Aggregation in MongoDB using Python
Last Updated :
03 Oct, 2022
MongoDB is free, open-source,cross-platform and document-oriented database management system(dbms). It is a NoSQL type of database. It store the data in BSON format on hard disk. BSON is binary form for representing simple data structure, associative array and various data types in MongoDB. NoSQL is most recently used database which provide mechanism for storage and retrieval of data. Instead of using tables and rows as in relational databases, mongodb architecture is made up of collections and documents.
Aggregation in MongoDB
Aggregation operation groups the values from multiple documents(rows in case of SQL) together to perform a variety of operations on the grouped data and is going to return a single result for each grouped data after aggregation.
Syntax:
db.collection_name.aggregate(aggregate operations)
Sample Database used in all the below examples:
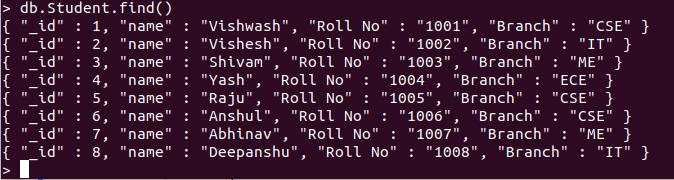
Example 1:
Python3
from pymongo import MongoClient
my_client = MongoClient( 'localhost' , 27017 )
db = my_client[ "GFG" ]
coll = db[ "Student" ]
cursor = coll.aggregate([{ "$group" :
{ "_id" : "$Branch" ,
"similar_branches" :{ "$sum" : 1 }
}
}])
for document in cursor:
print (document)
|
Output:
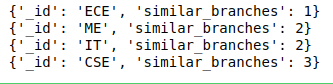
Here, we use “$group” command for grouping then by “_id”:”branches” we are grouping ids according to the branches. “similar_branches” is the keyword used for the total number of similar branches,we can use any keyword here. “$sum:1” is used as a counter of total number of each branches. The sum is incrementing by 1.
Example 2: We can also use the aggregation query for counting the number of document in the database.
Python3
from pymongo import MongoClient
my_client = MongoClient( 'localhost' , 27017 )
db = my_client[ "GFG" ]
coll = db[ "Student" ]
cursor = coll.aggregate([{ "$group" :
{ "_id" : "$None" ,
"total collections" :{ "$sum" : 1 }
}
}])
for document in cursor:
print (document)
|
Output:
{'_id': None, 'total collections': 8}
Share your thoughts in the comments
Please Login to comment...