_result = (True and False)
print("AND Result:", and_result)
or_result = (True or False)
print("OR Result:", or_result)
not_result = (not True)
print("NOT Result:", not_result)
Output('Sum:', 8)
('Difference:', 6)
('Product:', 12)
('Quotient:', 4)
('Remainder:', 3)
('Power:', 8)
('Is Equal:', True)
('Is Greater:', True)
('Is Less or Equal:', True)
('AND Result:', False)
('OR Resul...
Python Data Structures
Python data structures are ways to organize and store data in your code. They help you manage and work with different types of information more efficiently. Here are some common Python data structures –
1. Lists in Python
Lists are ordered collections of items that can contain elements of different data types. They are mutable, meaning you can modify the elements after the list is created.
Syntax
my_list = [element1, element2, element3, …]
Example:
Python
# Creating a list
my_list = [1, 2, 3, 'apple', 'banana']
# Accessing elements in a list
print("First element:", my_list[0])
# Modifying elements in a list
my_list[2] = 10
print("Modified list:", my_list)
# Adding elements to a list
my_list.append('orange')
print("List with added element:", my_list)
# Removing elements from a list
my_list.remove(2)
print("List with removed element:", my_list)
Output('First element:', 1)
('Modified list:', [1, 2, 10, 'apple', 'banana'])
('List with added element:', [1, 2, 10, 'apple', 'banana', 'orange'])
('List with removed element:', [1, 10, 'apple', 'banana', ...
2.Tuples in Python
Tuples are ordered collections of items similar to lists, but they are immutable, meaning once created, their contents cannot be changed.
Synatx
my_tuple = (element1, element2, element3, …)
Example :
Python
# Creating a tuple
my_tuple = (1, 2, 3, 'apple', 'banana')
# Accessing elements in a tuple
print("Third element:", my_tuple[2])
Output('Third element:', 3)
3.Dictionaries in Python
Dictionaries are unordered collections of key-value pairs. Each key is unique and maps to a corresponding value, similar to a real-world dictionary.
Synatx
my_dict = {“key1”: value1, “key2”: value2, “key3”: value3, …}
Example:-
Python
# Creating a dictionary
my_dict = {"name": "John", "age": 30, "city": "New York"}
# Accessing values in a dictionary
print("Name:", my_dict["name"])
# Modifying values in a dictionary
my_dict["age"] = 35
print("Modified dictionary:", my_dict)
Output('Name:', 'John')
('Modified dictionary:', {'city': 'New York', 'age': 35, 'name': 'John'})
4. Sets in Python
Sets are unordered collections of unique elements. They are useful for tasks like removing duplicates from a list or checking for membership.
Syntax
my_set = {element1, element2, element3, …}
Example:-
Python
# Creating a set
my_set = {1, 2, 3, 4, 5}
# Adding elements to a set
my_set.add(6)
print("Set with added element:", my_set)
Output('Set with added element:', set([1, 2, 3, 4, 5, 6]))
Control Flow in Python
Control flow in Python refers to the order in which statements are executed in a program. It allows users to make decisions, repeat actions, and execute code based on conditions.
1. Conditional Statements (if, elif, else) in Python
Conditional statements allow you to execute different blocks of code based on whether a condition is true or false.
Syntax:-
if condition:
# Code block executed if condition1 is True
elif condition2:
# Code block executed if condition1 is False and condition2 is True
else:
# Code block executed if both condition1 and condition2 are False
Example:-
Python
x = 10
if x > 5:
print("x is greater than 5")
elif x == 5:
print("x is equal to 5")
else:
print("x is less than 5")
Outputx is greater than 5
2.Loops (for, while) in Python
Loops allows to repeat a block of code multiple times.
Executes a block of code for each item in a sequence.
Syntax:-
for item in sequence:
# Code block executed for each item in the sequence
Example:-
Python
numbers = [1, 2, 3, 4, 5]
for num in numbers:
print(num)
Executes a block of code repeatedly as long as a condition is true.
Syntax:-
while condition:
# Code block executed as long as the condition is True
Example:-
Python
x = 0
while x < 5:
print(x)
x += 1
3.Break and Continue Statements in Python
- break: Terminates the loop prematurely when a condition is met.
- continue: Skips the rest of the loop and continues with the next iteration.
Example:-
Python
numbers = [1, 2, 3, 4, 5]
for num in numbers:
if num == 3:
break # Exit the loop when num is 3
print(num)
4.Exception Handling (try, except) in Python
Exception handling allows users to handle errors gracefully without crashing the program.
Syntax:-
try:
# Code that may raise an exception
except ExceptionType:
# Code executed if an exception of type ExceptionType occurs
else:
# Code executed if no exception occurs
finally:
# Code that always executes, regardless of whether an exception occurs
Example:-
Python
try:
result = 10 / 0
except ZeroDivisionError:
print("Division by zero is not allowed")
else:
print("Division successful")
finally:
print("Execution completed")
OutputDivision by zero is not allowed
Execution completed
Python Libraries and Frameworks
Below, are the explanation of Python libraries and frameworks.
Python Libraries
- Libraries are collections of functions and modules that you can import into your Python code to perform specific tasks.
- They offer ready-made solutions for common programming tasks, such as mathematical operations, working with data, or interacting with external services.
- Examples of Python libraries include math for mathematical operations, requests for making HTTP requests, and random for generating random numbers.
Example:-
Suppose we want to import the random library and use it to generate a random number. The random library provides functions for generating random numbers and selecting random elements from sequences.
Python
import random
# Generate a random integer between 1 and 10
random_number = random.randint(1, 10)
# Print the random number
print("Random number:", random_number)
Output('Random number:', 7)
Python Frameworks
- Frameworks are pre-built structures that provide a foundation for developing applications.
- They offer a set of tools, rules, and conventions to streamline the development process and ensure consistency across projects.
- Frameworks often include features like routing, templating, and database integration, making it easier to build complex applications.
- Examples of Python frameworks include Flask and Django for web development, TensorFlow and PyTorch for machine learning, and pandas for data analysis.
Best Practices in Python Programming
- Use Descriptive Variable Name- Choose variable names that describe their purpose clearly. This makes your code more readable and understandable.
- Comment Your Code- Add comments to explain complex logic or to provide context for your code. This helps others understand your code and facilitates collaboration.
- Avoid Magic Numbers- Instead of using literal numbers directly in your code, assign them to variables with descriptive names. This makes your code more maintainable and easier to modify.
- Use List Comprehensions- Employ list comprehensions for concise and readable code when creating lists from iterables or applying operations to elements.
- Document Your Code- Write docstrings for functions and modules to provide documentation on their purpose, parameters, and return values. This helps other developers understand how to use your code.
- Test Your Code- Write unit tests to verify that individual components of your code work as expected. This ensures the reliability and correctness of your code.
Conclusion
In conclusion, Python is a great programming language for beginners and experts alike. It’s easy to learn and can be used for many different things. Whether you’re just starting out or want to improve your skills, learning Python is definitely a good idea. This article is a helpful guide to getting started with Python, covering all the basics and giving you tips for writing better code. Python has a simple syntax, lots of built-in functions, and a helpful community, making it a great choice for anyone who wants to learn programming.
Share your thoughts in the comments
Please Login to comment...