Angular orderBy Pipe
Last Updated :
06 Dec, 2023
Angular Pipes are a way to transform the format of output data for display. The data can be strings, currency amounts, dates, etc. In this article, we will learn How to use orderBy in Angular pipe. The orderBy is used with a dependency called lodash. We will implement a pipe to achieve the desired result.
Steps for Installing & Configuring the Angular Application
Step 1: Create an Angular application using the following command.
ng new appname
Step 2: After creating your project folder i.e. appname, move to it using the following command.
cd appname
Step 3: Create a pipe, go to src/app
ng generate pipe geek
Step 4: Install Dependency
npm i --save-dev @types/lodash
Project Structure
It will look like the following:
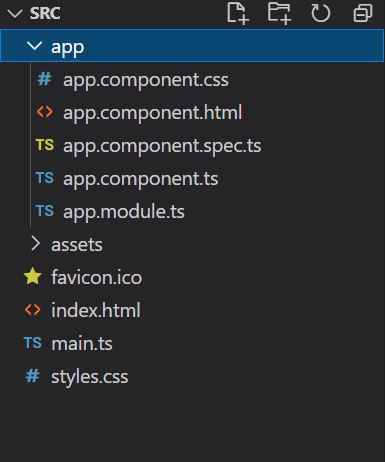
Example 1: In this example, we will learn how to use orderBy to display the values in ascending order.
HTML
< h2 style = "color: green" >GeeksforGeeks</ h2 >
< h2 >Angular orderBy pipe</ h2 >
< div * ngFor = "let item of gfg | orderBy: 'cost'" >
{{item.course}} : {{ item.cost}}
</ div >
|
Javascript
import { Component, OnInit } from '@angular/core' ;
import { KeyValue } from '@angular/common' ;
import { Pipe, PipeTransform } from '@angular/core' ;
@Component({
selector: 'app-root' ,
templateUrl: "./app.component.html" ,
styleUrls: [ './app.component.css' ]
})
export class AppComponent {
gfg = [
{ course: "HTML " , cost: "2100" },
{ course: "CSS" , cost: "1800" },
{ course: "XML" , cost: "1900" },
{ course: "Angular " , cost: "1700" },
{ course: "React " , cost: "1600" }
]
}
|
Javascript
import { NgModule }
from '@angular/core' ;
import { BrowserModule }
from '@angular/platform-browser' ;
import { HttpClientModule }
from '@angular/common/http' ;
import { AppComponent }
from './app.component' ;
import { GeekPipe }
from './geek.pipe' ;
@NgModule({
declarations: [
AppComponent,
GeekPipe
],
imports: [
BrowserModule,
HttpClientModule,
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
|
Javascript
import { Pipe, PipeTransform } from "@angular/core" ;
import { orderBy } from 'lodash' ;
@Pipe({
name: "orderBy"
})
export class GeekPipe implements PipeTransform {
transform(array: any, arg2: any): any[] {
return orderBy(array, "cost" , 'asc' );
}
}
|
Output:
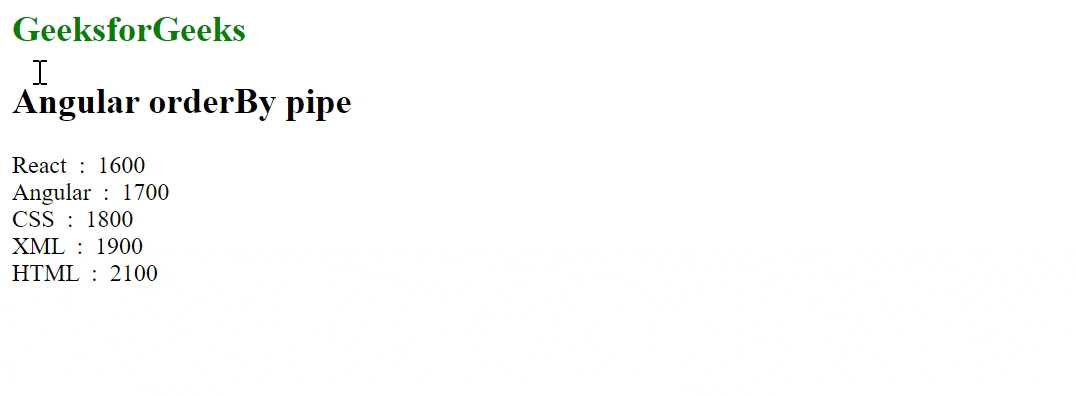
Example 2: In this example, we will learn how to use orderBy to display the values in descending order.
HTML
< h2 style = "color: green" >GeeksforGeeks</ h2 >
< h2 >Angular orderBy pipe</ h2 >
< div * ngFor = "let item of gfg | orderBy: 'cost'" >
{{item.course}} : {{ item.cost}}
</ div >
|
Javascript
import { Component, OnInit } from '@angular/core' ;
import { KeyValue } from '@angular/common' ;
import { Pipe, PipeTransform } from '@angular/core' ;
@Component({
selector: 'app-root' ,
templateUrl: "./app.component.html" ,
styleUrls: [ './app.component.css' ]
})
export class AppComponent {
gfg = [
{ course: "HTML " , cost: "2100" },
{ course: "CSS" , cost: "1800" },
{ course: "XML" , cost: "1900" },
{ course: "Angular " , cost: "1700" },
{ course: "React " , cost: "1600" }
]
}
|
Javascript
import { NgModule }
from '@angular/core' ;
import { BrowserModule }
from '@angular/platform-browser' ;
import { HttpClientModule }
from '@angular/common/http' ;
import { AppComponent }
from './app.component' ;
import { GeekPipe }
from './geek.pipe' ;
@NgModule({
declarations: [
AppComponent,
GeekPipe
],
imports: [
BrowserModule,
HttpClientModule,
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
|
Javascript
import { Pipe, PipeTransform } from "@angular/core" ;
import { orderBy } from 'lodash' ;
@Pipe({
name: "orderBy"
})
export class GeekPipe implements PipeTransform {
transform(array: any, arg2: any): any[] {
return orderBy(array, "cost" , 'desc' );
}
}
|
Output:
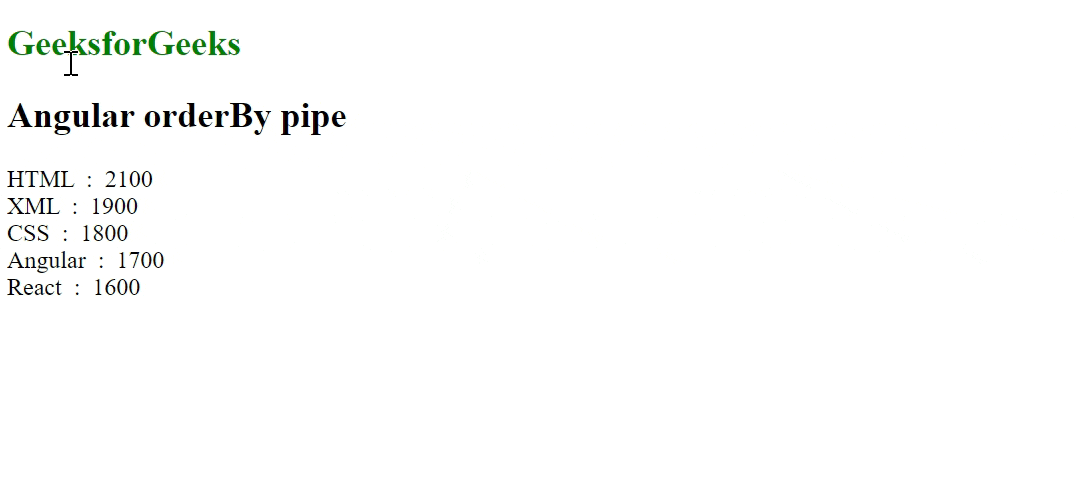
Share your thoughts in the comments
Please Login to comment...