Angular 10 formatNumber() Method
Last Updated :
02 Jun, 2021
In this article, we are going to see what is formatNumber in Angular 10 and how to use it.
formatNumber is used to format a number based on our requirement in decimal form.
Syntax:
formatNumber(value, locale, digitsInfo)
Parameters:
- value: The number to format.
- locale: A locale code for the locale format.
- digitsInfo: Decimal representation options.
Return Value:
- string: the formatted text string.
NgModule: Module used by formatNumber is:
Approach:
- In app.component.ts import formatNumber and LOCALE_ID
- inject LOCALE_ID as a public variable.
- In app.component.html show the local variable using string interpolation
- Serve the angular app using ng serve to see the output.
Example 1:
app.component.ts
import {
formatNumber
}
from '@angular/common' ;
import {Component,
Inject,
LOCALE_ID }
from '@angular/core' ;
@Component({
selector: 'app-root' ,
templateUrl: './app.component.html'
})
export class AppComponent {
curr = formatNumber(1000, this .locale,
'7.1-5' );
constructor(
@Inject(LOCALE_ID) public locale: string,){}
}
|
app.component.html
< h1 >
GeeksforGeeks
</ h1 >
< p >Locale Number is : {{curr}}</ p >
|
Output:
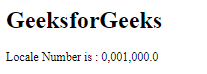
Example 2:
app.component.ts
import {
formatNumber
}
from '@angular/common' ;
import {Component,
Inject,
LOCALE_ID }
from '@angular/core' ;
@Component({
selector: 'app-root' ,
templateUrl: './app.component.html'
})
export class AppComponent {
curr = formatNumber(100, this .locale,
'2.1-5' );
constructor(
@Inject(LOCALE_ID) public locale: string,){}
}
|
app.component.html
< h1 >
GeeksforGeeks
</ h1 >
< p >Locale Number is : {{curr}}</ p >
|
Output:
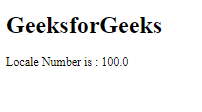
Example 3:
app.component.ts
import {
formatNumber
}
from '@angular/common' ;
import {Component,
Inject,
LOCALE_ID }
from '@angular/core' ;
@Component({
selector: 'app-root' ,
templateUrl: './app.component.html'
})
export class AppComponent {
curr = formatNumber(3234, this .locale,
'3.1-4' );
constructor(
@Inject(LOCALE_ID) public locale: string,){}
}
|
app.component.html
< h1 >
GeeksforGeeks
</ h1 >
< p >Locale Number is : {{curr}}</ p >
|
Output:
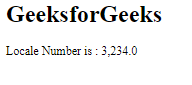
Reference: https://angular.io/api/common/formatNumber
Share your thoughts in the comments
Please Login to comment...