Python Mongodb – Delete_many()
Last Updated :
11 Jul, 2022
MongoDB is a general-purpose, document-based, distributed database built for modern application developers and the cloud. It is a document database, which means it stores data in JSON-like documents. This is an efficient way to think about data and is more expressive and powerful than the traditional table model.
Delete_many()
Delete_many() is used when one needs to delete more than one document. A query object containing which document to be deleted is created and is passed as the first parameter to the delete_many().
Syntax:
collection.delete_many(filter, collation=None, hint=None, session=None)
Parameters:
- ‘filter’ : A query that matches the document to delete.
- ‘collation’ (optional) : An instance of class: ‘~pymongo.collation.Collation’. This option is only supported on MongoDB 3.4 and above.
- ‘hint’ (optional) : An index to use to support the query predicate. This option is only supported on MongoDB 3.11 and above.
- ‘session’ (optional) : a class:’~pymongo.client_session.ClientSession’.
Sample Database:
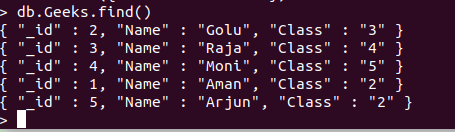
Example 1: Deleting all the documents where the name starts with ‘A’.
Python3
import pymongo
client = pymongo.MongoClient("mongodb: / / localhost: 27017 / ")
mydb = client["GFG"]
col = mydb["Geeks"]
query = {"Name": {"$regex": "^A"}}
d = col.delete_many(query)
print (d.deleted_count, " documents deleted !!")
|
Output:
2 documents deleted !!
MongoDB Shell:
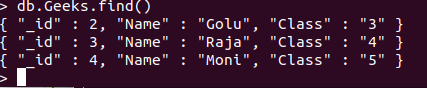
Example 2:
Python3
import pymongo
client = pymongo.MongoClient("mongodb: / / localhost: 27017 / ")
mydb = client["GFG"]
col = mydb["Geeks"]
query = {"Class": '3' }
d = col.delete_many(query)
print (d.deleted_count, " documents deleted !!")
|
Output:
1 documents deleted !!
MongoDB Shell:
Share your thoughts in the comments
Please Login to comment...